写一个C语言的俄罗斯方块代码
时间: 2023-12-28 22:05:45 浏览: 62
以下是一个简单的C语言俄罗斯方块代码示例,仅供参考:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 12
#define HEIGHT 20
#define BLOCK_SIZE 4
int board[HEIGHT][WIDTH];
void init_board() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
board[i][j] = (j == 0 || j == WIDTH - 1 || i == HEIGHT - 1) ? 1 : 0;
}
}
}
int check_collision(int block[BLOCK_SIZE][BLOCK_SIZE], int x, int y) {
int i, j;
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
if (block[i][j] && board[y + i][x + j]) {
return 1;
}
}
}
return 0;
}
void draw_block(int block[BLOCK_SIZE][BLOCK_SIZE], int x, int y) {
int i, j;
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
if (block[i][j]) {
board[y + i][x + j] = 1;
}
}
}
}
void clear_line() {
int i, j, k;
for (i = HEIGHT - 2; i > 0; i--) {
for (j = 1; j < WIDTH - 1; j++) {
if (!board[i][j]) {
break;
}
}
if (j == WIDTH - 1) {
for (k = i; k > 0; k--) {
memcpy(board[k], board[k - 1], sizeof(board[k]));
}
i++;
}
}
}
void draw_board() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
if (board[i][j]) {
printf("[]");
} else {
printf(" ");
}
}
printf("\n");
}
}
int main() {
int blocks[7][4][4] = {
{{1,1,0,0}, {1,1,0,0}, {0,0,0,0}, {0,0,0,0}}, // O
{{0,1,1,0}, {1,1,0,0}, {0,0,0,0}, {0,0,0,0}}, // Z
{{1,1,0,0}, {0,1,1,0}, {0,0,0,0}, {0,0,0,0}}, // S
{{0,1,0,0}, {1,1,1,0}, {0,0,0,0}, {0,0,0,0}}, // T
{{1,0,0,0}, {1,1,1,0}, {0,0,0,0}, {0,0,0,0}}, // L
{{0,0,1,0}, {1,1,1,0}, {0,0,0,0}, {0,0,0,0}}, // J
{{0,1,0,0}, {1,1,1,0}, {0,0,0,0}, {0,0,0,0}} // I
};
srand((unsigned) time(NULL));
init_board();
while (1) {
int block_index = rand() % 7;
int block_x = WIDTH / 2 - 2;
int block_y = 0;
int block_rotation = 0;
int block[BLOCK_SIZE][BLOCK_SIZE];
memcpy(block, blocks[block_index][block_rotation], sizeof(block));
if (check_collision(block, block_x, block_y)) {
break;
}
while (1) {
system("cls");
draw_block(block, block_x, block_y);
draw_board();
Sleep(100);
if (kbhit()) {
char ch = getch();
if (ch == 'a' && !check_collision(block, block_x - 1, block_y)) {
block_x--;
} else if (ch == 'd' && !check_collision(block, block_x + 1, block_y)) {
block_x++;
} else if (ch == 'w') {
int next_rotation = (block_rotation + 1) % 4;
int next_block[BLOCK_SIZE][BLOCK_SIZE];
memcpy(next_block, blocks[block_index][next_rotation], sizeof(next_block));
if (!check_collision(next_block, block_x, block_y)) {
block_rotation = next_rotation;
memcpy(block, next_block, sizeof(block));
}
} else if (ch == 's') {
while (!check_collision(block, block_x, block_y + 1)) {
block_y++;
}
}
}
if (check_collision(block, block_x, block_y + 1)) {
draw_block(block, block_x, block_y);
clear_line();
break;
}
block_y++;
}
int i, j;
for (i = 1; i < HEIGHT - 1; i++) {
for (j = 1; j < WIDTH - 1; j++) {
if (board[i][j]) {
printf("[]");
} else {
printf(" ");
}
}
printf("\n");
}
printf("Game Over!\n");
}
return 0;
}
```
阅读全文
相关推荐
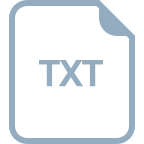
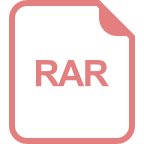
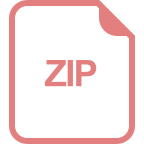
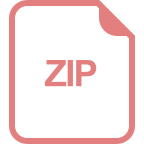
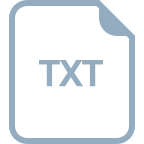
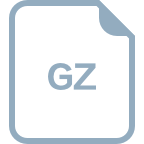
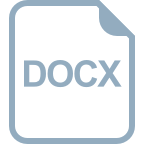
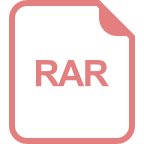
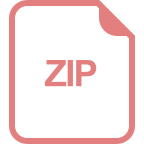
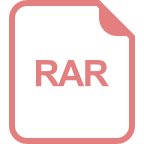

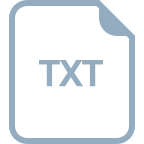
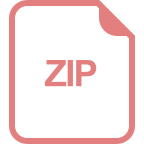