pyqt中点击按钮开启或者关闭串口和线程
时间: 2023-09-02 21:09:49 浏览: 226
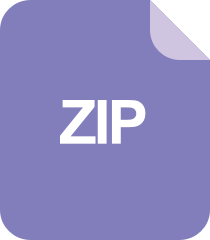
PyQT多线程串口工程文件 PyCharm

在PyQt中,可以使用QSerialPort类来控制串口的开启和关闭。同时,可以使用QThread类来控制线程的开启和关闭。
以下是一个简单的示例代码,可以通过点击按钮来开启或关闭串口和线程:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
from PyQt5.QtSerialPort import QSerialPort
from PyQt5.QtCore import QThread, pyqtSignal
class SerialThread(QThread):
# 自定义信号,用于在主线程中更新UI
received_data = pyqtSignal(str)
def __init__(self, serial_port):
super().__init__()
self.serial_port = serial_port
self.is_running = False
def run(self):
self.is_running = True
while self.is_running:
if self.serial_port.isOpen():
# 读取串口数据
data = self.serial_port.readLine()
# 发送自定义信号
self.received_data.emit(data.decode())
def stop(self):
self.is_running = False
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建串口对象
self.serial_port = QSerialPort(self)
self.serial_port.setBaudRate(9600)
# 创建线程对象
self.serial_thread = SerialThread(self.serial_port)
self.serial_thread.received_data.connect(self.update_ui)
# 创建按钮对象
self.btn_open = QPushButton('Open', self)
self.btn_open.clicked.connect(self.open_serial)
self.btn_close = QPushButton('Close', self)
self.btn_close.clicked.connect(self.close_serial)
# 添加按钮到主窗口
self.setCentralWidget(self.btn_open)
self.addToolBar('Serial').addWidget(self.btn_close)
def open_serial(self):
# 打开串口
self.serial_port.setPortName('/dev/ttyACM0')
if self.serial_port.open(QSerialPort.ReadWrite):
# 启动线程
self.serial_thread.start()
# 切换按钮
self.setCentralWidget(self.btn_close)
def close_serial(self):
# 停止线程
self.serial_thread.stop()
self.serial_thread.wait()
# 关闭串口
self.serial_port.close()
# 切换按钮
self.setCentralWidget(self.btn_open)
def update_ui(self, data):
# 在主线程中更新UI
print(data.strip())
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的示例中,SerialThread类继承自QThread类,重写了run()方法,用于在新线程中读取串口数据并发送自定义信号。MainWindow类继承自QMainWindow类,创建了串口对象和线程对象,并通过按钮的clicked信号来启动和停止串口和线程。同时,MainWindow类还实现了一个update_ui()方法,用于在主线程中更新UI。
阅读全文
相关推荐
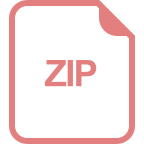
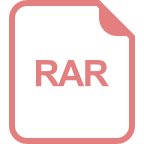



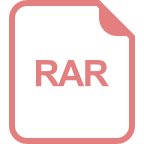


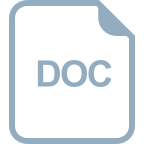
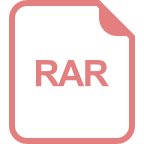

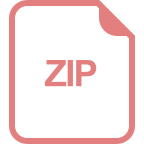
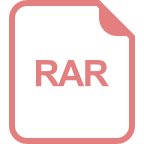

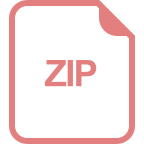
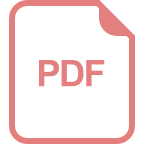
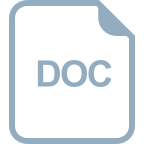
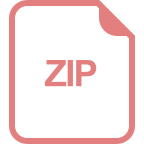