yolov5调用摄像头
时间: 2023-09-09 17:12:15 浏览: 120
要使用 YOLOv5 调用摄像头,你需要:
1. 安装 PyTorch 和 YOLOv5
2. 连接摄像头
在安装好 PyTorch 和 YOLOv5 之后,你可以尝试以下代码:
```python
import cv2
import torch
from yolov5.models.experimental import attempt_load
from yolov5.utils.general import non_max_suppression, scale_coords
from yolov5.utils.torch_utils import select_device, time_sync
def detect():
# 设置使用的设备,可以是 "cpu" 或 "cuda:0" 等等
device = select_device('')
# 加载模型
model = attempt_load('yolov5s.pt', map_location=device)
# 开启摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取一帧图像
ret, frame = cap.read()
# 将图像转换为 Torch 张量并归一化
img = torch.from_numpy(frame).to(device)
img = img.float() / 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 检测物体
pred = model(img)[0]
pred = non_max_suppression(pred, 0.4, 0.5)
# 绘制检测结果
for det in pred:
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], frame.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{model.names[int(cls)]} {conf:.2f}'
plot_one_box(xyxy, frame, label=label, color=colors(int(cls)), line_thickness=3)
# 显示图像
cv2.imshow('frame', frame)
# 按下 q 键退出
if cv2.waitKey(1) == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
def plot_one_box(xyxy, img, color=None, label=None, line_thickness=None):
# 绘制一个框
tl = line_thickness or round(0.002 * max(img.shape[0:2])) + 1 # line thickness
color = color or [random.randint(0, 255) for _ in range(3)]
c1, c2 = (int(xyxy[0]), int(xyxy[1])), (int(xyxy[2]), int(xyxy[3]))
cv2.rectangle(img, c1, c2, color, thickness=tl, lineType=cv2.LINE_AA)
if label:
tf = max(tl - 1, 1) # font thickness
t_size = cv2.getTextSize(label, 0, fontScale=tl / 3, thickness=tf)[0]
c2 = c1[0] + t_size[0], c1[1] - t_size[1] - 3
cv2.rectangle(img, c1, c2, color, -1, cv2.LINE_AA) # filled
cv2.putText(img, label, (c1[0], c1[1] - 2), 0, tl / 3, [225, 255, 255], thickness=tf, lineType=cv2.LINE_AA)
detect()
```
此代码将使用 YOLOv5 模型从摄像头中检测物体,并在图像上绘制检测结果。你需要设置 `device` 变量以指定使用的设备,例如 `cuda:0` 表示使用第一个 GPU。摄像头的 ID 默认为 0,如果你有多个摄像头,可以指定不同的 ID。
阅读全文
相关推荐
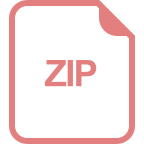
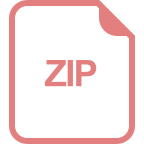













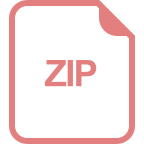