Android AIDL实例
时间: 2023-08-18 18:24:58 浏览: 136
好的,以下是一个简单的Android AIDL实例:
1. 在你的Android Studio项目中,创建一个新的模块,并选择“Android Library”类型。
2. 在该模块中,创建一个名为“IMyAidlInterface”的AIDL接口文件,并在其中定义要使用的方法。例如:
```
// IMyAidlInterface.aidl
package com.example.myaidl;
// Declare any non-default types here with import statements
interface IMyAidlInterface {
int add(int a, int b);
}
```
3. 在同一模块中,创建一个实现AIDL接口的类。例如:
```
// MyAidlInterfaceImpl.java
package com.example.myaidl;
import android.os.RemoteException;
public class MyAidlInterfaceImpl extends IMyAidlInterface.Stub {
@Override
public int add(int a, int b) throws RemoteException {
return a + b;
}
}
```
4. 在你的应用程序中,创建一个Service,并在其中使用上面定义的AIDL接口和实现类。例如:
```
// MyService.java
package com.example.myaidl;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
public class MyService extends Service {
private MyAidlInterfaceImpl myAidlInterfaceImpl;
@Override
public void onCreate() {
super.onCreate();
myAidlInterfaceImpl = new MyAidlInterfaceImpl();
}
@Override
public IBinder onBind(Intent intent) {
return myAidlInterfaceImpl;
}
}
```
5. 在你的应用程序中,通过bindService()方法绑定MyService,并使用IMyAidlInterface接口中的方法。例如:
```
// MainActivity.java
package com.example.myaidl;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private static final String TAG = MainActivity.class.getSimpleName();
private IMyAidlInterface myAidlInterface;
private boolean isBound = false;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
myAidlInterface = IMyAidlInterface.Stub.asInterface(iBinder);
isBound = true;
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
myAidlInterface = null;
isBound = false;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button addButton = findViewById(R.id.add_button);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (isBound) {
try {
int a = 1;
int b = 2;
int result = myAidlInterface.add(a, b);
Log.d(TAG, "Result: " + result);
} catch (RemoteException e) {
e.printStackTrace();
}
}
}
});
}
@Override
protected void onStart() {
super.onStart();
Intent intent = new Intent(this, MyService.class);
bindService(intent, connection, Context.BIND_AUTO_CREATE);
}
@Override
protected void onStop() {
super.onStop();
if (isBound) {
unbindService(connection);
isBound = false;
}
}
}
```
这就是一个简单的Android AIDL实例,它演示了如何在应用程序中使用AIDL接口。
阅读全文
相关推荐
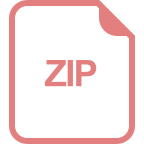
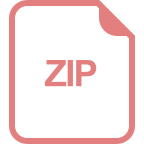
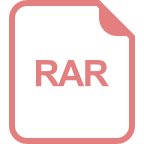
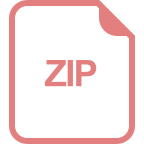
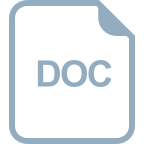
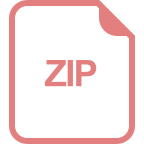
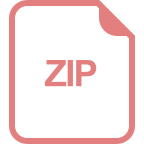
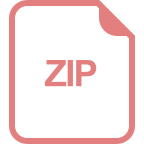
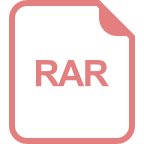
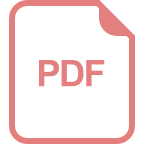
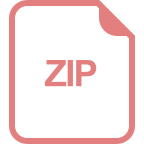
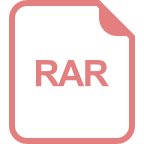
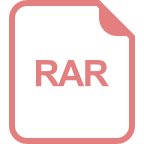