定义一个函数asc(),函数的原型为:void asc(int a[],int n);该函数的功能为:给n个长度的整数数组进行升序排列。
时间: 2023-03-25 10:03:02 浏览: 116
asc函数的实现如下:
void asc(int a[], int n) {
int i, j, temp;
for (i = ; i < n - 1; i++) {
for (j = i + 1; j < n; j++) {
if (a[i] > a[j]) {
temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
}
}
该函数使用了冒泡排序算法,通过比较相邻的元素,将较大的元素交换到后面,从而实现升序排列。
相关问题
1.从键盘输入一个整数,判定该数是否为完数,不是完数则显示“某数不是完数”,如: 8不是完数;是的话则显示“某数是完数,它的因数为: 如: 6是完数,它的因数为1 2 3 3.定义一个函数asc (),函数的原型为: void asc(int a[],int n);该函数的功能为:给n个长度的整数数组进行升序排列。在主函数中定义一个包含10个元素的整数数组b,通过键盘输入10个整数,调用asc()函数,将b数组中的数据进行升序排21列,并将排序后的数据输出。
某数是完数,它的因数为: 6是完数,它的因数为1 2 3
void asc(int a[],int n) {
int i,j,temp;
for(i=;i<n-1;i++) {
for(j=;j<n-i-1;j++) {
if(a[j]>a[j+1]) {
temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
}
}
}
}
//主函数
int main() {
int b[10],i;
for(i=;i<10;i++) {
scanf("%d",&b[i]);
}
asc(b,10);
for(i=;i<10;i++) {
printf("%d ",b[i]);
}
return ;
}
在c语言中将字符串用asc值排序
可以使用字符串排序函数 `qsort`,结合自定义的比较函数,来实现按照 ASCII 值排序字符串的功能。
比较函数的原型为:
```c
int cmp(const void *a, const void *b);
```
其中,`a` 和 `b` 分别指向待比较的两个字符串,函数返回值根据比较结果决定:
- 若 `a` 应排在 `b` 前面,返回负数;
- 若 `a` 应排在 `b` 后面,返回正数;
- 若 `a` 和 `b` 相等,返回 0。
下面是一个例子,演示了如何使用 `qsort` 函数来排序字符串:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int cmp(const void *a, const void *b) {
const char *sa = *(const char **)a;
const char *sb = *(const char **)b;
return strcmp(sa, sb);
}
int main() {
const int n = 5;
char *s[n] = {"hello", "world", "apple", "banana", "cat"};
qsort(s, n, sizeof(char *), cmp);
for (int i = 0; i < n; ++i) {
printf("%s\n", s[i]);
}
return 0;
}
```
输出结果为:
```
apple
banana
cat
hello
world
```
如果需要按照字符串中每个字符的 ASCII 值排序,则可以修改比较函数实现。下面是一个例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int cmp(const void *a, const void *b) {
const char *sa = *(const char **)a;
const char *sb = *(const char **)b;
const int la = strlen(sa);
const int lb = strlen(sb);
const int len = la < lb ? la : lb;
for (int i = 0; i < len; ++i) {
if (sa[i] != sb[i]) {
return sa[i] - sb[i];
}
}
return la - lb;
}
int main() {
const int n = 5;
char *s[n] = {"hello", "world", "apple", "banana", "cat"};
qsort(s, n, sizeof(char *), cmp);
for (int i = 0; i < n; ++i) {
printf("%s\n", s[i]);
}
return 0;
}
```
输出结果为:
```
apple
banana
cat
hello
world
```
阅读全文
相关推荐
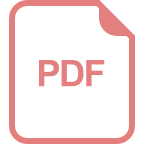
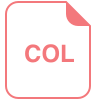
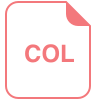
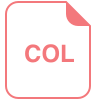
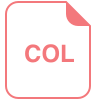
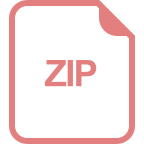
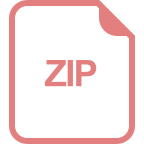
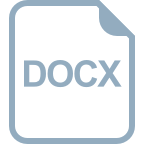
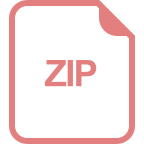