react无缝滚动_react 实现一个无限循环的轮播器,不使用任何hook和轮播UI库
时间: 2024-02-28 17:52:40 浏览: 140
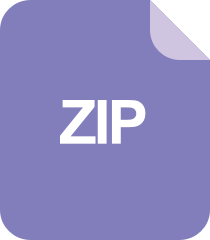
React UI库:一个美观、快速且现代的React UI库,适用于前端开发和UI设计
好的,实现一个无限循环的轮播器,不使用任何hook和轮播UI库,可以按照以下步骤进行:
1. 创建一个React组件,命名为Carousel。
2. 在组件的constructor中初始化state,包括图片数据、当前图片的索引、轮播定时器等。
```
constructor(props) {
super(props);
this.state = {
images: [
{ id: 1, src: 'image1.jpg' },
{ id: 2, src: 'image2.jpg' },
{ id: 3, src: 'image3.jpg' },
{ id: 4, src: 'image4.jpg' },
{ id: 5, src: 'image5.jpg' },
],
currentIndex: 0,
timer: null,
};
}
```
3. 在组件的componentDidMount生命周期方法中,启动轮播定时器。
```
componentDidMount() {
const timer = setInterval(() => {
this.handleNext();
}, 3000);
this.setState({ timer });
}
```
4. 在组件的componentWillUnmount生命周期方法中,清除轮播定时器。
```
componentWillUnmount() {
clearInterval(this.state.timer);
}
```
5. 实现handleNext和handlePrev方法,分别用于切换到下一张和上一张图片。
```
handleNext = () => {
const { images, currentIndex } = this.state;
const nextIndex = currentIndex === images.length - 1 ? 0 : currentIndex + 1;
this.setState({ currentIndex: nextIndex });
}
handlePrev = () => {
const { images, currentIndex } = this.state;
const prevIndex = currentIndex === 0 ? images.length - 1 : currentIndex - 1;
this.setState({ currentIndex: prevIndex });
}
```
6. 在render方法中,渲染轮播图片和切换按钮。
```
render() {
const { images, currentIndex } = this.state;
const prevIndex = currentIndex === 0 ? images.length - 1 : currentIndex - 1;
const nextIndex = currentIndex === images.length - 1 ? 0 : currentIndex + 1;
return (
<div className="carousel">
<div className="carousel-images">
<img src={images[prevIndex].src} alt="prev" />
<img src={images[currentIndex].src} alt="current" />
<img src={images[nextIndex].src} alt="next" />
</div>
<div className="carousel-buttons">
<button onClick={this.handlePrev}>Prev</button>
<button onClick={this.handleNext}>Next</button>
</div>
</div>
);
}
```
7. 在CSS中设置轮播图片的样式,包括绝对定位、宽度、高度等。
```
.carousel-images {
position: relative;
width: 100%;
height: 400px;
}
.carousel-images img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
object-fit: cover;
}
.carousel-images img:nth-child(2) {
z-index: 1;
}
.carousel-images img:first-child {
z-index: 2;
transform: translateX(-100%);
}
.carousel-images img:last-child {
transform: translateX(100%);
}
```
这样就完成了一个简单的React无缝滚动轮播器的实现。
阅读全文
相关推荐
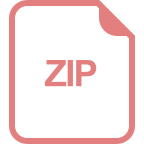
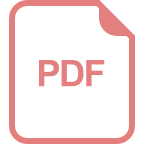















