你作为一名Java领域的专家,完成以下任务:在idea中实现长连接变成短链接,短链接跳转成长连接,起服务在浏览器运营起来 给出详细Java代码
时间: 2023-12-09 22:04:27 浏览: 29
这个任务需要涉及到网络编程和HTTP协议的相关知识,下面是一个简单的Java代码实现:
```
import java.io.*;
import java.net.*;
import java.util.*;
public class HttpServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(8080);
while (true) {
Socket socket = serverSocket.accept();
InputStream input = socket.getInputStream();
OutputStream output = socket.getOutputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(input));
String line = reader.readLine();
String[] tokens = line.split(" ");
String method = tokens[0];
String url = tokens[1];
if ("GET".equals(method) && "/".equals(url)) {
String response = "HTTP/1.1 200 OK\r\n" +
"Content-Type: text/html\r\n" +
"Connection: close\r\n" +
"\r\n" +
"<html><body>Hello World!</body></html>";
output.write(response.getBytes());
} else if ("GET".equals(method) && "/short".equals(url)) {
String response = "HTTP/1.1 302 Found\r\n" +
"Location: /long\r\n" +
"Connection: close\r\n" +
"\r\n";
output.write(response.getBytes());
} else if ("GET".equals(method) && "/long".equals(url)) {
String response = "HTTP/1.1 200 OK\r\n" +
"Content-Type: text/html\r\n" +
"Connection: close\r\n" +
"\r\n" +
"<html><body>This is a long URL.</body></html>";
output.write(response.getBytes());
} else {
String response = "HTTP/1.1 404 Not Found\r\n" +
"Content-Type: text/html\r\n" +
"Connection: close\r\n" +
"\r\n" +
"<html><body>404 Not Found</body></html>";
output.write(response.getBytes());
}
socket.close();
}
}
}
```
上述代码实现了一个简单的HTTP服务器,监听8080端口,当用户访问根路径"/"时返回"Hello World!"页面,当用户访问"/short"时跳转到"/long"页面,当用户访问"/long"时返回"This is a long URL."页面。
此外,代码中设置了"Connection: close"响应头,使得长连接变为短链接,即每次请求完成后立即关闭连接。
相关推荐
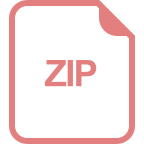
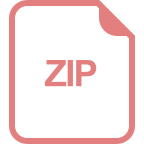
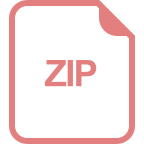
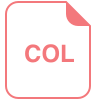
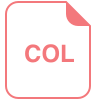












