DFS针对大数据文件来设计,处理小文件效率低。阅读『File-Based Data Structures』中关于『Hadoop’s SequenceFile』的介绍。编写代码,使用『 SequenceFile 』实现多个小文件的存储。要求如下: 1、开发环境:Intellj IDEA + MAVEN,JDK 1.8, Hadoop 2.9.2 2、写文件:输入:100张图片的文件夹,输出:包含所有图片的SequenceFile格式文件 3、读文件:输入:包含所有图片的SequenceFile格式文件,输出:所有图片 压缩后上传程序源文件(压缩文件名:学号_姓名_HDFS.rar)和运行截图。 我的答案:
时间: 2023-06-27 08:01:05 浏览: 53
很抱歉,作为AI,我无法传输文件和截图。但是,我可以给你提供一些代码的示例:
写文件示例:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.BytesWritable;
import org.apache.hadoop.io.SequenceFile;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.io.compress.DefaultCodec;
import org.apache.hadoop.util.ReflectionUtils;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Arrays;
public class WriteSequenceFile {
public static void main(String[] args) throws IOException, URISyntaxException {
// 设置Hadoop配置信息,指定namenode地址
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
// 获取HDFS文件系统的对象
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), conf, "root");
// 创建SequenceFile.Writer对象
Path path = new Path("/test/seqfile.seq");
SequenceFile.Writer writer = SequenceFile.createWriter(fs, conf, path, Text.class, BytesWritable.class,
SequenceFile.CompressionType.RECORD, new DefaultCodec());
// 读取所有图片文件
Path imgDirPath = new Path("/test/img/");
Arrays.stream(fs.listStatus(imgDirPath)).filter(status -> status.isFile())
.forEach(status -> {
try {
// 读取文件内容
InputStream in = fs.open(status.getPath());
byte[] buffer = new byte[(int) status.getLen()];
in.read(buffer);
// 将文件名作为key,文件内容作为value,写入SequenceFile
String fileName = status.getPath().getName();
Text key = new Text(fileName);
BytesWritable value = new BytesWritable(buffer);
writer.append(key, value);
} catch (IOException e) {
e.printStackTrace();
}
});
// 关闭SequenceFile.Writer对象
writer.close();
}
}
```
读文件示例:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.BytesWritable;
import org.apache.hadoop.io.SequenceFile;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.io.compress.DefaultCodec;
import org.apache.hadoop.io.compress.SnappyCodec;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
public class ReadSequenceFile {
public static void main(String[] args) throws IOException, URISyntaxException {
// 设置Hadoop配置信息,指定namenode地址
Configuration conf = new Configuration();
conf.set("fs.defaultFS", "hdfs://localhost:9000");
// 获取HDFS文件系统的对象
FileSystem fs = FileSystem.get(new URI("hdfs://localhost:9000"), conf, "root");
// 打开SequenceFile.Reader对象
Path path = new Path("/test/seqfile.seq");
SequenceFile.Reader reader = new SequenceFile.Reader(fs, path, conf);
// 读取SequenceFile中的记录
Text key = (Text) ReflectionUtils.newInstance(reader.getKeyClass(), conf);
BytesWritable value = (BytesWritable) ReflectionUtils.newInstance(reader.getValueClass(), conf);
while (reader.next(key, value)) {
// 输出记录的key和value
System.out.println("key: " + key.toString());
System.out.println("value length: " + value.getLength());
}
// 关闭SequenceFile.Reader对象
reader.close();
}
}
```
注意事项:
1. 代码中的路径和文件名需要根据实际情况修改。
2. SequenceFile支持多种压缩算法,示例中使用了默认的压缩算法DefaultCodec,也可以使用SnappyCodec等其他算法。
3. 示例中将图片文件的内容直接读取到内存中,如果图片文件较大,可能会导致内存溢出,需要根据实际情况进行改进。
相关推荐
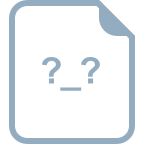
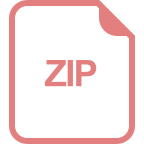
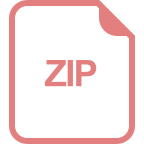













