从串口1接收由计算机发送过来的6个字节的数据串
时间: 2023-12-14 18:37:38 浏览: 41
以下是一个简单的C语言示例代码,演示如何从串口1接收计算机发送过来的6个字节的数据串:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
// 打开串口1
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
perror("open serial port");
exit(1);
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 读取6个字节的数据串
char buf[6];
int bytes_read = read(fd, buf, 6);
if (bytes_read == -1)
{
perror("read serial port");
exit(1);
}
else if (bytes_read != 6)
{
printf("unexpected number of bytes read: %d\n", bytes_read);
exit(1);
}
// 输出读取到的数据串
printf("received: %s\n", buf);
// 关闭串口1
close(fd);
return 0;
}
```
在上面的示例代码中,首先打开了串口1并配置了串口参数。然后使用read函数读取6个字节的数据串,并将读取到的数据输出到终端。最后关闭串口1。注意,在使用read函数时需要注意错误处理和读取到的字节数。
相关推荐
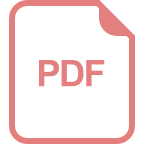
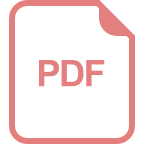














