java 后端实现秒杀接口demo
时间: 2023-12-04 18:05:32 浏览: 33
以下是一个简单的Java后端实现秒杀接口的示例代码:
1. 定义一个秒杀商品的实体类:
```java
public class SeckillGoods {
private Long id; // 商品ID
private String name; // 商品名称
private Integer stock; // 商品库存
private Date startTime; // 秒杀开始时间
private Date endTime; // 秒杀结束时间
// 省略getter和setter方法
}
```
2. 定义一个秒杀服务类:
```java
public interface SeckillService {
/**
* 查询所有秒杀商品
*/
List<SeckillGoods> listAll();
/**
* 根据ID查询秒杀商品
*/
SeckillGoods getById(Long id);
/**
* 开始秒杀
* @param id 秒杀商品ID
* @param userId 用户ID
*/
void startSeckill(Long id, Long userId);
}
```
3. 实现秒杀服务类:
```java
@Service
public class SeckillServiceImpl implements SeckillService {
@Autowired
private SeckillGoodsMapper seckillGoodsMapper;
@Autowired
private SeckillOrderMapper seckillOrderMapper;
@Autowired
private RedisTemplate redisTemplate;
@Override
public List<SeckillGoods> listAll() {
return seckillGoodsMapper.selectAll();
}
@Override
public SeckillGoods getById(Long id) {
return seckillGoodsMapper.selectByPrimaryKey(id);
}
@Override
public void startSeckill(Long id, Long userId) {
// 1. 判断库存是否足够
SeckillGoods seckillGoods = getById(id);
if (seckillGoods == null) {
throw new RuntimeException("秒杀商品不存在");
}
if (seckillGoods.getStock() <= 0) {
throw new RuntimeException("秒杀商品已售罄");
}
// 2. 判断用户是否已经秒杀过该商品
String key = "seckill:user:" + userId + ":goods:" + id;
Boolean isExist = redisTemplate.hasKey(key);
if (isExist) {
throw new RuntimeException("您已经秒杀过该商品");
}
// 3. 减库存
seckillGoods.setStock(seckillGoods.getStock() - 1);
seckillGoodsMapper.updateByPrimaryKeySelective(seckillGoods);
// 4. 创建秒杀订单
SeckillOrder seckillOrder = new SeckillOrder();
seckillOrder.setUserId(userId);
seckillOrder.setGoodsId(id);
seckillOrder.setCreateTime(new Date());
seckillOrder.setAmount(BigDecimal.valueOf(0)); // 省略计算金额
seckillOrderMapper.insertSelective(seckillOrder);
// 5. 记录用户秒杀商品记录到Redis中
redisTemplate.opsForValue().set(key, true);
}
}
```
4. 控制器中使用秒杀服务类:
```java
@RestController
@RequestMapping("/seckill")
public class SeckillController {
@Autowired
private SeckillService seckillService;
@GetMapping("/list")
public List<SeckillGoods> listAll() {
return seckillService.listAll();
}
@GetMapping("/{id}")
public SeckillGoods getById(@PathVariable Long id) {
return seckillService.getById(id);
}
@PostMapping("/{id}")
public void startSeckill(@PathVariable Long id, Long userId) {
seckillService.startSeckill(id, userId);
}
}
```
这样就完成了一个简单的Java后端实现秒杀接口的示例代码。需要注意的是,这只是一个示例,实际应用中还需要针对性能、安全等方面进行优化。
相关推荐
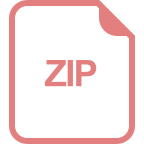














