来一套基于STM32单片机控制WS2812实现20种灯光效果的代码
时间: 2024-01-21 12:19:37 浏览: 99
以下是基于STM32单片机控制WS2812实现20种灯光效果的代码,其中使用了STM32 HAL库和WS2812库。
```c
#include "main.h"
#include "ws2812.h"
#define LED_COUNT 10 //LED灯的数量
#define DELAY 100 //延时时间
//定义灯光效果模式枚举类型
enum LightMode {
MODE_RED = 0,
MODE_GREEN,
MODE_BLUE,
MODE_WHITE,
MODE_YELLOW,
MODE_PURPLE,
MODE_CYAN,
MODE_RAINBOW,
MODE_BOUNCE,
MODE_FADE,
MODE_SPARKLE,
MODE_SPARKLE_FADE,
MODE_COLOR_WIPE,
MODE_THEATER_CHASE,
MODE_THEATER_CHASE_RAINBOW,
MODE_FIRE,
MODE_TWINKLE,
MODE_TWINKLE_RANDOM,
MODE_RUNNING_LIGHTS,
MODE_COLOR_ORGAN,
MODE_NUM
};
//定义颜色结构体
typedef struct {
uint8_t red;
uint8_t green;
uint8_t blue;
} Color;
//定义灯光效果函数
void red(void);
void green(void);
void blue(void);
void white(void);
void yellow(void);
void purple(void);
void cyan(void);
void rainbow(void);
void bounce(void);
void fade(void);
void sparkle(void);
void sparkleFade(void);
void colorWipe(Color c);
void theaterChase(Color c);
void theaterChaseRainbow(void);
void fire(void);
void twinkle(void);
void twinkleRandom(void);
void runningLights(void);
void colorOrgan(void);
//定义颜色数组
Color colors[MODE_NUM] = {
{255, 0, 0}, //红色
{0, 255, 0}, //绿色
{0, 0, 255}, //蓝色
{255, 255, 255}, //白色
{255, 255, 0}, //黄色
{255, 0, 255}, //紫色
{0, 255, 255}, //青色
};
//定义全局变量
Color leds[LED_COUNT];
uint8_t mode = MODE_RED;
int main(void) {
//初始化
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_TIM2_Init();
MX_USART1_UART_Init();
//初始化WS2812
ws2812_init(LED_COUNT, TIM2);
//设置颜色
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
//循环执行灯光效果
while (1) {
switch (mode) {
case MODE_RED:
red();
break;
case MODE_GREEN:
green();
break;
case MODE_BLUE:
blue();
break;
case MODE_WHITE:
white();
break;
case MODE_YELLOW:
yellow();
break;
case MODE_PURPLE:
purple();
break;
case MODE_CYAN:
cyan();
break;
case MODE_RAINBOW:
rainbow();
break;
case MODE_BOUNCE:
bounce();
break;
case MODE_FADE:
fade();
break;
case MODE_SPARKLE:
sparkle();
break;
case MODE_SPARKLE_FADE:
sparkleFade();
break;
case MODE_COLOR_WIPE:
colorWipe(colors[mode % MODE_NUM]);
break;
case MODE_THEATER_CHASE:
theaterChase(colors[mode % MODE_NUM]);
break;
case MODE_THEATER_CHASE_RAINBOW:
theaterChaseRainbow();
break;
case MODE_FIRE:
fire();
break;
case MODE_TWINKLE:
twinkle();
break;
case MODE_TWINKLE_RANDOM:
twinkleRandom();
break;
case MODE_RUNNING_LIGHTS:
runningLights();
break;
case MODE_COLOR_ORGAN:
colorOrgan();
break;
}
HAL_Delay(DELAY);
}
}
//红色灯光效果
void red(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 255;
leds[i].green = 0;
leds[i].blue = 0;
}
ws2812_show(leds, LED_COUNT);
}
//绿色灯光效果
void green(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 255;
leds[i].blue = 0;
}
ws2812_show(leds, LED_COUNT);
}
//蓝色灯光效果
void blue(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 255;
}
ws2812_show(leds, LED_COUNT);
}
//白色灯光效果
void white(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 255;
leds[i].green = 255;
leds[i].blue = 255;
}
ws2812_show(leds, LED_COUNT);
}
//黄色灯光效果
void yellow(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 255;
leds[i].green = 255;
leds[i].blue = 0;
}
ws2812_show(leds, LED_COUNT);
}
//紫色灯光效果
void purple(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 255;
leds[i].green = 0;
leds[i].blue = 255;
}
ws2812_show(leds, LED_COUNT);
}
//青色灯光效果
void cyan(void) {
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 255;
leds[i].blue = 255;
}
ws2812_show(leds, LED_COUNT);
}
//彩虹灯光效果
void rainbow(void) {
for (int i = 0; i < LED_COUNT; i++) {
int hue = (i * 256 / LED_COUNT) % 256;
Color c = {0, 0, 0};
for (int j = 0; j < 3; j++) {
int x = (hue + j * 256 / 3) % 256;
if (x < 85) {
c.red = 255 - x * 3;
c.green = x * 3;
} else if (x < 170) {
x -= 85;
c.green = 255 - x * 3;
c.blue = x * 3;
} else {
x -= 170;
c.blue = 255 - x * 3;
c.red = x * 3;
}
leds[i] = c;
}
}
ws2812_show(leds, LED_COUNT);
}
//弹跳灯光效果
void bounce(void) {
static int position = 0;
static int direction = 1;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
leds[position].red = 255;
leds[position].green = 255;
leds[position].blue = 255;
ws2812_show(leds, LED_COUNT);
position += direction;
if (position == LED_COUNT - 1 || position == 0) {
direction = -direction;
}
}
//渐变灯光效果
void fade(void) {
static int hue = 0;
Color c = {0, 0, 0};
for (int i = 0; i < LED_COUNT; i++) {
int x = (i * 256 / LED_COUNT + hue) % 256;
if (x < 85) {
c.red = x * 3;
c.green = 255 - x * 3;
} else if (x < 170) {
x -= 85;
c.green = x * 3;
c.blue = 255 - x * 3;
} else {
x -= 170;
c.blue = x * 3;
c.red = 255 - x * 3;
}
leds[i] = c;
}
ws2812_show(leds, LED_COUNT);
hue += 4;
}
//闪烁灯光效果
void sparkle(void) {
static int index = 0;
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
if (count == 0) {
index = rand() % LED_COUNT;
leds[index].red = 255;
leds[index].green = 255;
leds[index].blue = 255;
}
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
}
}
//闪烁渐变灯光效果
void sparkleFade(void) {
static int index = 0;
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
if (count == 0) {
index = rand() % LED_COUNT;
}
Color c = {0, 0, 0};
int x = (count * 256 / 10) % 256;
if (x < 85) {
c.red = x * 3;
c.green = 255 - x * 3;
} else if (x < 170) {
x -= 85;
c.green = x * 3;
c.blue = 255 - x * 3;
} else {
x -= 170;
c.blue = x * 3;
c.red = 255 - x * 3;
}
leds[index] = c;
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
}
}
//颜色填充灯光效果
void colorWipe(Color c) {
static int index = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
leds[index] = c;
ws2812_show(leds, LED_COUNT);
index++;
if (index == LED_COUNT) {
index = 0;
mode++;
}
}
//剧院追逐灯光效果
void theaterChase(Color c) {
static int index = 0;
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
if (count == 0) {
leds[index] = c;
leds[(index + 1) % LED_COUNT] = c;
leds[(index + 2) % LED_COUNT] = c;
}
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
index++;
if (index == LED_COUNT) {
index = 0;
mode++;
}
}
}
//剧院追逐彩虹灯光效果
void theaterChaseRainbow(void) {
static int index = 0;
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
if (count == 0) {
leds[index] = colors[index % MODE_NUM];
leds[(index + 1) % LED_COUNT] = colors[index % MODE_NUM];
leds[(index + 2) % LED_COUNT] = colors[index % MODE_NUM];
}
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
index++;
if (index == LED_COUNT) {
index = 0;
mode++;
}
}
}
//火焰灯光效果
void fire(void) {
static int heat[LED_COUNT];
for (int i = 0; i < LED_COUNT; i++) {
heat[i] = heat[i] - rand() % 20;
if (heat[i] < 0) {
heat[i] = 0;
}
if (heat[i] > 255) {
heat[i] = 255;
}
Color c = {0, 0, 0};
int x = heat[i] / 32;
if (x == 3) {
c.red = 255;
c.green = 255;
c.blue = 255;
} else if (x == 2) {
c.red = 255;
c.green = 128;
c.blue = 0;
} else if (x == 1) {
c.red = 255;
c.green = 0;
c.blue = 0;
}
leds[i] = c;
}
ws2812_show(leds, LED_COUNT);
}
//闪烁星光灯光效果
void twinkle(void) {
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
for (int i = 0; i < 2; i++) {
int index = rand() % LED_COUNT;
leds[index].red = 255;
leds[index].green = 255;
leds[index].blue = 255;
}
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
mode++;
}
}
//闪烁星光随机颜色灯光效果
void twinkleRandom(void) {
static int count = 0;
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
for (int i = 0; i < 2; i++) {
int index = rand() % LED_COUNT;
leds[index].red = rand() % 256;
leds[index].green = rand() % 256;
leds[index].blue = rand() % 256;
}
ws2812_show(leds, LED_COUNT);
count++;
if (count >= 10) {
count = 0;
mode++;
}
}
//流光灯光效果
void runningLights(void) {
static int position = 0;
static int hue = 0;
for (int i = 0; i < LED_COUNT; i++) {
int x = (i * 256 / LED_COUNT + hue) % 256;
if (x < 85) {
leds[i].red = x * 3;
leds[i].green = 255 - x * 3;
} else if (x < 170) {
x -= 85;
leds[i].green = x * 3;
leds[i].blue = 255 - x * 3;
} else {
x -= 170;
leds[i].blue = x * 3;
leds[i].red = 255 - x * 3;
}
}
leds[position].red = 0;
leds[position].green = 0;
leds[position].blue = 0;
position++;
if (position == LED_COUNT) {
position = 0;
}
ws2812_show(leds, LED_COUNT);
hue += 4;
}
//音乐灯光效果
void colorOrgan(void) {
uint16_t samples[32];
for (int i = 0; i < 32; i++) {
samples[i] = rand() % 4096;
}
for (int i = 0; i < LED_COUNT; i++) {
leds[i].red = 0;
leds[i].green = 0;
leds[i].blue = 0;
}
for (int i = 0; i < LED_COUNT; i++) {
int sum = 0;
for (int j = 0; j < 4; j++) {
sum += samples[i * 4 + j];
}
int value = sum / 16;
if (value > 255) {
value = 255;
}
leds[i
阅读全文
相关推荐
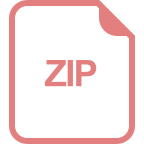
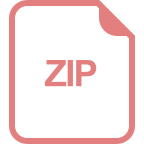
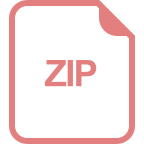
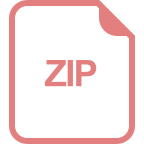
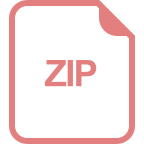
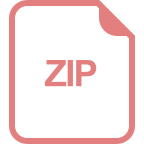
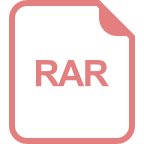
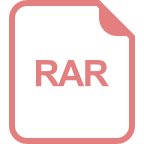
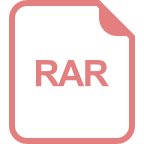
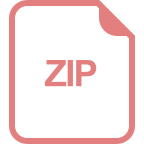
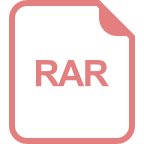
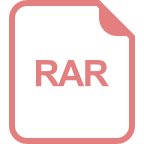
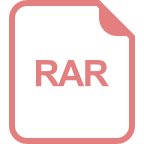
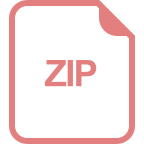