用python写一段模拟砍价的代码
时间: 2023-06-02 19:05:35 浏览: 266
好的,以下是一段简单的模拟砍价的 Python 代码:
```python
import random
price = 100 # 商品售价
while True:
offer = int(input("请输入您的出价:"))
if offer > price:
print("出价高于商品售价,请重新出价!")
elif offer == price:
print("恭喜您!砍价成功,您成功购买此商品!")
break
else:
print("出价低于商品售价!")
if random.random() > 0.5:
price = price - random.randint(1, 10)
print("商家已接受您的出价,但是只能给您减少 %d 元,商品价格为 %d 元。" % (price - offer, price))
else:
print("非常抱歉,商家拒绝了您的出价!")
```
这段代码模拟了用户输入出价,商家接受或拒绝出价的过程。如果商家接受出价,则随机给出一个减价的幅度,商品的售价会相应减少;否则,用户需要重新出价。
相关问题
帮我用用python写一段模拟iic的代码
以下是使用Python模拟I2C通信的示例代码:
```python
import time
# 模拟I2C设备
class I2CDevice:
def __init__(self, address):
self.address = address
# 发送数据
def send(self, data):
print("Send data:", data)
# 接收数据
def receive(self, count):
print("Receive data:", count)
# 模拟I2C总线
class I2CBus:
def __init__(self):
self.devices = []
# 添加设备
def add_device(self, device):
self.devices.append(device)
# 发送数据
def send(self, address, data):
for device in self.devices:
if device.address == address:
device.send(data)
return
# 接收数据
def receive(self, address, count):
for device in self.devices:
if device.address == address:
device.receive(count)
return
# 创建I2C设备和总线
device = I2CDevice(0x27)
bus = I2CBus()
bus.add_device(device)
# 发送数据
bus.send(0x27, [0x01, 0x02, 0x03])
# 接收数据
bus.receive(0x27, 4)
```
以上代码模拟了一个I2C设备和总线,可以发送和接收数据。在实际应用中,需要根据具体的I2C设备和应用场景进行修改。
使用python写一段验证码识别代码
好的,这里给您提供一份基于Python的验证码识别代码,使用了PIL库进行图像处理和识别:
```python
from PIL import Image
import pytesseract
# 加载图片
image = Image.open('captcha.png')
# 转化为灰度图像
gray_image = image.convert('L')
# 去除噪声
filtered_image = gray_image.point(lambda p: p > 170 and 255)
# 识别验证码
captcha_text = pytesseract.image_to_string(filtered_image, lang='eng', config='--psm 10')
print('验证码识别结果为:', captcha_text)
```
这段代码首先使用PIL库加载验证码图片,然后将其转化为灰度图像,再使用图像处理技术去除噪声,最后使用pytesseract库进行验证码识别,输出识别结果。注意:这段代码仅适用于简单的数字或字母验证码,并不能保证100%的识别准确率。
阅读全文
相关推荐
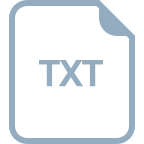
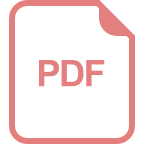
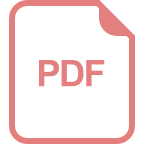













