antdesignvue 1.7 table keydown事件
时间: 2023-10-11 22:08:43 浏览: 195
Ant Design Vue 1.7 的表格组件(Table)并没有提供直接的键盘事件绑定方法,但是可以通过在表格的 `@keydown.native` 事件中监听键盘事件来实现相应的功能。
例如,如果想要在表格中按下 Enter 键时执行某个操作,可以在表格中添加如下代码:
```html
<template>
<a-table @keydown.native.enter="handleEnter">
<!-- 表格列的定义 -->
</a-table>
</template>
```
这里的 `handleEnter` 方法就是在按下 Enter 键时要执行的操作。需要注意的是,如果在表格中使用了分页组件,那么在输入分页页码时也会触发 Enter 键,这时需要根据具体情况做出相应的处理。
另外,如果需要监听其他的键盘事件,可以参考以下键码对应表:
- Enter:13
- Esc:27
- 上箭头:38
- 下箭头:40
- 左箭头:37
- 右箭头:39
在监听键盘事件时,可以通过 `event.keyCode` 属性获取当前按下的键码。
相关问题
antdesignvue 1.7 table 键盘事件
Ant Design Vue 1.7 的表格组件 `a-table` 支持键盘事件。具体可以监听以下事件:
- `@keydown`:当用户按下键盘上的任意键时触发,可以使用 `event.keyCode` 获取按下的键的编码。
- `@keyup`:当用户释放键盘上的任意键时触发,可以使用 `event.keyCode` 获取释放的键的编码。
- `@keypress`:当用户按下并释放键盘上的任意键时触发,可以使用 `event.keyCode` 获取按下的键的编码。
在表格中,可以使用 `rowKey` 属性来指定每一行的唯一键值,也可以使用 `selection` 属性来开启行选择功能。当选中行后,可以使用 `@keydown.enter` 事件监听回车键,进一步处理选中行的数据。
以下是一个示例代码:
```html
<template>
<a-table :columns="columns" :data-source="dataSource" row-key="id" selection v-model="selectedRowKeys" @keydown.enter="handleEnter"></a-table>
</template>
<script>
export default {
data() {
return {
columns: [
{
title: 'Name',
dataIndex: 'name',
},
{
title: 'Age',
dataIndex: 'age',
},
{
title: 'Address',
dataIndex: 'address',
},
],
dataSource: [
{
id: 1,
name: 'John Brown',
age: 32,
address: 'New York No. 1 Lake Park',
},
{
id: 2,
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
},
{
id: 3,
name: 'Joe Black',
age: 32,
address: 'Sidney No. 1 Lake Park',
},
],
selectedRowKeys: [],
};
},
methods: {
handleEnter() {
const selectedRow = this.dataSource.find(item => item.id === this.selectedRowKeys[0]);
console.log(selectedRow);
},
},
};
</script>
```
在上述示例中,我们监听了 `@keydown.enter` 事件,当用户按下回车键时,会触发 `handleEnter` 方法,该方法会根据选中行的唯一键值 `id` 查找到该行数据,并打印到控制台上。
antdesignvue1.7 table 上下键切换选中行
在 Ant Design Vue 1.7 中,可以通过监听键盘事件来实现在 Table 中使用上下键切换选中行的功能。具体实现步骤如下:
1. 在 Table 组件上添加 ref 属性,方便在代码中引用该组件。
```html
<template>
<a-table ref="myTable" :columns="columns" :data-source="data"></a-table>
</template>
```
2. 在组件的 mounted 钩子函数中,给表格绑定键盘事件。
```javascript
mounted() {
this.$refs.myTable.$el.addEventListener('keydown', this.handleKeyDown);
},
```
3. 在组件中定义键盘事件的回调函数 handleKeyDown,当按下上下键时,根据当前选中行的 index 值,计算出下一个需要选中的行的 index 值,并使用 Table 组件提供的 setSelectedRowKeys 方法来更新选中行。
```javascript
methods: {
handleKeyDown(event) {
const { keyCode } = event;
const { selectedRowKeys } = this.$refs.myTable;
if (keyCode === 38 || keyCode === 40) { // 上下键
event.preventDefault(); // 阻止默认事件,避免滚动条跟随键盘滚动
let nextIndex = -1;
if (selectedRowKeys.length === 0) { // 如果没有选中行,则选中第一行
nextIndex = 0;
} else { // 否则根据当前选中行的 index 计算出下一个需要选中的行的 index
const currentIndex = this.data.findIndex(item => item.key === selectedRowKeys[0]);
if (keyCode === 38) { // 上键
nextIndex = currentIndex > 0 ? currentIndex - 1 : 0;
} else if (keyCode === 40) { // 下键
nextIndex = currentIndex < this.data.length - 1 ? currentIndex + 1 : this.data.length - 1;
}
}
// 更新选中行
const nextRow = this.data[nextIndex];
this.$refs.myTable.setSelectedRowKeys([nextRow.key]);
}
},
},
```
完整代码如下:
```html
<template>
<a-table ref="myTable" :columns="columns" :data-source="data"></a-table>
</template>
<script>
export default {
data() {
return {
data: [
{ key: '1', name: 'John Brown', age: 32, address: 'New York No. 1 Lake Park' },
{ key: '2', name: 'Jim Green', age: 42, address: 'London No. 1 Lake Park' },
{ key: '3', name: 'Joe Black', age: 32, address: 'Sidney No. 1 Lake Park' },
{ key: '4', name: 'Disabled User', age: 99, address: 'Sidney No. 1 Lake Park' },
],
columns: [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
],
};
},
mounted() {
this.$refs.myTable.$el.addEventListener('keydown', this.handleKeyDown);
},
beforeDestroy() {
this.$refs.myTable.$el.removeEventListener('keydown', this.handleKeyDown);
},
methods: {
handleKeyDown(event) {
const { keyCode } = event;
const { selectedRowKeys } = this.$refs.myTable;
if (keyCode === 38 || keyCode === 40) { // 上下键
event.preventDefault(); // 阻止默认事件,避免滚动条跟随键盘滚动
let nextIndex = -1;
if (selectedRowKeys.length === 0) { // 如果没有选中行,则选中第一行
nextIndex = 0;
} else { // 否则根据当前选中行的 index 计算出下一个需要选中的行的 index
const currentIndex = this.data.findIndex(item => item.key === selectedRowKeys[0]);
if (keyCode === 38) { // 上键
nextIndex = currentIndex > 0 ? currentIndex - 1 : 0;
} else if (keyCode === 40) { // 下键
nextIndex = currentIndex < this.data.length - 1 ? currentIndex + 1 : this.data.length - 1;
}
}
// 更新选中行
const nextRow = this.data[nextIndex];
this.$refs.myTable.setSelectedRowKeys([nextRow.key]);
}
},
},
};
</script>
```
阅读全文
相关推荐
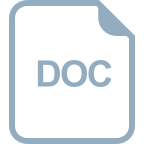
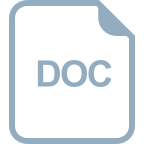
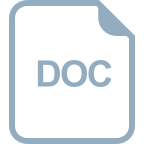













