c++代码转化python
时间: 2023-10-12 17:10:55 浏览: 58
这是一个 C++ 代码示例:
```cpp
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 20;
int c = a + b;
cout << "The sum of a and b is: " << c << endl;
return 0;
}
```
这是相应的 Python 代码:
```python
a = 10
b = 20
c = a + b
print("The sum of a and b is:", c)
```
注意,Python 不需要使用 `namespace`,并且不需要显示声明函数的返回类型。此外,C++ 中使用 `cout` 输出信息,而在 Python 中使用 `print`。
相关问题
将上面代码转化为Python代码
下面是将上面的C++代码转换为Python代码的结果:
```python
import cv2
import numpy as np
def sharpness_detect(src_img):
gaussian_size = 3
if src_img.ndim == 3:
img = cv2.cvtColor(src_img, cv2.COLOR_BGR2GRAY)
else:
img = src_img
out = cv2.GaussianBlur(img, (gaussian_size, gaussian_size), 0, 0)
d_Fver = 0
d_Fhor = 0
d_Bver = 0
d_Bhor = 0
vver = 0
vhor = 0
s_Fver = 0
s_Fhor = 0
s_Vver = 0
s_Vhor = 0
b_Fver = 0.0
b_Fhor = 0.0
blur_F = 0.0
for r in range(img.shape[0]):
for c in range(img.shape[1]):
if r != 0:
d_Fver = abs(img[r, c] - img[r - 1, c])
if c != 0:
d_Fhor = abs(img[r, c] - img[r, c - 1])
if r != 0:
d_Bver = abs(out[r, c] - out[r - 1, c])
if c != 0:
d_Bhor = abs(out[r, c] - out[r, c - 1])
vver = (d_Fver - d_Bver) if d_Fver - d_Bver > 0 else 0
vhor = (d_Fhor - d_Bhor) if d_Fhor - d_Bhor > 0 else 0
s_Fver += d_Fver
s_Fhor += d_Fhor
s_Vver += vver
s_Vhor += vhor
b_Fver = (s_Fver - s_Vver) / (float(s_Fver) + 1)
b_Fhor = (s_Fhor - s_Vhor) / (float(s_Fhor) + 1)
blur_F = b_Fver if b_Fver > b_Fhor else b_Fhor
return 1 - blur_F
```
注意:这段代码中的细节可能与原始C++代码略有不同,但整体思路是相同的。
把以下代码转化为c++代码
以下是Python代码:
```
def reverseString(s):
left, right = 0, len(s)-1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
```
以下是对应的C++代码:
```
#include <vector>
using namespace std;
vector<char> reverseString(vector<char>& s) {
int left = 0, right = s.size()-1;
while (left < right) {
swap(s[left], s[right]);
left++;
right--;
}
return s;
}
```
需要注意的是,在C++中需要使用vector来代替Python中的列表。此外,C++中的swap函数可以用来交换两个变量的值。
相关推荐
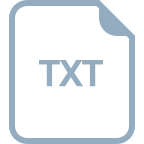
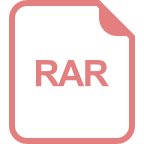












