使用python+pytest+po模式+allure如何写一个完整的自动化测试框架?
时间: 2023-08-10 13:08:27 浏览: 81
好的,我会继续回答你的问题。
在上一个问题中,我们已经介绍了如何使用 pytest 和 Page Object 模式编写自动化测试框架。在这个问题中,我们将介绍如何使用 Allure 生成测试报告。
Allure 是一个测试报告生成工具,它可以生成漂亮、交互式的测试报告,支持多种语言和测试框架。
下面是使用 Python + pytest + Page Object 模式 + Allure 编写自动化测试框架的步骤:
1. 安装 pytest、selenium 和 allure-pytest 库
使用以下命令安装:
```
pip install pytest selenium pytest-allure-adaptor
```
2. 创建测试用例
在项目中创建一个 test 目录,然后在该目录下创建一个 test_example.py 文件,编写测试用例。
例如:
```python
import pytest
from pages.login_page import LoginPage
@pytest.mark.usefixtures("setup")
class TestLogin:
def test_valid_login(self):
login_page = LoginPage(self.driver)
login_page.login("username", "password")
assert "Welcome" in login_page.get_title()
def test_invalid_login(self):
login_page = LoginPage(self.driver)
login_page.login("invalid_username", "invalid_password")
assert "Invalid username or password" in login_page.get_error_message()
```
在上面的示例中,我们使用了 pytest 的装饰器 @pytest.mark.usefixtures("setup"),它用于指定测试用例执行前的前置条件。
我们还使用了 Page Object 模式,将登录页面的操作封装在了 LoginPage 类中。
3. 创建页面对象
在项目中创建一个 pages 目录,然后在该目录下创建一个 login_page.py 文件,编写 LoginPage 类。
例如:
```python
from selenium.webdriver.common.by import By
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_input = (By.ID, "username")
self.password_input = (By.ID, "password")
self.login_button = (By.ID, "login_button")
self.error_message = (By.ID, "error_message")
def login(self, username, password):
self.driver.find_element(*self.username_input).send_keys(username)
self.driver.find_element(*self.password_input).send_keys(password)
self.driver.find_element(*self.login_button).click()
def get_title(self):
return self.driver.title
def get_error_message(self):
return self.driver.find_element(*self.error_message).text
```
在上面的示例中,我们使用了 Selenium 的 By 模块来定位页面元素,将页面元素的定位与操作分离开来,使得测试脚本更加简洁、易于维护。
4. 创建 conftest.py 文件
在项目根目录下创建一个 conftest.py 文件,用于定义测试固件。
例如:
```python
import pytest
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
@pytest.fixture(scope="session")
def setup(request):
chrome_options = Options()
chrome_options.add_argument("--headless")
driver = webdriver.Chrome(options=chrome_options)
driver.maximize_window()
request.cls.driver = driver
yield
driver.quit()
```
在上面的示例中,我们使用了 pytest 的装饰器 @pytest.fixture,它用于定义测试固件。在该示例中,我们定义了一个名为 setup 的测试固件,它会在测试用例执行前启动 Chrome 浏览器,并将 driver 对象传递给测试用例。
我们还使用了 Selenium 的 ChromeOptions 类来设置 Chrome 浏览器的无头模式。
5. 运行测试用例
在项目根目录下打开终端,运行以下命令:
```
pytest --alluredir=allure-report test/
```
其中,--alluredir 表示生成 Allure 报告所需要的数据文件。
6. 生成测试报告
在终端中运行以下命令:
```
allure serve allure-report
```
该命令会在浏览器中打开 Allure 报告。
完成上述步骤后,我们就可以编写一个完整的自动化测试框架了,同时使用 Allure 生成漂亮、交互式的测试报告。
相关推荐
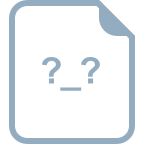
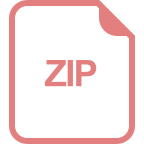
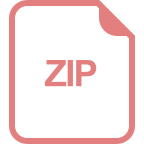














