request nodejs
时间: 2023-10-04 07:07:27 浏览: 98
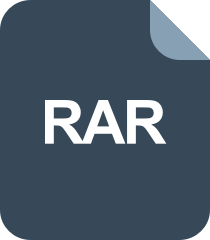
关于java请求nodejs
您是想问如何在 Node.js 中发送 HTTP 请求吗?
如果是的话,您可以使用内置的 `http` 模块或者第三方的 `axios` 模块进行请求。以下是两种方法的示例:
使用内置的 `http` 模块:
```javascript
const http = require('http');
const options = {
hostname: 'example.com',
port: 80,
path: '/api/endpoint',
method: 'POST',
headers: {
'Content-Type': 'application/json'
}
};
const data = JSON.stringify({
key1: 'value1',
key2: 'value2'
});
const req = http.request(options, (res) => {
let response = '';
res.on('data', (chunk) => {
response += chunk;
});
res.on('end', () => {
console.log(response);
});
});
req.on('error', (error) => {
console.error(error);
});
req.write(data);
req.end();
```
使用第三方的 `axios` 模块:
```javascript
const axios = require('axios');
const url = 'http://example.com/api/endpoint';
const data = {
key1: 'value1',
key2: 'value2'
};
axios.post(url, data)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
```
请注意,您需要将 `url` 替换为您要发送 POST 请求的目标 URL,将 `data` 替换为您要发送的数据。根据 API 的要求,您可能还需要设置其他请求头或以不同的方式发送数据。
阅读全文
相关推荐
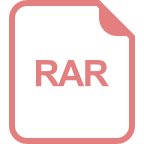














