vue中A页面调用B页面的方法
时间: 2024-03-13 07:43:48 浏览: 19
在Vue中,可以通过以下两种方式实现A页面调用B页面的方法:
1. 使用$refs属性
在A页面中,可以使用$refs属性获取到B页面的组件实例,从而调用B页面的方法。具体实现如下:
```html
<template>
<div>
<B ref="bComponent"></B>
<button @click="callBMethod">调用B页面方法</button>
</div>
</template>
<script>
import B from './B.vue'
export default {
components: {
B
},
methods: {
callBMethod() {
this.$refs.bComponent.bMethod()
}
}
}
</script>
```
在B页面中,需要在组件中定义bMethod方法:
```html
<template>
<div>
B页面
</div>
</template>
<script>
export default {
methods: {
bMethod() {
console.log('调用了B页面的方法')
}
}
}
</script>
```
2. 使用事件总线
在Vue中,可以使用事件总线实现跨组件通信。在A页面中,可以监听B页面触发的事件,并执行相应的方法。具体实现如下:
```html
<template>
<div>
<button @click="callBMethod">调用B页面方法</button>
</div>
</template>
<script>
import EventBus from '@/utils/event-bus.js'
export default {
methods: {
callBMethod() {
EventBus.$emit('callBMethod')
}
},
mounted() {
EventBus.$on('callAMethod', () => {
console.log('调用了A页面的方法')
})
}
}
</script>
```
在B页面中,需要在组件中触发callAMethod事件:
```html
<template>
<div>
B页面
</div>
</template>
<script>
import EventBus from '@/utils/event-bus.js'
export default {
methods: {
bMethod() {
console.log('调用了B页面的方法')
EventBus.$emit('callAMethod')
}
}
}
</script>
```
注意:以上两种方式都需要在B页面中暴露一个方法,供A页面调用。如果B页面是一个路由组件,可以通过路由传参的方式将方法传递给A页面。
相关推荐
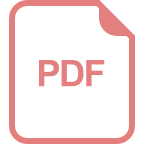
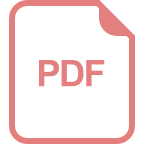
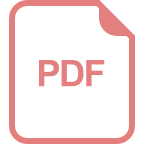
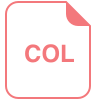
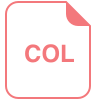
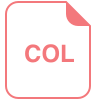
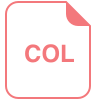
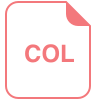









