python使用tkinter搭建有增删改查的名片管理项目
时间: 2024-03-06 19:49:13 浏览: 205
好的,我可以帮你解答这个问题。
首先,我们需要导入`tkinter`模块和`sqlite3`模块。`sqlite3`模块是因为我们要使用数据库来存储名片信息。
```python
import tkinter as tk
import sqlite3
```
然后,我们需要创建一个GUI窗口,用于显示我们的名片管理项目。我们可以使用`tkinter`的`Tk`类来创建窗口。
```python
root = tk.Tk()
root.title("名片管理系统")
```
接下来,我们需要创建一个用于显示名片信息的列表框。我们可以使用`tkinter`的`Listbox`类来创建一个列表框,然后使用`sqlite3`模块从数据库中读取名片信息并显示在列表框中。
```python
# 创建一个列表框
listbox = tk.Listbox(root)
listbox.pack()
# 连接数据库
connection = sqlite3.connect("cards.db")
cursor = connection.cursor()
# 从数据库中读取名片信息
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
# 显示名片信息
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
```
现在,我们已经可以显示名片信息了。接下来,我们需要添加一些按钮和文本框,用于添加、修改和删除名片信息。
```python
# 添加按钮和文本框
add_label = tk.Label(root, text="添加名片")
add_label.pack()
add_name_label = tk.Label(root, text="姓名")
add_name_label.pack()
add_name_entry = tk.Entry(root)
add_name_entry.pack()
add_phone_label = tk.Label(root, text="电话")
add_phone_label.pack()
add_phone_entry = tk.Entry(root)
add_phone_entry.pack()
add_email_label = tk.Label(root, text="邮箱")
add_email_label.pack()
add_email_entry = tk.Entry(root)
add_email_entry.pack()
add_button = tk.Button(root, text="添加")
add_button.pack()
# 修改按钮和文本框
edit_label = tk.Label(root, text="修改名片")
edit_label.pack()
edit_id_label = tk.Label(root, text="ID")
edit_id_label.pack()
edit_id_entry = tk.Entry(root)
edit_id_entry.pack()
edit_name_label = tk.Label(root, text="姓名")
edit_name_label.pack()
edit_name_entry = tk.Entry(root)
edit_name_entry.pack()
edit_phone_label = tk.Label(root, text="电话")
edit_phone_label.pack()
edit_phone_entry = tk.Entry(root)
edit_phone_entry.pack()
edit_email_label = tk.Label(root, text="邮箱")
edit_email_label.pack()
edit_email_entry = tk.Entry(root)
edit_email_entry.pack()
edit_button = tk.Button(root, text="修改")
edit_button.pack()
# 删除按钮和文本框
delete_label = tk.Label(root, text="删除名片")
delete_label.pack()
delete_id_label = tk.Label(root, text="ID")
delete_id_label.pack()
delete_id_entry = tk.Entry(root)
delete_id_entry.pack()
delete_button = tk.Button(root, text="删除")
delete_button.pack()
```
现在,我们已经添加了按钮和文本框。接下来,我们需要定义这些按钮的点击事件,以便添加、修改和删除名片信息。
```python
# 添加按钮的点击事件
def add_card():
name = add_name_entry.get()
phone = add_phone_entry.get()
email = add_email_entry.get()
cursor.execute("INSERT INTO cards (name, phone, email) VALUES (?, ?, ?)", (name, phone, email))
connection.commit()
listbox.insert(tk.END, f"{cursor.lastrowid} {name} {phone} {email}")
add_button.config(command=add_card)
# 修改按钮的点击事件
def edit_card():
id = edit_id_entry.get()
name = edit_name_entry.get()
phone = edit_phone_entry.get()
email = edit_email_entry.get()
cursor.execute("UPDATE cards SET name = ?, phone = ?, email = ? WHERE id = ?", (name, phone, email, id))
connection.commit()
listbox.delete(0, tk.END)
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
edit_button.config(command=edit_card)
# 删除按钮的点击事件
def delete_card():
id = delete_id_entry.get()
cursor.execute("DELETE FROM cards WHERE id = ?", (id,))
connection.commit()
listbox.delete(0, tk.END)
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
delete_button.config(command=delete_card)
```
现在,我们已经完成了整个名片管理项目的代码。完整代码如下:
```python
import tkinter as tk
import sqlite3
# 创建窗口
root = tk.Tk()
root.title("名片管理系统")
# 创建列表框和滚动条
listbox = tk.Listbox(root)
listbox.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
scrollbar = tk.Scrollbar(root)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
listbox.config(yscrollcommand=scrollbar.set)
scrollbar.config(command=listbox.yview)
# 连接数据库
connection = sqlite3.connect("cards.db")
cursor = connection.cursor()
# 创建表格
cursor.execute("""
CREATE TABLE IF NOT EXISTS cards (
id INTEGER PRIMARY KEY,
name TEXT,
phone TEXT,
email TEXT
)
""")
connection.commit()
# 从数据库中读取名片信息
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
# 显示名片信息
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
# 添加按钮和文本框
add_label = tk.Label(root, text="添加名片")
add_label.pack()
add_name_label = tk.Label(root, text="姓名")
add_name_label.pack()
add_name_entry = tk.Entry(root)
add_name_entry.pack()
add_phone_label = tk.Label(root, text="电话")
add_phone_label.pack()
add_phone_entry = tk.Entry(root)
add_phone_entry.pack()
add_email_label = tk.Label(root, text="邮箱")
add_email_label.pack()
add_email_entry = tk.Entry(root)
add_email_entry.pack()
add_button = tk.Button(root, text="添加")
add_button.pack()
# 修改按钮和文本框
edit_label = tk.Label(root, text="修改名片")
edit_label.pack()
edit_id_label = tk.Label(root, text="ID")
edit_id_label.pack()
edit_id_entry = tk.Entry(root)
edit_id_entry.pack()
edit_name_label = tk.Label(root, text="姓名")
edit_name_label.pack()
edit_name_entry = tk.Entry(root)
edit_name_entry.pack()
edit_phone_label = tk.Label(root, text="电话")
edit_phone_label.pack()
edit_phone_entry = tk.Entry(root)
edit_phone_entry.pack()
edit_email_label = tk.Label(root, text="邮箱")
edit_email_label.pack()
edit_email_entry = tk.Entry(root)
edit_email_entry.pack()
edit_button = tk.Button(root, text="修改")
edit_button.pack()
# 删除按钮和文本框
delete_label = tk.Label(root, text="删除名片")
delete_label.pack()
delete_id_label = tk.Label(root, text="ID")
delete_id_label.pack()
delete_id_entry = tk.Entry(root)
delete_id_entry.pack()
delete_button = tk.Button(root, text="删除")
delete_button.pack()
# 添加按钮的点击事件
def add_card():
name = add_name_entry.get()
phone = add_phone_entry.get()
email = add_email_entry.get()
cursor.execute("INSERT INTO cards (name, phone, email) VALUES (?, ?, ?)", (name, phone, email))
connection.commit()
listbox.insert(tk.END, f"{cursor.lastrowid} {name} {phone} {email}")
add_button.config(command=add_card)
# 修改按钮的点击事件
def edit_card():
id = edit_id_entry.get()
name = edit_name_entry.get()
phone = edit_phone_entry.get()
email = edit_email_entry.get()
cursor.execute("UPDATE cards SET name = ?, phone = ?, email = ? WHERE id = ?", (name, phone, email, id))
connection.commit()
listbox.delete(0, tk.END)
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
edit_button.config(command=edit_card)
# 删除按钮的点击事件
def delete_card():
id = delete_id_entry.get()
cursor.execute("DELETE FROM cards WHERE id = ?", (id,))
connection.commit()
listbox.delete(0, tk.END)
cursor.execute("SELECT * FROM cards")
cards = cursor.fetchall()
for card in cards:
listbox.insert(tk.END, f"{card[0]} {card[1]} {card[2]} {card[3]}")
delete_button.config(command=delete_card)
# 运行窗口
root.mainloop()
```
希望这个代码能够帮助到你。
阅读全文
相关推荐
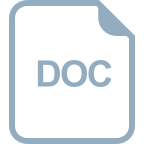
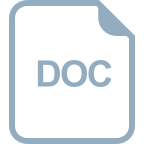
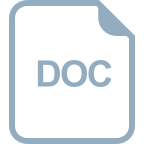
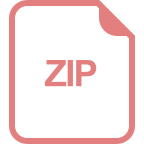
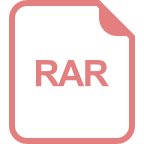
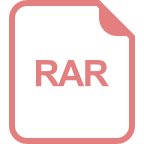
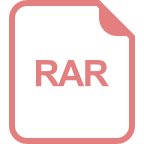
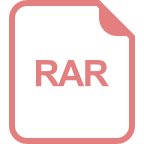
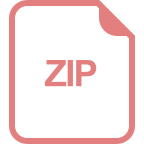
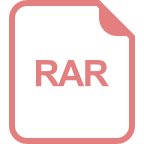
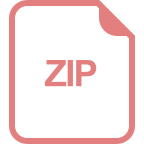
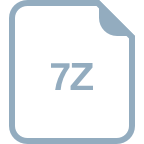
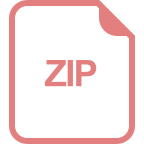
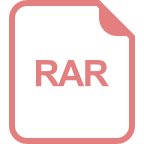
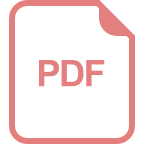
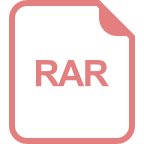
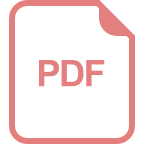
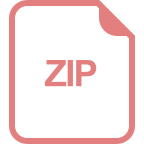