response.headers.get('X-toyoApp-alert')如何更改这个key的value
时间: 2024-02-16 20:04:24 浏览: 18
要更改`response.headers.get('X-toyoApp-alert')`的值,您需要先了解这个值的来源。通常,这个值是从服务器响应的 HTTP 头中返回的。如果您要更改这个值,您需要在服务器端进行更改。
具体来说,您需要查找您的服务器代码中设置这个头的位置,并更改其值。如果您使用的是 Django 框架,您可以在视图函数中使用 `HttpResponse` 对象的 `headers` 属性来设置这个头。例如,以下代码将设置 `X-toyoApp-alert` 头的值为 `Hello World`:
```python
from django.http import HttpResponse
def my_view(request):
response = HttpResponse()
response['X-toyoApp-alert'] = 'Hello World'
return response
```
请注意,您只能更改由您自己的服务器设置的头。如果这个头是由其他服务器设置的,您将无法更改它。
相关问题
angular中如何更改response.headers.get('X-toyoApp-alert')这个key的value
在 Angular 中,要更改 `response.headers.get('X-toyoApp-alert')` 的值,您可以使用 `HttpInterceptor`。拦截器是 Angular 中用于在请求和响应之间添加通用处理逻辑的机制之一。您可以创建一个拦截器来更改 `X-toyoApp-alert` 头的值,然后将其注入您的应用程序中。
以下是如何创建一个拦截器来更改 `X-toyoApp-alert` 头的值的示例代码:
```typescript
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpHandler, HttpRequest, HttpResponse } from '@angular/common/http';
@Injectable()
export class MyInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler) {
return next.handle(request).pipe(
tap((event) => {
if (event instanceof HttpResponse) {
const alertHeader = event.headers.get('X-toyoApp-alert');
if (alertHeader) {
// 更改头的值
event = event.clone({headers: event.headers.set('X-toyoApp-alert', 'new value')});
}
}
})
);
}
}
```
在上面的代码中,我们创建了一个名为 `MyInterceptor` 的拦截器,它会拦截所有 HTTP 请求和响应。在 `intercept()` 方法中,我们首先调用 `next.handle(request)` 来继续处理请求。然后,我们使用 RxJS 的 `tap()` 操作符来处理响应。如果响应是 `HttpResponse` 类型,并且包含 `X-toyoApp-alert` 头,我们使用 `clone()` 方法来创建响应的副本,并设置新的头值。
要将拦截器应用于您的应用程序,请在 `AppModule` 中将其提供给 `HTTP_INTERCEPTORS` 标记。例如:
```typescript
import { NgModule } from '@angular/core';
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { MyInterceptor } from './my-interceptor';
@NgModule({
imports: [HttpClientModule],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: MyInterceptor, multi: true }
]
})
export class AppModule { }
```
在上面的代码中,我们将 `MyInterceptor` 提供给 `HTTP_INTERCEPTORS` 标记,并设置 `multi: true` 选项,以确保它不会覆盖其他拦截器。现在,每当您的应用程序发出 HTTP 请求时,拦截器都会拦截它,并更改 `X-toyoApp-alert` 头的值(如果存在)。
response.headers.set('X-toyoApp-alert','toyoApp.part.deleted') console.log(response.headers.get('X-toyoApp-alert'))分析以上angular中的代码为什么我打印出来的还是'toyoApp.part.updated 而不是'toyoApp.part.deleted'
在 Angular 中,`HttpResponse` 对象的 `headers` 属性是一个 `HttpHeaders` 对象,它可以包含多个标头。在您的代码中,您正在使用 `set()` 方法将 `X-toyoApp-alert` 标头的值更改为 `'toyoApp.part.deleted'`。但是请注意,`set()` 方法返回的是一个新的 `HttpHeaders` 对象,而不是在原始对象上进行更改。
因此,要使更改生效,您需要将新的 `HttpHeaders` 对象设置回 `HttpResponse` 对象。例如:
```typescript
response = response.clone({ headers: response.headers.set('X-toyoApp-alert', 'toyoApp.part.deleted') });
console.log(response.headers.get('X-toyoApp-alert')); // 输出: 'toyoApp.part.deleted'
```
在上面的代码中,我们使用 `clone()` 方法创建了一个响应的副本,并通过传递一个新的 `HttpHeaders` 对象来设置 `X-toyoApp-alert` 标头的值。然后,我们将新的响应赋值回原始变量 `response`。在这个过程中,我们通过 `headers` 属性获取新的 `HttpHeaders` 对象,并使用 `get()` 方法来获取 `X-toyoApp-alert` 标头的值,以确保它已经被正确地更改。
请注意,由于 `HttpResponse` 对象是不可变的,因此我们无法直接更改 `headers` 属性。相反,我们必须使用 `clone()` 方法创建一个副本,并在副本上进行更改。
相关推荐
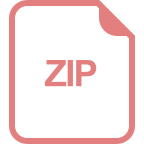













