解释一下这些代码 import cv2 img = cv2.imread('image.png') hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) lower_red = np.array([30,150,50]) upper_red = np.array([255,255,180]) mask = cv2.inRange(hsv, lower_red, upper_red) res = cv2.bitwise_and(img,img, mask= mask) cv2.imshow('img',img) cv2.imshow('mask',mask) cv2.imshow('res',res) cv2.waitKey(0) cv2.destroyAllWindows()
时间: 2023-03-06 21:56:54 浏览: 43
这些代码可以用来检测图像中的红色部分,并在图像上显示出红色部分。它先从图像中读取数据,然后将其转换为HSV颜色空间,定义红色的范围,使用inRange函数检测出红色部分,然后用bitwise_and函数显示红色部分,最后使用waitKey和destroyAllWindows函数来停止显示图像。
相关问题
import cv2 import numpy as np # 加载图像 img = cv2.imread('color_blind_road_2.png') # 转换为灰度图像 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 直线检测 edges = cv2.Canny(gray, 50, 150, apertureSize=3) lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=100, maxLineGap=10) for line in lines: x1, y1, x2, y2 = line[0] cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2) # 阈值分割 ret, thresh = cv2.threshold(gray, 150, 255, cv2.THRESH_BINARY) thresh = cv2.medianBlur(thresh, 5) # 彩色连续性空间分割 hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) h, s, v = cv2.split(hsv) mask = cv2.inRange(h, 0, 20) | cv2.inRange(h, 160, 180) mask = cv2.bitwise_and(thresh, mask) # 纹理分割 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) texture = cv2.Canny(gray, 100, 200, apertureSize=3) texture = cv2.dilate(texture, None, iterations=3) texture = cv2.erode(texture, None, iterations=3) texture = cv2.bitwise_and(thresh, texture) # 显示结果 cv2.imshow('img', img) cv2.imshow('thresh', thresh) cv2.imshow('color', mask) cv2.imshow('texture', texture) cv2.waitKey(0) cv2.destroyAllWindows()改进代码
以下是对代码的一些改进建议:
1. 在 import 语句之间添加空格,使代码更易于阅读。
2. 使用函数封装代码,将不同的操作分别放入不同的函数中,可以使代码更加清晰和易于维护。
3. 添加异常处理,以避免因为文件路径或读取失败而导致程序崩溃。
4. 将一些常量或者需要调整的参数提取出来,以方便后续调整和修改。
5. 添加注释,以便代码的阅读和理解。
6. 使用 with 语句释放资源。
改进后的代码如下:
```python
import cv2
import numpy as np
def load_image(path):
"""加载图片"""
try:
img = cv2.imread(path)
return img
except Exception as e:
print(e)
return None
def gray_transform(img):
"""灰度转换"""
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
return gray
def edge_detection(img, threshold1=50, threshold2=150, apertureSize=3):
"""边缘检测"""
edges = cv2.Canny(img, threshold1, threshold2, apertureSize=apertureSize)
return edges
def line_detection(img, edges, threshold=100, minLineLength=100, maxLineGap=10):
"""直线检测"""
lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=threshold, minLineLength=minLineLength, maxLineGap=maxLineGap)
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
return img
def threshold_segmentation(img, threshold=150):
"""阈值分割"""
ret, thresh = cv2.threshold(img, threshold, 255, cv2.THRESH_BINARY)
thresh = cv2.medianBlur(thresh, 5)
return thresh
def hsv_segmentation(img, lower_range, upper_range):
"""HSV颜色空间分割"""
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
mask = cv2.inRange(hsv, lower_range, upper_range)
return mask
def color_segmentation(img, thresh, lower_range1=(0, 100, 100), upper_range1=(20, 255, 255), lower_range2=(160, 100, 100), upper_range2=(180, 255, 255)):
"""颜色分割"""
mask1 = hsv_segmentation(img, lower_range1, upper_range1)
mask2 = hsv_segmentation(img, lower_range2, upper_range2)
mask = cv2.bitwise_or(mask1, mask2)
mask = cv2.bitwise_and(thresh, mask)
return mask
def texture_segmentation(img, thresh, threshold1=100, threshold2=200, iterations=3):
"""纹理分割"""
gray = gray_transform(img)
texture = cv2.Canny(gray, threshold1, threshold2, apertureSize=3)
texture = cv2.dilate(texture, None, iterations=iterations)
texture = cv2.erode(texture, None, iterations=iterations)
texture = cv2.bitwise_and(thresh, texture)
return texture
def show_image(img, winname='image'):
"""显示图片"""
cv2.imshow(winname, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
if __name__ == '__main__':
# 加载图片
img = load_image('color_blind_road_2.png')
if img is None:
exit()
# 灰度转换
gray = gray_transform(img)
# 边缘检测
edges = edge_detection(gray)
# 直线检测
img = line_detection(img, edges)
# 阈值分割
thresh = threshold_segmentation(gray)
# 颜色分割
mask = color_segmentation(img, thresh)
# 纹理分割
texture = texture_segmentation(img, thresh)
# 显示结果
show_image(img, 'img')
show_image(thresh, 'thresh')
show_image(mask, 'color')
show_image(texture, 'texture')
```
import cv2 import numpy as np def retinex(img, sigma_list): retinex = np.zeros_like(img) for sigma in sigma_list: img_blur = cv2.GaussianBlur(img, (0, 0), sigma) img_blur = np.where(img_blur == 0, 0.1, img_blur) retinex += np.log10(img) - np.log10(img_blur) retinex = retinex / len(sigma_list) retinex = np.where(retinex < 0, 0, retinex) retinex = np.where(retinex > 1, 1, retinex) return retinex def enhance_image(img): hsi = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) h, s, i = cv2.split(hsi) i_enhanced = retinex(i.astype(np.float64), [15, 80, 250]) i_enhanced = (i_enhanced * 255).astype(np.uint8) s_enhanced = cv2.equalizeHist(s) hsi_enhanced = cv2.merge([h, s_enhanced, i_enhanced]) enhanced_img = cv2.cvtColor(hsi_enhanced, cv2.COLOR_HSV2BGR) return enhanced_img img = cv2.imread('low_illumination_1.png') enhanced_img = enhance_image(img) cv2.imshow('Enhanced Image', enhanced_img) cv2.waitKey(0) cv2.destroyAllWindows()
这是一段基于Retinex算法对图像进行增强的Python代码。Retinex算法是一种用于图像增强的经典算法,它可以增强图像的对比度和颜色鲜艳度,同时可以保留图像的细节信息。该算法的基本思想是将图像分解为多个尺度的高斯模糊图像,然后通过计算不同尺度的高斯模糊图像与原始图像之间的差异来获得图像的反射性和色彩性信息。在这段代码中,首先将输入图像转换为HSI颜色空间,然后对亮度通道进行Retinex增强处理,对饱和度通道进行直方图均衡化处理,最后将三个通道重新组合成BGR颜色空间的图像输出。
相关推荐
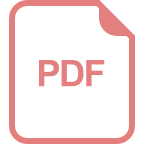
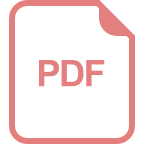
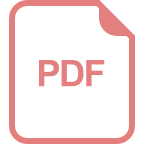












