怎么保存yolov5摄像头实时检测所有目标的位置信息
时间: 2023-05-11 19:01:44 浏览: 170
您可以使用Python中的OpenCV库来实现这个功能。首先,您需要使用OpenCV中的VideoCapture函数来打开摄像头并捕获视频流。然后,您可以使用YoloV5模型来检测视频流中的所有目标,并将它们的位置信息保存在一个列表中。最后,您可以将这个列表保存到一个文件中,以便以后使用。
以下是一个示例代码,可以实现这个功能:
```python
import cv2
import numpy as np
import torch
from models.experimental import attempt_load
from utils.general import non_max_suppression
# 加载YoloV5模型
model = attempt_load('yolov5s.pt', map_location=torch.device('cpu'))
# 打开摄像头
cap = cv2.VideoCapture(0)
# 定义输出文件名
output_file = 'detections.txt'
# 循环读取视频流
while True:
# 读取一帧视频
ret, frame = cap.read()
# 将帧转换为模型所需的格式
img = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
img = np.transpose(img, (2, 0, 1))
img = np.expand_dims(img, axis=0)
img = img.astype(np.float32) / 255.0
# 使用YoloV5模型检测目标
with torch.no_grad():
detections = model(torch.from_numpy(img))
detections = non_max_suppression(detections, conf_thres=0.5, iou_thres=0.5)
# 将检测到的目标位置信息保存到文件中
with open(output_file, 'a') as f:
for detection in detections[0]:
x1, y1, x2, y2, conf, cls = detection.tolist()
f.write(f'{x1},{y1},{x2},{y2},{conf},{cls}\n')
# 显示检测结果
for detection in detections[0]:
x1, y1, x2, y2, conf, cls = detection.tolist()
cv2.rectangle(frame, (int(x1), int(y1)), (int(x2), int(y2)), (0, 255, 0), 2)
cv2.putText(frame, f'{cls:.2f}', (int(x1), int(y1) - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow('frame', frame)
# 按下q键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
这个代码将检测到的目标位置信息保存到名为"detections.txt"的文件中。您可以在需要的时候读取这个文件,并使用保存的位置信息来进行后续处理。
相关推荐
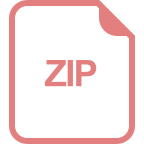














