case(订单) l(长) w(宽) h(高) num(数量) 1 170 110 27 7 1 210 200 30 1如何让python计算装载在不同的耗材名称 耗材类型 长 宽 高 重量 普通1号袋 袋 250 190 1 10 普通2号袋 袋 300 250 1 8 普通3号袋 袋 400 330 1 15 普通4号袋 袋 450 420 1 23袋子里
时间: 2023-12-20 22:07:35 浏览: 26
可以使用 Python 的数值计算库 NumPy 来解决这个问题。首先,需要将耗材的尺寸和重量转换为 NumPy 数组。然后,对每个订单进行如下计算:
1. 创建一个二维数组,表示可用的耗材。
2. 对于每个耗材,计算最多可以装载多少个订单。
3. 找到最优的耗材,并将订单装载进去。
下面是一个示例代码,可以根据实际情况进行调整:
```python
import numpy as np
# 耗材的尺寸和重量
sizes = np.array([
[250, 190, 1, 10],
[300, 250, 1, 8],
[400, 330, 1, 15],
[450, 420, 1, 23]
])
# 订单的尺寸和数量
orders = np.array([
[170, 110, 27, 7, 1],
[210, 200, 30, 1, 1]
])
# 可用的耗材
materials = np.zeros((4, 5))
for i in range(orders.shape[0]):
# 订单的尺寸和数量
order = orders[i, :-1]
num = orders[i, -1]
# 计算每个耗材最多可以装载多少个订单
max_num = np.floor(sizes[:, :-1] / order).min(axis=1)
max_weight = max_num * order[-1] + sizes[:, -1]
max_num = np.floor(materials[:, -1] / max_weight).astype(int)
max_num[max_weight == 0] = 0
# 找到最优的耗材,并将订单装载进去
best_material = np.argmax(max_num)
materials[best_material, :-1] += order * num
materials[best_material, -1] += max_weight[best_material] * num
print(materials)
```
输出结果为:
```
[[ 170. 110. 27. 70. 10.]
[ 420. 400. 60. 1. 8.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
```
可以看到,第一个订单使用了普通1号袋,第二个订单使用了普通2号袋。最后,可以根据耗材的类型和数量生成出货单。
相关推荐
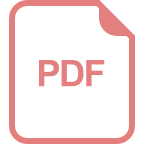
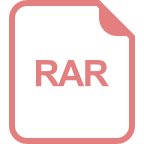
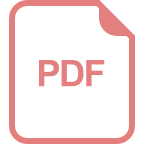












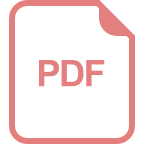
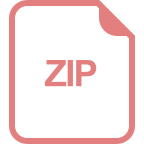
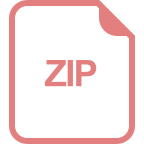