JavaScript拼图最优解的示例代码
时间: 2023-09-10 10:05:43 浏览: 95
### 回答1:
JavaScript 拼图最优解的示例代码可以使用 A* 算法来实现。它有一个函数,可以用来查找最短路径,从而求出最优解。示例代码如下:function astar(start, goal) {
let openSet = [start],
closedSet = [],
cameFrom = [],
gScore = [],
fScore = [];
gScore[start] = 0;
fScore[start] = heuristicCostEstimate(start, goal); while (openSet.length > 0) {
let current = getLowestScoreIndex(openSet, fScore); if (current == goal) {
return reconstructPath(cameFrom, current);
} openSet.splice(openSet.indexOf(current), 1);
closedSet.push(current); for (let i = 0; i < neighbors.length; i++) {
let neighbor = neighbors[i]; if (closedSet.includes(neighbor)) {
continue;
} let tentativeGScore = gScore[current] + distBetween(current, neighbor);
if (!openSet.includes(neighbor)) {
openSet.push(neighbor);
}
else if (tentativeGScore >= gScore[neighbor]) {
continue;
} cameFrom[neighbor] = current;
gScore[neighbor] = tentativeGScore;
fScore[neighbor] = gScore[neighbor] + heuristicCostEstimate(neighbor, goal);
}
}
}
### 回答2:
JavaScript拼图的最优解是使用深度优先搜索算法(DFS)来实现。以下是一个示例代码:
```javascript
// 定义拼图的初始状态
var puzzle = [[1, 2, 3], [4, 6, 0], [7, 5, 8]];
var target = [[1, 2, 3], [4, 5, 6], [7, 8, 0]];
// 定义移动方向数组
var directions = [[-1, 0], [1, 0], [0, -1], [0, 1]]; // 上下左右
// 定义拼图大小
var puzzleSize = 3;
// 定义存储搜索路径的栈
var stack = [];
// 定义已访问状态的集合,防止重复搜索
var visited = new Set();
// 深度优先搜索函数
function dfs() {
stack.push(puzzle); // 将初始状态放入栈中
visited.add(puzzle.toString()); // 将初始状态添加到已访问集合中
while (stack.length > 0) {
var cur = stack.pop(); // 取出当前状态
if (cur.toString() === target.toString()) {
console.log("找到最优解!");
return;
}
// 获取空格位置
var x, y;
for (var i = 0; i < puzzleSize; i++) {
for (var j = 0; j < puzzleSize; j++) {
if (cur[i][j] === 0) {
x = i;
y = j;
break;
}
}
}
// 移动到相邻状态
for (var k = 0; k < directions.length; k++) {
var newX = x + directions[k][0];
var newY = y + directions[k][1];
if (newX >= 0 && newX < puzzleSize && newY >= 0 && newY < puzzleSize) {
var newPuzzle = JSON.parse(JSON.stringify(cur)); // 深拷贝当前状态
newPuzzle[x][y] = newPuzzle[newX][newY];
newPuzzle[newX][newY] = 0;
if (!visited.has(newPuzzle.toString())) {
stack.push(newPuzzle); // 将新状态加入栈中
visited.add(newPuzzle.toString()); // 将新状态添加到已访问集合中
}
}
}
}
console.log("无解!");
}
dfs(); // 执行深度优先搜索
```
这段代码通过深度优先搜索算法来寻找拼图的最优解。它首先定义了拼图的初始状态和目标状态,然后以初始状态为起点开始搜索。在搜索过程中,使用一个栈来保存搜索路径,使用一个集合来存储已访问状态,以防止重复搜索。在每一步搜索中,首先判断当前状态是否达到目标状态,如果是,则找到了最优解,算法结束;否则,找到空格的位置,然后尝试将空格与相邻的位置交换,生成新的状态,并将新的状态加入栈中。最后,如果栈为空,算法结束并输出"无解"。
### 回答3:
JavaScript拼图游戏的最优解是使用A*搜索算法来实现。下面是一个示例代码:
```javascript
// 定义拼图状态类
class PuzzleState {
constructor(board, moves, heuristic) {
this.board = board; // 当前拼图状态的棋盘
this.moves = moves; // 已经移动的步数
this.heuristic = heuristic; // 启发式函数的值
}
}
// 定义启发式函数(曼哈顿距离)
function manhattanDistance(board) {
let distance = 0;
for (let i = 0; i < board.length; i++) {
for (let j = 0; j < board[i].length; j++) {
let value = board[i][j];
if (value !== 0) {
let targetX = Math.floor((value - 1) / board.length);
let targetY = (value - 1) % board.length;
distance += Math.abs(i - targetX) + Math.abs(j - targetY);
}
}
}
return distance;
}
// 定义获取邻居状态的函数
function getNeighbors(state) {
let neighbors = [];
let zeroX, zeroY;
for (let i = 0; i < state.board.length; i++) {
for (let j = 0; j < state.board[i].length; j++) {
if (state.board[i][j] === 0) {
zeroX = i;
zeroY = j;
break;
}
}
}
// 交换0与上、下、左、右邻居的位置
const directions = [[-1, 0], [1, 0], [0, -1], [0, 1]];
for (const direction of directions) {
let newX = zeroX + direction[0];
let newY = zeroY + direction[1];
if (newX >= 0 && newX < state.board.length && newY >= 0 && newY < state.board.length) {
let newBoard = JSON.parse(JSON.stringify(state.board));
[newBoard[zeroX][zeroY], newBoard[newX][newY]] = [newBoard[newX][newY], newBoard[zeroX][zeroY]]; // 交换位置
let newMoves = state.moves + 1;
let newHeuristic = manhattanDistance(newBoard);
neighbors.push(new PuzzleState(newBoard, newMoves, newHeuristic));
}
}
return neighbors;
}
// 定义A*算法函数
function solvePuzzle(initialState) {
let openList = [initialState]; // 存放待搜索的状态
let closedList = []; // 存放已搜索的状态
while (openList.length > 0) {
// 从openList中找到启发式值最小的状态
let current = openList[0];
let currentIndex = 0;
for (let i = 1; i < openList.length; i++) {
if (openList[i].heuristic < current.heuristic) {
current = openList[i];
currentIndex = i;
}
}
// 判断是否已经达到目标状态
if (current.heuristic === 0) {
return current.moves;
}
// 将当前状态从openList中移除,并加入closedList
openList.splice(currentIndex, 1);
closedList.push(current);
// 获取邻居状态
let neighbors = getNeighbors(current);
for (const neighbor of neighbors) {
// 判断该邻居状态是否已经在closedList中
let isVisited = false;
for (const state of closedList) {
if (JSON.stringify(state.board) === JSON.stringify(neighbor.board)) {
isVisited = true;
break;
}
}
if (isVisited) {
continue;
}
// 计算邻居状态的g值
let gValue = current.moves + 1;
// 判断该邻居状态是否已经在openList中
let isOpen = false;
for (const state of openList) {
if (JSON.stringify(state.board) === JSON.stringify(neighbor.board)) {
isOpen = true;
if (gValue < state.moves) {
state.moves = gValue;
}
break;
}
}
// 如果邻居状态不在openList中,则加入openList
if (!isOpen) {
neighbor.moves = gValue;
openList.push(neighbor);
}
}
}
// 如果没有找到解,则返回-1
return -1;
}
// 定义初始状态的棋盘
let initialBoard = [
[1, 2, 3],
[4, 0, 6],
[7, 5, 8]
];
// 创建初始状态对象
let initialState = new PuzzleState(initialBoard, 0, manhattanDistance(initialBoard));
// 解决拼图问题
let moves = solvePuzzle(initialState);
console.log("最短移动步数:" + moves);
```
这段代码实现了一个带有A*搜索算法的拼图求解器。使用了PuzzleState类来表示拼图状态,manhattanDistance函数作为启发式函数计算曼哈顿距离,getNeighbors函数获取邻居状态,solvePuzzle函数实现拼图求解的A*算法。通过调用solvePuzzle函数并传入初始状态,即可得到最优解的移动步数。
阅读全文
相关推荐
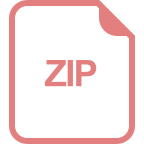
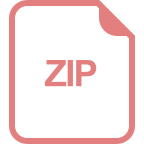
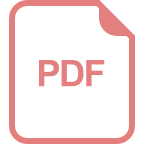
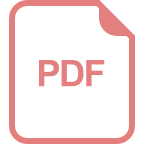
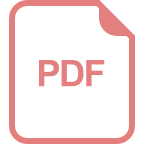
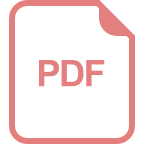
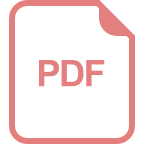
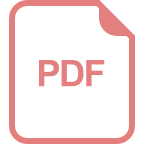
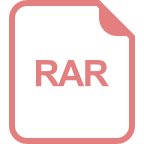
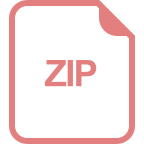
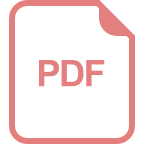
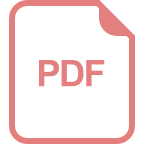
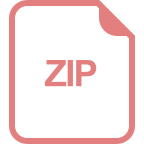
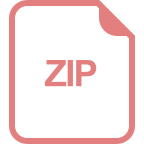
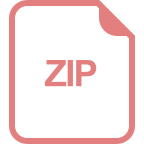