java对mysql使用javaoop方法并且使用占用符对数据进行修改
时间: 2023-06-02 17:01:58 浏览: 144
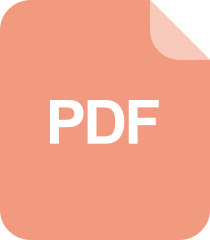
Java对MySQL数据库进行连接、查询和修改操作方法

这是一个示例代码,演示如何使用Java OOP方法连接MySQL数据库,并使用占位符对数据进行修改:
```java
import java.sql.*;
public class MySQLExample {
private Connection conn;
private PreparedStatement stmt;
public void connect(String url, String user, String password) throws SQLException {
conn = DriverManager.getConnection(url, user, password);
}
public void prepareStatement(String sql) throws SQLException {
stmt = conn.prepareStatement(sql);
}
public void setInt(int parameterIndex, int x) throws SQLException {
stmt.setInt(parameterIndex, x);
}
public void setString(int parameterIndex, String x) throws SQLException {
stmt.setString(parameterIndex, x);
}
public void executeUpdate() throws SQLException {
stmt.executeUpdate();
}
public void close() throws SQLException {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
}
public static void main(String[] args) {
MySQLExample example = new MySQLExample();
try {
example.connect("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
example.prepareStatement("UPDATE mytable SET name=? WHERE id=?");
example.setString(1, "John");
example.setInt(2, 1);
example.executeUpdate();
example.close();
System.out.println("Data updated successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们使用了Java的面向对象编程方法实现了一个连接MySQL数据库的类,并提供了一些方法来执行SQL语句。我们使用了占位符来避免SQL注入攻击,通过将输入参数放在占位符中,而不是将它们直接插入SQL语句中。
在main方法中,我们首先连接到MySQL数据库,然后使用prepareStatement方法准备一个SQL语句。接下来,我们使用setString和setInt方法来设置占位符的值,然后使用executeUpdate方法执行SQL语句。最后,我们关闭连接,并打印出成功更新数据的信息。
请注意,这只是一个示例代码,实际使用时需要根据具体情况进行修改和优化。
阅读全文
相关推荐

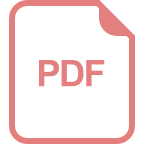
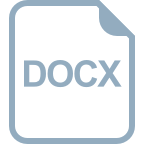
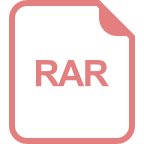
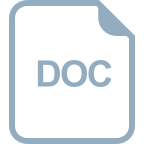
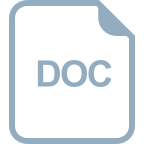
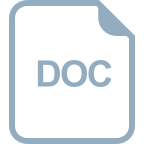
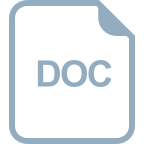
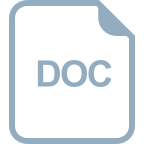
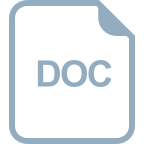
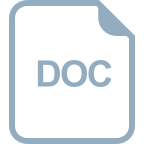
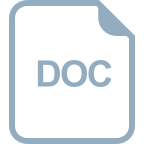
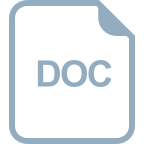
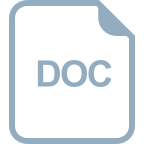
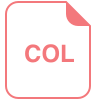
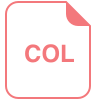