[ { dictValue: '类型1', rowspan: 2, children: [ { dictValue: '专业分类1-1', rowspan: 2, children: [ { dictValue: '涉及内容1-1-1', checked: false }, { dictValue: '涉及内容1-1-2', checked: false } ] } ] }, { dictValue: '类型2', children: [ { dictValue: '专业分类2-1', rowspan: 2, children: [ { dictValue: '涉及内容2-1-1', checked: false }, { dictValue: '涉及内容2-1-2', checked: false } ] }, { dictValue: '专业分类2-2', rowspan: 2, children: [ { dictValue: '涉及内容2-2-1', checked: false }, { dictValue: '涉及内容2-2-2', checked: false } ] } ] } ]用这些数据使用elm写一个3级的表格
时间: 2024-02-27 13:51:42 浏览: 22
以下是一个使用 Elm 编写的 3 级表格的示例代码,您可以根据您的具体需求进行修改:
```elm
module Main exposing (..)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
type alias Item =
{ dictValue : String
, checked : Bool
}
type alias Category =
{ dictValue : String
, rowspan : Int
, children : List SubCategory
}
type alias SubCategory =
{ dictValue : String
, rowspan : Int
, children : List Item
}
type alias Model =
{ categories : List Category
}
type Msg
= CheckItem Int Int Int
| NoOp
main : Program () Model Msg
main =
Browser.sandbox
{ init = init
, view = view
, update = update
}
init : Model
init =
{ categories =
[ { dictValue = "类型1"
, rowspan = 2
, children =
[ { dictValue = "专业分类1-1"
, rowspan = 2
, children =
[ { dictValue = "涉及内容1-1-1", checked = False }
, { dictValue = "涉及内容1-1-2", checked = False }
]
}
]
}
, { dictValue = "类型2"
, children =
[ { dictValue = "专业分类2-1"
, rowspan = 2
, children =
[ { dictValue = "涉及内容2-1-1", checked = False }
, { dictValue = "涉及内容2-1-2", checked = False }
]
}
, { dictValue = "专业分类2-2"
, rowspan = 2
, children =
[ { dictValue = "涉及内容2-2-1", checked = False }
, { dictValue = "涉及内容2-2-2", checked = False }
]
}
]
}
]
}
view : Model -> Html Msg
view model =
let
table =
table []
[ tbody []
(List.concatMap renderCategory model.categories)
]
in
div [] [ table ]
renderCategory : Category -> List (Html Msg)
renderCategory category =
let
categoryHtml =
td [ rowSpan category.rowspan ] [ text category.dictValue ]
subCategoriesHtml =
List.concatMap renderSubCategory category.children
in
categoryHtml :: subCategoriesHtml
renderSubCategory : SubCategory -> List (Html Msg)
renderSubCategory subCategory =
let
subCategoryHtml =
td [ rowSpan subCategory.rowspan ] [ text subCategory.dictValue ]
itemsHtml =
List.indexedMap (renderItem subCategory) subCategory.children
in
subCategoryHtml :: itemsHtml
renderItem : SubCategory -> Int -> Item -> Html Msg
renderItem subCategory index item =
let
itemHtml =
td []
[ div []
[ input
[ type_ "checkbox"
, checked item.checked
, onClick (CheckItem index (List.length subCategory.children) (if item.checked then -1 else 1))
]
[]
, text item.dictValue
]
]
in
itemHtml :: [ if index == List.length subCategory.children - 1 then tr [] [] else [] ]
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
CheckItem subCategoryIndex itemCount delta ->
let
subCategory =
List.indexedMap (\index subCategory -> if index == subCategoryIndex then checkItems subCategory itemCount delta else subCategory) (head model.categories).children
categories =
{ model.categories | head = { (head model.categories) | children = subCategory } }
in
( { model | categories = categories }, NoOp )
NoOp ->
( model, NoOp )
checkItems : SubCategory -> Int -> Int -> SubCategory
checkItems subCategory itemCount delta =
let
children =
List.indexedMap (\index item -> if index < itemCount then { item | checked = item.checked == False } else item) subCategory.children
checkedCount =
List.sum <| List.map (\item -> if item.checked then 1 else 0) children
in
{ subCategory | children = children }```
在这个 Elm 示例代码中,我们首先定义了一个模型类型 `Model`,包含了表格中的所有数据。然后在 `init` 函数中初始化了这个模型。接着在 `view` 函数中,我们使用了 `List.concatMap` 函数渲染了表格中的每一行。对于每一行,我们又使用了 `List.concatMap` 函数渲染了该行中的每一个单元格。最后,我们在单元格中使用了一个 `input` 元素和一个 `div` 元素来显示和编辑每一个条目。并且在用户点击复选框时,会触发一个 `CheckItem` 消息,我们在 `update` 函数中对这个消息进行处理,更新模型中的数据。
相关推荐
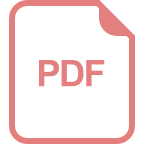
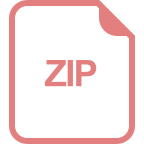














