C语言建立二叉树实现先序中序后序遍历
时间: 2023-10-17 12:05:38 浏览: 58
以下是用 C 语言建立二叉树并实现先序、中序、后序遍历的代码:
```c
#include <stdio.h>
#include <stdlib.h>
//定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
//创建二叉树
TreeNode* createTree() {
TreeNode *root = NULL;
int val;
scanf("%d", &val);
if (val != -1) { //输入-1表示该节点为空
root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = val;
root->left = createTree();
root->right = createTree();
}
return root;
}
//先序遍历
void preOrder(TreeNode *root) {
if (root != NULL) {
printf("%d ", root->val);
preOrder(root->left);
preOrder(root->right);
}
}
//中序遍历
void inOrder(TreeNode *root) {
if (root != NULL) {
inOrder(root->left);
printf("%d ", root->val);
inOrder(root->right);
}
}
//后序遍历
void postOrder(TreeNode *root) {
if (root != NULL) {
postOrder(root->left);
postOrder(root->right);
printf("%d ", root->val);
}
}
int main() {
printf("请输入二叉树的先序遍历,-1表示该节点为空:\n");
TreeNode *root = createTree();
printf("二叉树的先序遍历为:");
preOrder(root);
printf("\n");
printf("二叉树的中序遍历为:");
inOrder(root);
printf("\n");
printf("二叉树的后序遍历为:");
postOrder(root);
printf("\n");
return 0;
}
```
输入格式:
先序遍历的每个节点值,-1表示该节点为空。
输出格式:
二叉树的先序、中序、后序遍历结果。
示例:
输入:
```
1 2 -1 -1 3 4 -1 -1 5 -1 -1
```
输出:
```
二叉树的先序遍历为:1 2 3 4 5
二叉树的中序遍历为:2 1 4 3 5
二叉树的后序遍历为:2 4 5 3 1
```
相关推荐
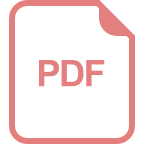














