RX蓝牙库连接低功耗蓝牙设备,java代码,maven项目
时间: 2023-11-09 14:15:28 浏览: 215
以下是使用RX蓝牙库连接低功耗蓝牙设备的Java代码示例,适用于Maven项目:
首先,需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.polidea.rxandroidble2</groupId>
<artifactId>rxandroidble</artifactId>
<version>1.12.0</version>
</dependency>
```
然后,可以使用以下代码片段连接低功耗蓝牙设备:
```java
import com.polidea.rxandroidble2.RxBleClient;
import com.polidea.rxandroidble2.RxBleConnection;
import com.polidea.rxandroidble2.exceptions.BleException;
import com.polidea.rxandroidble2.scan.ScanFilter;
import com.polidea.rxandroidble2.scan.ScanSettings;
import io.reactivex.Observable;
import io.reactivex.disposables.Disposable;
public class BluetoothManager {
private RxBleClient rxBleClient;
private RxBleConnection rxBleConnection;
private Disposable scanDisposable;
public void connectToBluetoothDevice() {
// 初始化RxBleClient
rxBleClient = RxBleClient.create(this);
// 扫描低功耗蓝牙设备
scanDisposable = rxBleClient.scanBleDevices(
new ScanSettings.Builder()
.setScanMode(ScanSettings.SCAN_MODE_LOW_POWER)
.build(),
new ScanFilter.Builder()
.setDeviceName("YOUR_DEVICE_NAME")
.build()
).subscribe(
scanResult -> {
// 找到设备
String deviceName = scanResult.getBleDevice().getName();
if (deviceName != null && deviceName.equals("YOUR_DEVICE_NAME")) {
// 连接设备
connectToDevice(scanResult.getBleDevice().getMacAddress());
scanDisposable.dispose();
}
},
throwable -> {
// 扫描出错
}
);
}
private void connectToDevice(String macAddress) {
// 建立低功耗蓝牙连接
Observable<RxBleConnection> connectionObservable = rxBleClient.getBleDevice(macAddress).establishConnection(false);
connectionObservable.subscribe(
rxBleConnection -> {
// 连接成功
this.rxBleConnection = rxBleConnection;
},
throwable -> {
// 连接失败
}
);
}
public void disconnectFromBluetoothDevice() {
// 断开低功耗蓝牙连接
if (rxBleConnection != null) {
rxBleConnection.dispose();
}
}
@Override
protected void onDestroy() {
super.onDestroy();
// 停止扫描
if (scanDisposable != null && !scanDisposable.isDisposed()) {
scanDisposable.dispose();
}
}
}
```
请注意,以上示例代码仅供参考,实际使用时需要根据实际情况进行修改和调整。
阅读全文
相关推荐
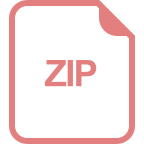
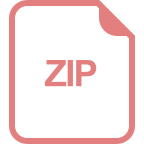
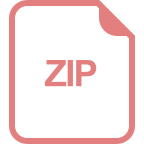
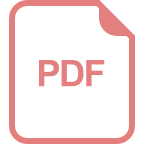
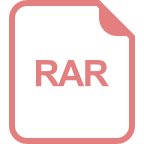
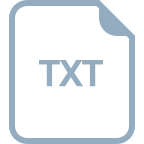
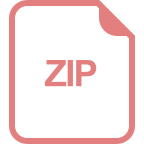
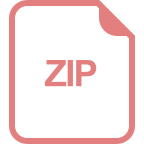
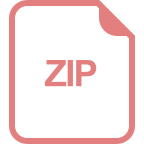
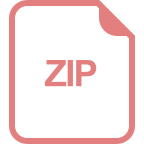
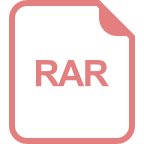
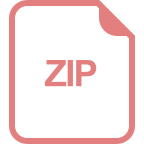
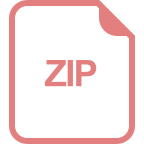
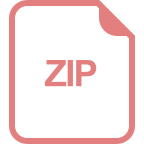
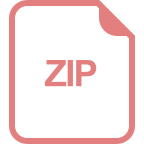
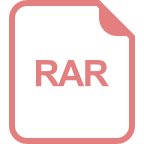