使用mybatis-plus如何分页
时间: 2023-08-31 14:38:20 浏览: 108
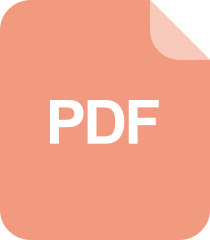
Mybatis实现分页功能

### 回答1:
MyBatis-Plus 提供了分页功能,可以通过 Page 对象进行分页查询。
下面是一个简单的示例:
1. 在 Mapper 接口中定义分页查询方法:
```java
@Mapper
public interface UserMapper extends BaseMapper<User> {
IPage<User> selectPageVo(Page page, @Param("state") Integer state);
}
```
2. 在 Service 层调用分页查询方法:
```java
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Autowired
private UserMapper userMapper;
public IPage<User> selectUserPage(Page page, Integer state) {
return userMapper.selectPageVo(page, state);
}
}
```
3. 在 Controller 层调用 Service 层的方法:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/user")
public IPage<User> getUserPage(Page page, Integer state) {
return userService.selectUserPage(page, state);
}
}
```
在这个示例中,我们使用了 MyBatis-Plus 提供的 Page 对象进行分页查询,通过 `selectPageVo` 方法实现了分页查询功能。
### 回答2:
使用MyBatis-Plus进行分页需要以下步骤:
1. 首先,确保已经正确集成了MyBatis-Plus依赖,并配置了相关的数据库连接信息。
2. 在Mapper接口中定义查询方法,该方法的参数包括分页对象(com.baomidou.mybatisplus.extension.plugins.pagination.Page)和查询条件。
3. 使用MyBatis-Plus提供的PageHelper类创建分页对象,并设置分页参数,如当前页码和每页显示数量。
4. 调用Mapper接口中的查询方法,将分页对象和查询条件作为参数传入。
5. 在查询方法中,使用MyBatis-Plus提供的Page对象进行分页查询,使用Page对象的selectPage方法,并将Page对象和查询条件作为参数传入。
6. 查询结果将会封装在Page对象中,通过Page对象的getRecords方法可以获取查询结果集。
7. 最后,可以通过Page对象的getTotal方法获取总记录数,从而得到总页数和当前页的记录数。
下面是一个示例:
```java
// Mapper接口方法定义
List<User> getUserList(Page<User> page, @Param("name") String name);
// 分页查询示例
Page<User> page = new Page<>(1, 10); // 创建分页对象,当前页码为1,每页显示10条记录
List<User> userList = userMapper.getUserList(page, "张三"); // 调用查询方法,传入分页对象和查询条件
List<User> records = page.getRecords(); // 获取查询结果集
long total = page.getTotal(); // 获取总记录数
long pages = page.getPages(); // 获取总页数
long current = page.getCurrent(); // 获取当前页码
long size = page.getSize(); // 获取每页显示数量
```
这样就可以使用MyBatis-Plus进行分页查询了。
### 回答3:
使用Mybatis-Plus进行分页,首先需要在代码中引入Mybatis-Plus的依赖。
分页查询可以通过Page类来实现,具体步骤如下:
1. 创建一个Page对象:
```java
Page<T> page = new Page<T>(current, size);
```
其中,current代表当前页码,size代表每页记录数。
2. 调用Mybatis-Plus提供的分页查询方法,将Page对象作为参数传入:
```java
IPage<T> result = myMapper.selectPage(page, queryWrapper);
```
其中,myMapper是你自己创建的Mapper接口对象,queryWrapper是条件构造器,用于指定查询条件。
3. 从IPage对象中获取分页查询结果:
```java
List<T> records = result.getRecords(); // 当前页的数据列表
long total = result.getTotal(); // 总记录数
long pages = result.getPages(); // 总页数
```
通过这种方式,我们可以方便地实现分页查询操作。同时,Mybatis-Plus还提供了一些其他的分页查询方法,如selectMapsPage()方法用于返回Map类型的分页查询结果,selectPage()方法的重载版本等,可以根据具体需求进行选择。
需要注意的是,使用Mybatis-Plus进行分页查询时,需要在实体类中声明分页相关的属性,以及对应的getter和setter方法。这些属性包括current(当前页)、size(每页记录数)、total(总记录数)、pages(总页数)等。
阅读全文
相关推荐



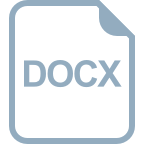









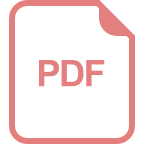