function numStar(arr1,arr2,WeiXingList,SendCount,ts) { let map1 = new Map() let map2 = new Map() for (let i = 0; i < arr1.length; i++) { let el = (arr1[i].split(","))[7] if (!map1.has(el)) {//如果没有 map1.set(el, 1) } else { let num1 = map1.get(el) num1++; map1.set(el, num1) } } for (let i = 0; i < arr2.length; i++) { let el = (arr2[i].split(","))[7] if (!map2.has(el)) {//如果没有 map2.set(el, 1) } else { let num2 = map2.get(el) num2++; map2.set(el, num2) } } map1.forEach((Value1, key1) => { let p = WeiXingList.indexOf(key1) SendCount[p].push(Value1) }) map2.forEach((Value2,key2)=>{ let pos = WeiXingList.indexOf( key2 +'失败') SendCount[pos].push(Value2) }) for(let q = 0; q < SendCount.length; q++){ if(SendCount[q].length < ts ){ SendCount[q].push("_") } } return SendCount }
时间: 2024-03-16 08:45:01 浏览: 53
根据你提供的代码,我给出以下优化建议:
1. 变量名使用小驼峰命名法,函数名使用动词 + 名词的命名方式,例如 `countStar`。
2. 使用 `const` 声明不会被修改的变量,使用 `let` 声明可能会被修改的变量,以提高代码的可读性和可维护性。
3. 优化循环。使用 `for...of` 循环可以更方便地遍历数组,以及使用解构赋值获取数组元素。
4. 优化判断逻辑。使用三元运算符或逻辑或运算符可以简化判断逻辑,以提高代码的可读性和可维护性。
5. 使用默认参数。可以使用默认参数来设置函数的默认值,以简化函数的调用方式。
综上所述,以下是经过优化后的代码:
```javascript
function countStar(arr1, arr2, WeiXingList, SendCount, ts = 0) {
const map1 = new Map();
const map2 = new Map();
// 统计 arr1 中每个元素出现的次数
for (const [_, __, ___, ____, _____, ______, _______, el] of arr1) {
map1.set(el, (map1.get(el) || 0) + 1);
}
// 统计 arr2 中每个元素出现的次数
for (const [_, __, ___, ____, _____, ______, _______, el] of arr2) {
map2.set(`${el}失败`, (map2.get(`${el}失败`) || 0) + 1);
}
// 将统计结果加入 SendCount 数组中
map1.forEach((value1, key1) => {
const p = WeiXingList.indexOf(key1);
SendCount[p].push(value1);
});
map2.forEach((value2, key2) => {
const pos = WeiXingList.indexOf(key2);
SendCount[pos].push(value2);
});
// 补全 SendCount 数组
for (let i = 0; i < SendCount.length; i++) {
if (SendCount[i].length < ts) {
SendCount[i].push("_");
}
}
return SendCount;
}
```
这样的优化可以提高代码的性能、可读性和可维护性。
阅读全文
相关推荐
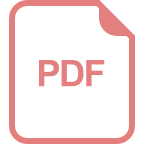
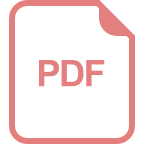
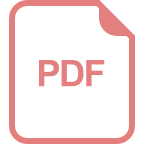


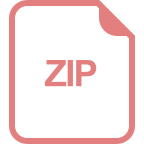
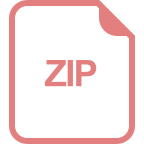
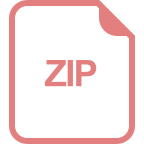
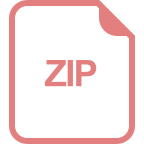
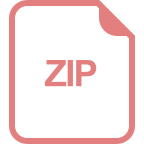
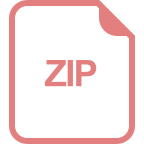
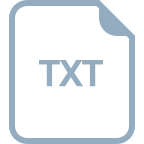
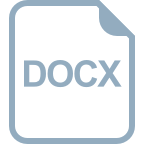
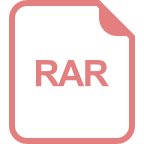