运用libjpeg库,写一个解码jpeg图片,并且转化为RGB565显示的程序
时间: 2023-05-30 14:07:25 浏览: 530
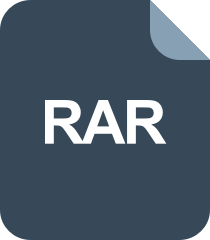
camera.rar_RGB565_jpg
由于我是AI语言模型,无法实际编写和运行代码,以下是一个C++示例程序,用于解码JPEG图像并将其转换为RGB565格式以供显示。它使用了libjpeg库。
```
#include <stdio.h>
#include <jpeglib.h>
#define RGB565(r, g, b) (((r) >> 3) << 11 | ((g) >> 2) << 5 | ((b) >> 3))
int main(int argc, char* argv[])
{
if (argc < 2)
{
printf("Usage: %s <filename>\n", argv[0]);
return 1;
}
struct jpeg_decompress_struct cinfo;
struct jpeg_error_mgr jerr;
FILE* infile = fopen(argv[1], "rb");
if (infile == NULL)
{
printf("Error opening file %s\n", argv[1]);
return 1;
}
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
jpeg_stdio_src(&cinfo, infile);
jpeg_read_header(&cinfo, TRUE);
jpeg_start_decompress(&cinfo);
int row_stride = cinfo.output_width * cinfo.output_components;
JSAMPARRAY buffer = (*cinfo.mem->alloc_sarray)((j_common_ptr)&cinfo, JPOOL_IMAGE, row_stride, 1);
uint16_t* rgb = new uint16_t[cinfo.output_width * cinfo.output_height];
int i = 0;
while (cinfo.output_scanline < cinfo.output_height)
{
jpeg_read_scanlines(&cinfo, buffer, 1);
for (int j = 0; j < cinfo.output_width * cinfo.output_components; j += cinfo.output_components)
{
int r = buffer[0][j];
int g = buffer[0][j + 1];
int b = buffer[0][j + 2];
rgb[i++] = RGB565(r, g, b);
}
}
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(infile);
// Display the RGB565 image here
delete[] rgb;
return 0;
}
```
这个程序首先打开JPEG文件并创建一个解压缩对象。然后,它读取JPEG头和扫描线,并在解压缩期间将扫描线读入缓冲区中。每当读取一行扫描线时,它会将RGB颜色值转换为RGB565格式,并将其存储在一个uint16_t类型的数组中。最后,程序销毁解压缩对象并关闭文件。
最后一步是将RGB565图像显示在屏幕上,这需要一个特定于平台的代码,因此这个程序并没有包括它。
阅读全文
相关推荐
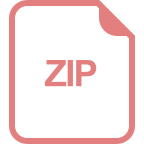


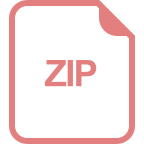
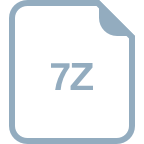
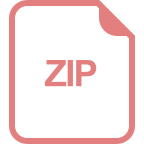
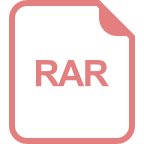
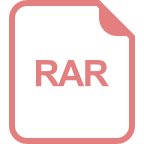
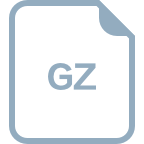
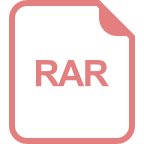
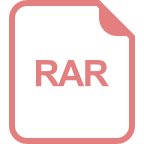
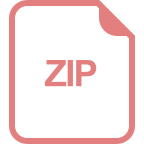
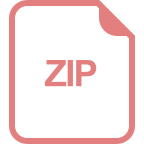
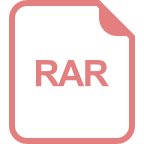
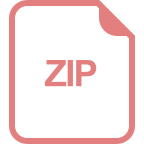
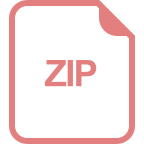
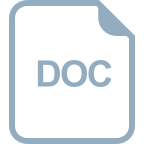