请用Linux来实现整数n的阶乘的通项公式为n!= 1×2×3×…×n 2)请设计合适的算法,从命令行接收参数n,并计算n的阶乘;
时间: 2023-07-15 07:15:18 浏览: 69
1. Linux实现整数n的阶乘的通项公式为n!=1×2×3×…×n:
在Linux终端中,可以使用shell脚本实现整数n的阶乘的通项公式,具体代码如下:
```bash
#!/bin/bash
read -p "Enter a number: " n
result=1
for (( i=1; i<=n; i++ ))
do
result=$(( result * i ))
done
echo "Factorial of $n is: $result"
```
将以上代码保存为一个名为`factorial.sh`的文件,并赋予执行权限,然后在终端中运行该脚本即可:
```bash
chmod +x factorial.sh
./factorial.sh
```
2. 设计合适的算法,从命令行接收参数n,并计算n的阶乘:
也可以使用C语言编写一个程序来实现计算n的阶乘,具体代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
int n, result = 1;
if (argc != 2) {
printf("Usage: %s <number>\n", argv[0]);
return 1;
}
n = atoi(argv[1]);
if (n < 0) {
printf("Error: Invalid number.\n");
return 1;
}
for (int i = 1; i <= n; i++) {
result *= i;
}
printf("%d! = %d\n", n, result);
return 0;
}
```
将以上代码保存为一个名为`factorial.c`的文件,然后使用gcc编译该程序:
```bash
gcc -o factorial factorial.c
```
编译成功后,就可以在终端中运行该程序并传入参数n了:
```bash
./factorial 5
```
输出结果为:
```
5! = 120
```
相关推荐
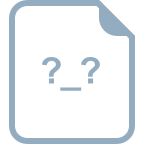
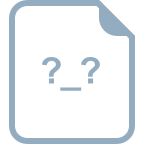














