深度强化学习代码gpt
时间: 2023-09-30 17:12:37 浏览: 154
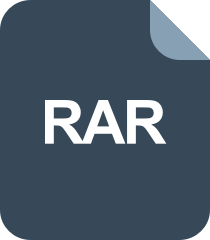
深度学习代码
以下是使用深度强化学习实现GPT的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# 定义GPT模型
class GPT(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim, num_layers):
super(GPT, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.lstm = nn.LSTM(embedding_dim, hidden_dim, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_dim, vocab_size)
def forward(self, x, h=None):
x = self.embedding(x)
out, h = self.lstm(x, h)
out = self.fc(out)
return out, h
# 定义强化学习训练函数
def train(model, data, optimizer, criterion, num_epochs=10):
for epoch in range(num_epochs):
total_loss = 0
h = None
for batch in data:
x, y = batch
optimizer.zero_grad()
out, h = model(x, h)
loss = criterion(out[:, :-1, :].reshape(-1, out.shape[2]), y[:, 1:].reshape(-1))
loss.backward()
optimizer.step()
total_loss += loss.item()
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, total_loss/len(data)))
# 设置超参数
vocab_size = 10000
embedding_dim = 32
hidden_dim = 64
num_layers = 2
num_epochs = 10
batch_size = 32
lr = 0.01
# 生成训练数据
data = []
for i in range(1000):
seq_len = np.random.randint(5, 20)
seq = np.random.randint(0, vocab_size, seq_len)
x = seq[:-1].reshape(1, -1)
y = seq[1:].reshape(1, -1)
data.append((torch.LongTensor(x), torch.LongTensor(y)))
# 初始化模型、优化器、损失函数
model = GPT(vocab_size, embedding_dim, hidden_dim, num_layers)
optimizer = optim.Adam(model.parameters(), lr=lr)
criterion = nn.CrossEntropyLoss()
# 训练模型
train(model, data, optimizer, criterion, num_epochs=num_epochs)
```
上述代码中,我们定义了一个GPT模型,使用LSTM作为基础模型,然后使用强化学习方法进行训练。在训练函数中,我们循环遍历数据集中的每一个batch,然后使用模型对输入进行预测,并计算预测结果与真实标签之间的交叉熵损失。最后使用反向传播更新模型参数。
阅读全文
相关推荐
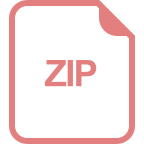
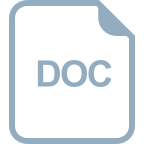
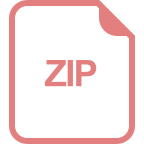
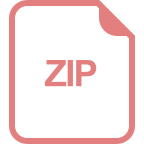
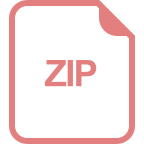
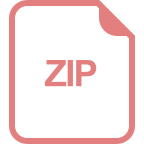
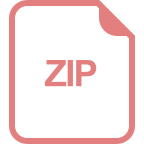
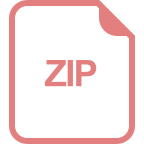
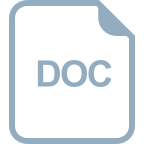
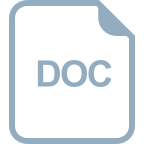
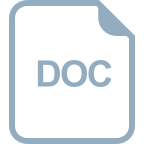
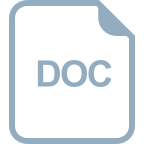
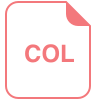
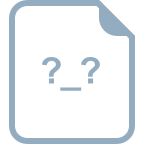