请帮我用c++语言完成一个超市商品管理系统,题目是超市中商品分为四类,分别是食品、化妆品、日用品和饮料。每种商品都包含商品名称、 价格、库存量和生产厂家、品牌等信息。 主要完成对商品的销售、统计和简单管理。
时间: 2023-07-10 13:13:20 浏览: 236
好的,我可以为您提供一个基本的超市商品管理系统的代码框架,您可以参考它来完成具体的实现。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 商品类
class Product {
public:
string name; // 商品名称
double price; // 商品价格
int stock; // 商品库存量
string manufacturer;// 生产厂家
string brand; // 商品品牌
string type; // 商品类别
// 构造函数
Product(string n, double p, int s, string m, string b, string t) {
name = n;
price = p;
stock = s;
manufacturer = m;
brand = b;
type = t;
}
// 显示商品信息
void show() {
cout << "商品名称:" << name << endl;
cout << "商品价格:" << price << endl;
cout << "商品库存:" << stock << endl;
cout << "生产厂家:" << manufacturer << endl;
cout << "商品品牌:" << brand << endl;
cout << "商品类别:" << type << endl;
}
};
// 超市类
class Supermarket {
public:
vector<Product> products; // 商品列表
// 添加商品
void add() {
string n, m, b, t;
double p;
int s;
cout << "请输入商品名称:";
cin >> n;
cout << "请输入商品价格:";
cin >> p;
cout << "请输入商品库存:";
cin >> s;
cout << "请输入生产厂家:";
cin >> m;
cout << "请输入商品品牌:";
cin >> b;
cout << "请输入商品类别:";
cin >> t;
Product product(n, p, s, m, b, t);
products.push_back(product);
cout << "商品添加成功!" << endl;
}
// 显示商品列表
void show() {
for (int i = 0; i < products.size(); i++) {
products[i].show();
cout << endl;
}
}
// 统计商品总数
int count() {
return products.size();
}
// 搜索商品
void search() {
string n;
cout << "请输入要搜索的商品名称:";
cin >> n;
for (int i = 0; i < products.size(); i++) {
if (products[i].name == n) {
products[i].show();
return;
}
}
cout << "没有找到该商品!" << endl;
}
// 修改商品信息
void modify() {
string n;
cout << "请输入要修改的商品名称:";
cin >> n;
for (int i = 0; i < products.size(); i++) {
if (products[i].name == n) {
products.erase(products.begin() + i); // 删除原来的商品信息
// 重新添加商品信息
add();
cout << "商品修改成功!" << endl;
return;
}
}
cout << "没有找到该商品!" << endl;
}
// 删除商品
void remove() {
string n;
cout << "请输入要删除的商品名称:";
cin >> n;
for (int i = 0; i < products.size(); i++) {
if (products[i].name == n) {
products.erase(products.begin() + i);
cout << "商品删除成功!" << endl;
return;
}
}
cout << "没有找到该商品!" << endl;
}
// 销售商品
void sell() {
string n;
cout << "请输入要销售的商品名称:";
cin >> n;
for (int i = 0; i < products.size(); i++) {
if (products[i].name == n) {
if (products[i].stock > 0) {
products[i].stock--;
cout << "商品销售成功!" << endl;
} else {
cout << "商品已售罄!" << endl;
}
return;
}
}
cout << "没有找到该商品!" << endl;
}
};
int main() {
Supermarket supermarket;
while (true) {
cout << "请选择操作:" << endl;
cout << "1.添加商品" << endl;
cout << "2.显示商品列表" << endl;
cout << "3.统计商品总数" << endl;
cout << "4.搜索商品" << endl;
cout << "5.修改商品信息" << endl;
cout << "6.删除商品" << endl;
cout << "7.销售商品" << endl;
cout << "8.退出程序" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
supermarket.add();
break;
case 2:
supermarket.show();
break;
case 3:
cout << "商品总数:" << supermarket.count() << endl;
break;
case 4:
supermarket.search();
break;
case 5:
supermarket.modify();
break;
case 6:
supermarket.remove();
break;
case 7:
supermarket.sell();
break;
case 8:
cout << "程序已退出!" << endl;
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
这是一个基本的超市商品管理系统,包含了添加商品、显示商品列表、统计商品总数、搜索商品、修改商品信息、删除商品和销售商品等功能。您可以根据您的实际需求进行修改和扩展。希望对您有帮助!
阅读全文
相关推荐
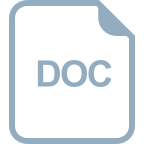
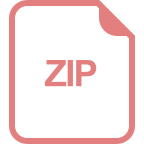
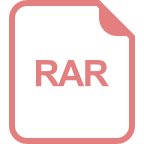
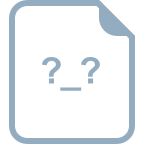
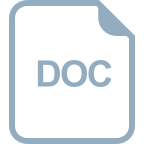
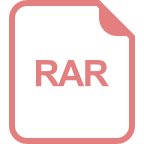
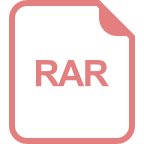
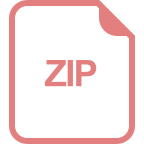
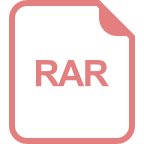
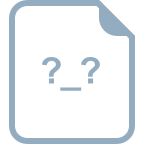
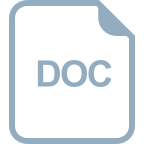
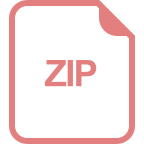
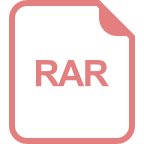
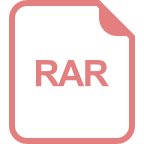
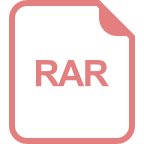