用SAXWriter类的write输出xml
时间: 2024-02-24 17:53:55 浏览: 94
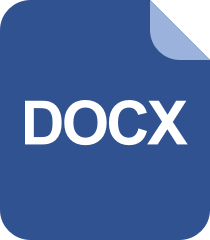
SAX处理xml
好的,以下是使用SAXWriter类的write方法输出xml的示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import javax.xml.transform.Result;
import javax.xml.transform.sax.SAXResult;
import javax.xml.transform.stream.StreamResult;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class XMLWriterExample {
public static void main(String[] args) {
try {
// 创建SAXParser实例
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
// 创建SAXWriter实例
SAXWriter writer = new SAXWriter();
// 设置输出文件
File file = new File("output.xml");
OutputStream outputStream = new FileOutputStream(file);
// 设置输出结果
Result result = new StreamResult(outputStream);
writer.setResult(result);
// 设置XML内容
List<Book> books = new ArrayList<>();
books.add(new Book("Java编程思想", "Bruce Eckel", 2017));
books.add(new Book("Effective Java", "Joshua Bloch", 2018));
// 写入XML
writer.startDocument();
writer.startElement("", "books", "");
for (Book book : books) {
writer.startElement("", "book", "");
writer.startElement("", "title", "");
writer.characters(book.getTitle().toCharArray(), 0, book.getTitle().length());
writer.endElement("", "title", "");
writer.startElement("", "author", "");
writer.characters(book.getAuthor().toCharArray(), 0, book.getAuthor().length());
writer.endElement("", "author", "");
writer.startElement("", "year", "");
writer.characters(String.valueOf(book.getYear()).toCharArray(), 0, String.valueOf(book.getYear()).length());
writer.endElement("", "year", "");
writer.endElement("", "book", "");
}
writer.endElement("", "books", "");
writer.endDocument();
// 关闭输出流
outputStream.close();
System.out.println("XML写入成功!");
} catch (Exception e) {
e.printStackTrace();
}
}
static class Book {
private String title;
private String author;
private int year;
public Book(String title, String author, int year) {
this.title = title;
this.author = author;
this.year = year;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public int getYear() {
return year;
}
}
static class SAXWriter extends DefaultHandler {
private Result result;
public void setResult(Result result) {
this.result = result;
}
public void startDocument() throws SAXException {
if (result != null) {
super.startDocument();
}
}
public void endDocument() throws SAXException {
if (result != null) {
super.endDocument();
}
}
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (result != null) {
super.startElement(uri, localName, qName, attributes);
}
}
public void endElement(String uri, String localName, String qName) throws SAXException {
if (result != null) {
super.endElement(uri, localName, qName);
}
}
public void characters(char[] ch, int start, int length) throws SAXException {
if (result != null) {
super.characters(ch, start, length);
}
}
public void ignorableWhitespace(char[] ch, int start, int length) throws SAXException {
if (result != null) {
super.ignorableWhitespace(ch, start, length);
}
}
public void processingInstruction(String target, String data) throws SAXException {
if (result != null) {
super.processingInstruction(target, data);
}
}
public void setDocumentLocator(org.xml.sax.Locator locator) {
if (result != null) {
super.setDocumentLocator(locator);
}
}
public void startPrefixMapping(String prefix, String uri) throws SAXException {
if (result != null) {
super.startPrefixMapping(prefix, uri);
}
}
public void endPrefixMapping(String prefix) throws SAXException {
if (result != null) {
super.endPrefixMapping(prefix);
}
}
}
}
```
在这个示例中,我们首先创建了一个SAXWriter类,用于写入XML数据。然后,我们设置了输出文件和输出结果,接着我们创建了一个包含书籍信息的列表,并使用SAXWriter类的startElement、characters和endElement方法将这些信息写入XML文件中。最后,我们关闭了输出流并输出了一条成功消息。
阅读全文
相关推荐
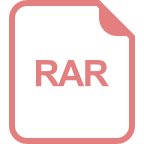
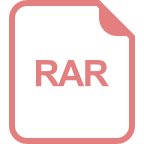
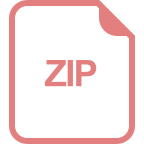
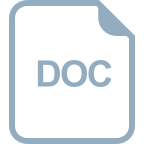
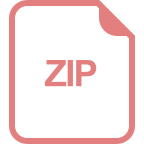
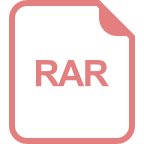
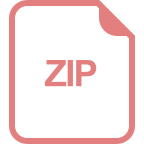
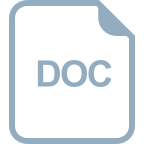
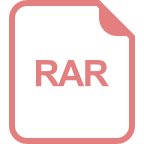
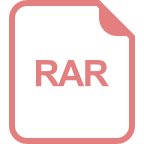
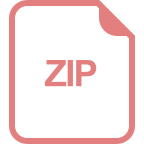
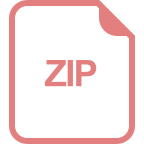
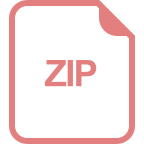
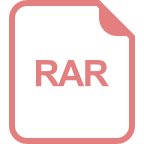