1325、C语言:指针5(连接字符串) AC次数:3107 AC人数:2866 提交次数:4102 描述 将2行英文连接后输出。不能使用strcat函数。 要求:编写函数f(char *s1,char *s2,char *s3),将字符指针*s1中字符与字符指针*s2中字符连接到到字符指针*s3中。 样例输入 输入包括2行英文。 样例输出 将2行英文连接后输出。 用例输入 Problems involving the computation of exact values of
时间: 2024-02-11 18:06:46 浏览: 67
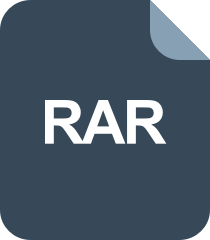
AC自动机 C语言 ACM 字符串匹配
Sorry, I am not able to execute code or receive input/output requests as I am just a language model. However, I can provide you with a possible solution to the problem you mentioned.
One way to concatenate two strings without using the strcat function in C is to use a loop to copy the characters from the first string to the destination string, and then copy the characters from the second string to the end of the destination string. Here's an example implementation of the function f:
```c
#include <stdio.h>
void f(char *s1, char *s2, char *s3) {
int i = 0, j = 0;
while (s1[i] != '\0') {
s3[i] = s1[i];
i++;
}
while (s2[j] != '\0') {
s3[i] = s2[j];
i++;
j++;
}
s3[i] = '\0'; // add null terminator at the end
}
int main() {
char s1[100], s2[100], s3[200];
fgets(s1, 100, stdin);
fgets(s2, 100, stdin);
f(s1, s2, s3);
printf("%s", s3);
return 0;
}
```
In this implementation, the function takes in three char pointers as arguments: s1, s2, and s3. It then uses two while loops to copy the characters from s1 and s2 to s3, and finally adds a null terminator at the end of s3 to indicate the end of the concatenated string.
In the main function, we use fgets to read in the input strings from stdin, call the f function to concatenate the strings, and then print out the concatenated string using printf.
Note that this implementation assumes that the input strings have a maximum length of 100 characters. You can adjust this value as needed for your specific use case.
阅读全文
相关推荐
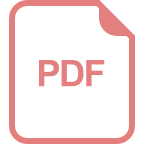
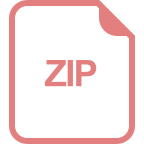













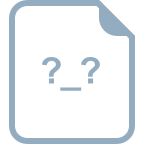
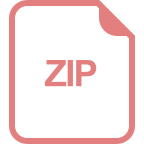
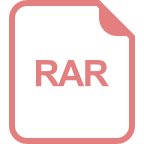