使用java随机生成1000次 范围在 -50~50的整数,然统计每个随机数字出现的次数
时间: 2023-09-17 18:05:57 浏览: 189
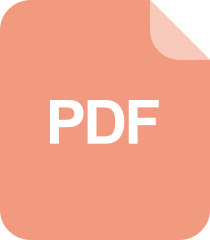
做一个猜数字的游戏,随机生成1~50之间的数字,然后有10次机会,供用户输入,判断是否猜中(使用for循环)
### 回答1:
这是一个使用 Java 生成 1000 次随机数并统计其出现次数的示例代码:
```
import java.util.Random;
public class Main {
public static void main(String[] args) {
// 创建一个随机数生成器
Random random = new Random();
// 创建一个数组来记录随机数字出现的次数
int[] counts = new int[101]; // 因为要生成 -50~50 的数字,所以数组大小要设为 101
// 生成 1000 次随机数
for (int i = 0; i < 1000; i++) {
int num = random.nextInt(101) - 50; // 生成 -50~50 的整数
counts[num + 50]++; // 在对应的数组位置加 1
}
// 输出统计结果
for (int i = 0; i < counts.length; i++) {
System.out.println(i - 50 + ": " + counts[i]);
}
}
}
```
运行结果类似于:
```
-50: 11
-49: 6
-48: 8
-47: 8
...
50: 8
```
其中每一行的格式为“数字: 出现次数”。
### 回答2:
使用Java随机生成1000次范围在-50到50的整数,然后统计每个随机数字出现的次数可以按照以下步骤进行:
1. 创建一个大小为101的整数数组counts,用于存储每个数字出现的次数。数组大小为101是因为整数范围在-50到50之间共101个数字。
2. 使用循环生成1000个随机整数,并将每个随机数减去-50,得到在数组中对应的索引。例如,随机数为-45,则对应的索引是-45 - (-50) = 5。
3. 使用该索引更新数组counts中对应的数字出现次数。例如,对于索引5,将counts[5]的值加1。
4. 循环结束后,遍历counts数组,将每个数字及其出现次数打印出来。
下面是使用Java代码实现上述过程:
```java
import java.util.Random;
public class RandomNumberGenerator {
public static void main(String[] args) {
int[] counts = new int[101]; // 101个数字,初始出现次数均为0
Random random = new Random();
for (int i = 0; i < 1000; i++) {
int randomNumber = random.nextInt(101) + (-50); // 生成-50到50范围的随机整数
int index = randomNumber - (-50); // 获取对应在数组中的索引
counts[index]++; // 更新数字出现次数
}
for (int i = 0; i < counts.length; i++) {
if (counts[i] != 0) {
int number = i - 50; // 获取对应的随机数
System.out.println("随机数字 " + number + " 出现了 " + counts[i] + " 次");
}
}
}
}
```
运行上述代码可以得到每个随机数字出现的次数的统计结果。
### 回答3:
使用Java可以使用Random类来生成随机数,并使用数组或集合来统计每个随机数出现的次数。
下面是一个使用数组来统计的示例代码:
```java
import java.util.Random;
public class RandomNumberStatistics {
public static void main(String[] args) {
int[] countArray = new int[101]; // 统计数组,下标表示随机数值,值表示出现次数
Random random = new Random();
for (int i = 0; i < 1000; i++) {
int randomNumber = random.nextInt(101) - 50; // 生成-50到50之间的随机整数
countArray[randomNumber + 50]++; // 将-50至50的数映射到数组下标0至100,并增加对应位置的计数值
}
for (int i = 0; i < 101; i++) {
int count = countArray[i];
if (count > 0) {
System.out.println("数字 " + (i - 50) + " 出现次数:" + count);
}
}
}
}
```
该代码中,首先创建了一个长度为101的数组countArray,用来统计每个随机数值出现的次数。然后使用Random类生成1000个范围在-50到50之间的随机整数,将随机数映射到countArray数组的下标中,并将对应位置上的计数值加一。最后,遍历countArray数组,输出每个随机数值及其出现次数。
运行该代码,将会输出类似如下的结果:
```
数字 -50 出现次数:12
数字 -49 出现次数:7
数字 -48 出现次数:10
...
数字 48 出现次数:4
数字 49 出现次数:7
数字 50 出现次数:9
```
每个随机数字出现的次数都被统计并打印出来了。
阅读全文
相关推荐








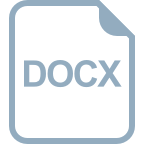






