golong用gin实现html的登录
时间: 2024-02-17 19:04:02 浏览: 44
要使用Gin框架实现HTML登录页面,你可以按照以下步骤操作:
1. 安装Gin框架和HTML模板引擎
```go
go get -u github.com/gin-gonic/gin
go get -u github.com/gin-gonic/contrib/sessions
go get -u github.com/gin-gonic/gin-contrib/multitemplate
```
2. 创建Gin应用程序,并配置HTML模板引擎
```go
package main
import (
"github.com/gin-gonic/contrib/sessions"
"github.com/gin-gonic/gin"
"github.com/gin-gonic/gin-contrib/multitemplate"
)
func main() {
router := gin.Default()
//配置HTML模板引擎
router.HTMLRender = createMyRender()
//设置session存储
store := sessions.NewCookieStore([]byte("secret"))
router.Use(sessions.Sessions("mysession", store))
//定义路由
router.GET("/", indexHandler)
router.POST("/login", loginHandler)
//启动服务
router.Run(":8080")
}
//创建HTML模板引擎
func createMyRender() multitemplate.Renderer {
r := multitemplate.NewRenderer()
r.AddFromFiles("index", "templates/base.tmpl", "templates/index.tmpl")
return r
}
```
3. 创建HTML模板文件
在templates文件夹下创建两个文件:base.tmpl和index.tmpl。
base.tmpl:
```html
<!DOCTYPE html>
<html>
<head>
<title>{{.Title}}</title>
<meta charset="UTF-8">
</head>
<body>
{{template "content" .}}
</body>
</html>
```
index.tmpl:
```html
{{define "content"}}
<h1>登录页面</h1>
<form method="post" action="/login">
<label>用户名:</label>
<input type="text" name="username" /><br>
<label>密码:</label>
<input type="password" name="password" /><br>
<button type="submit">登录</button>
</form>
{{end}}
```
4. 创建路由处理函数
```go
//首页处理函数
func indexHandler(c *gin.Context) {
c.HTML(http.StatusOK, "index", gin.H{
"Title": "登录页面",
})
}
//登录处理函数
func loginHandler(c *gin.Context) {
username := c.PostForm("username")
password := c.PostForm("password")
//验证用户名和密码
if username == "admin" && password == "123456" {
//设置session
session := sessions.Default(c)
session.Set("username", username)
session.Save()
//跳转到首页
c.Redirect(http.StatusFound, "/home")
} else {
//用户名和密码错误,返回登录页面
c.HTML(http.StatusOK, "index", gin.H{
"Title": "登录页面",
"Error": "用户名或密码错误",
})
}
}
```
在loginHandler函数中,首先获取用户提交的用户名和密码,然后进行验证。如果验证通过,就设置session,并跳转到首页;如果验证失败,就返回登录页面,并显示错误提示。
5. 创建其他页面处理函数
```go
//首页处理函数
func homeHandler(c *gin.Context) {
//获取session
session := sessions.Default(c)
username := session.Get("username")
c.HTML(http.StatusOK, "home", gin.H{
"Title": "首页",
"Username": username,
})
}
```
在homeHandler函数中,首先获取session中保存的用户名,然后将其传递给HTML模板,显示在页面上。
6. 创建其他HTML模板文件
在templates文件夹下创建home.tmpl文件。
home.tmpl:
```html
{{define "content"}}
<h1>欢迎 {{.Username}} 登录</h1>
{{end}}
```
7. 完成登录页面
现在你可以启动应用程序,在浏览器中访问http://localhost:8080,就可以看到登录页面了。输入正确的用户名和密码,就可以跳转到首页了。
相关推荐
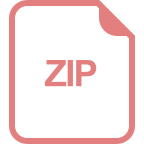
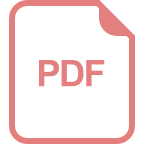
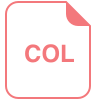
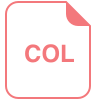













