16位转8位图像c++程序代码是什么
时间: 2023-09-07 15:05:00 浏览: 120
### 回答1:
16位转8位图像的 C 程序代码可能长这样:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
// 读入图像文件
FILE *fp_in = fopen("in.bmp", "rb");
if (fp_in == NULL) {
perror("fopen");
return 1;
}
fseek(fp_in, 0, SEEK_END);
long size_in = ftell(fp_in);
rewind(fp_in);
unsigned char *bmp_in = malloc(size_in);
if (bmp_in == NULL) {
perror("malloc");
return 1;
}
fread(bmp_in, size_in, 1, fp_in);
fclose(fp_in);
// 解析 BMP 文件头
int offset = *(int *)(bmp_in + 10); // 像素数据偏移量
int width = *(int *)(bmp_in + 18); // 宽度
int height = *(int *)(bmp_in + 22); // 高度
int depth = *(int *)(bmp_in + 28); // 位深度
if (depth != 16) {
fprintf(stderr, "Error: Unsupported image depth %d\n", depth);
return 1;
}
// 创建输出图像
unsigned char *bmp_out = malloc(size_in);
if (bmp_out == NULL) {
perror("malloc");
return 1;
}
memcpy(bmp_out, bmp_in, offset); // 复制文件头
// 转换像素
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
// 读入 16 位像素
int i = offset + (y * width + x) * 2;
unsigned short pixel_in = *(unsigned short *)(bmp_in + i);
// 转换为 8 位像素
unsigned char pixel_out = (pixel_in >> 8) & 0xFF;
// 写出 8 位像素
*(bmp_out + i) = pixel_out;
*(bmp_out + i + 1) = pixel_out;
}
}
// 写出图像文件
FILE *fp_out = fopen("out.bmp", "wb");
if
### 回答2:
要实现16位转8位图像c的程序代码,首先需要了解图像的数据表示以及转换的原理。
16位图像通常使用无符号整型数表示,像素值范围为0-65535。而8位图像使用无符号整型数表示,像素值范围为0-255。
下面是一个简单的示例代码,实现16位图像转换为8位图像的功能:
```c
#include <stdio.h>
// 定义图像的宽度和高度
#define WIDTH 640
#define HEIGHT 480
void convert16to8(const unsigned short* image16, unsigned char* image8) {
for (int i = 0; i < WIDTH * HEIGHT; i++) {
// 将16位像素值取高8位赋值给8位图像
image8[i] = (unsigned char)(image16[i] >> 8);
}
}
int main() {
// 假设已经读取到16位图像数据并存储在image16数组中
unsigned short image16[WIDTH * HEIGHT];
// 声明8位图像数组
unsigned char image8[WIDTH * HEIGHT];
// 调用转换函数
convert16to8(image16, image8);
// 输出8位图像数据
for (int i = 0; i < WIDTH * HEIGHT; i++) {
printf("%d ", image8[i]);
}
return 0;
}
```
在上述示例代码中,首先定义了图像的宽度和高度,并实现了一个转换函数convert16to8。该函数接受一个16位图像数组和一个8位图像数组作为参数,在循环中将每个16位像素值的高8位赋值给8位图像数组。
在主函数中,声明和初始化16位图像数组image16和8位图像数组image8,然后调用转换函数convert16to8,将16位图像转换为8位图像。最后,通过循环打印8位图像数组的值,以验证转换结果。
请注意,这只是一个简单的示例代码,实际的应用可能涉及更复杂的图像处理操作。
### 回答3:
要将16位转换为8位图像 c,可以使用以下程序代码:
```c
#include <stdio.h>
// 函数:将16位图像转换为8位
void convert16to8(unsigned short* img16, unsigned char* img8, int width, int height)
{
int i;
for(i=0; i<width*height; i++)
{
unsigned short pixel16 = img16[i];
// 由于8位图像的灰度范围是0-255,将16位图像范围映射到0-255
unsigned char pixel8 = (unsigned char) (pixel16 * 255 / 65535);
img8[i] = pixel8;
}
}
int main()
{
int width = 100; // 图像宽度
int height = 100; // 图像高度
unsigned short* img16 = (unsigned short*) calloc(width * height, sizeof(unsigned short));
unsigned char* img8 = (unsigned char*) calloc(width * height, sizeof(unsigned char));
// 假设此处为读取16位图像的代码,将图像数据存储在img16数组中
// 转换16位图像为8位图像
convert16to8(img16, img8, width, height);
// 假设此处为保存8位图像的代码,将图像数据存储在img8数组中
free(img16);
free(img8);
return 0;
}
```
这个程序定义了一个`convert16to8`函数,用于将16位图像数据转换为8位图像数据。在`main`函数中,可以先读取16位图像数据,并将其存储在`img16`数组中。然后,通过调用`convert16to8`函数,将16位图像转换为8位图像,转换后的图像数据存储在`img8`数组中。最后,可以将8位图像数据保存或进行其他处理。在程序结束前,需要释放动态分配的内存空间。
阅读全文
相关推荐







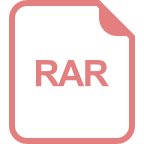

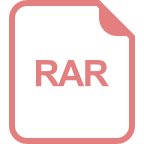


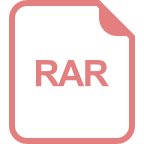


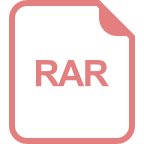

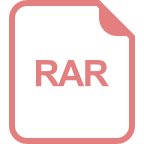