如何通过key对HashMap进行排序
时间: 2024-03-06 12:51:31 浏览: 36
在Java中,HashMap是一种无序的数据结构,因此不能直接对其进行排序。但是,可以通过将HashMap中的键值对转换成List,然后对List进行排序来实现对HashMap的排序。
具体步骤如下:
1. 将HashMap中的键值对转换成List,可以使用HashMap中的entrySet()方法,将其转换成Set,再使用ArrayList的构造函数将其转换成List。
2. 对List进行排序,可以使用Collections类中的sort()方法,并指定排序规则。这里可以使用Comparator接口的实现类来指定排序规则,也可以直接对值或键进行排序。
3. 将排序后的List转换回HashMap,可以使用HashMap的构造函数,并传入排序后的List作为参数。
以下是示例代码:
```java
HashMap<String, Integer> map = new HashMap<>();
map.put("c", 3);
map.put("a", 1);
map.put("d", 4);
map.put("b", 2);
List<Map.Entry<String, Integer>> list = new ArrayList<>(map.entrySet());
Collections.sort(list, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o1.getKey().compareTo(o2.getKey());
}
});
HashMap<String, Integer> sortedMap = new LinkedHashMap<>();
for (Map.Entry<String, Integer> entry : list) {
sortedMap.put(entry.getKey(), entry.getValue());
}
System.out.println(sortedMap); // 输出:{a=1, b=2, c=3, d=4}
```
在示例代码中,通过将HashMap中的键值对转换成List,并使用Collections类的sort()方法对List进行排序,指定了按照键的升序排序规则。最后,将排序后的List转换回HashMap。注意,这里使用了LinkedHashMap,是因为HashMap是无序的,如果想保留排序结果,需要使用有序的Map。
相关推荐
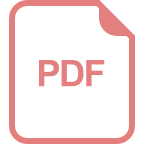
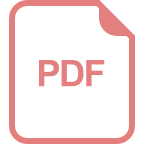
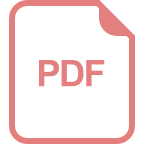














