def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop c++ Eigen
时间: 2024-03-20 11:41:17 浏览: 20
The code you provided is written in Python and uses NumPy library for array manipulation. It crops a 3D point cloud data based on the given parameters and returns the cropped data.
Here's the equivalent C++ code using Eigen library for array manipulation:
```
#include <Eigen/Dense>
Eigen::Tensor<float, 3> crop_pointcloud(Eigen::Tensor<float, 3> data_crop, float x_o, float y_o, float x_i, float y_i, float R_o, float R_i, int z_critical) {
int range_z = data_crop.dimension(2);
float K_o = R_o * R_o / range_z;
float K_i = R_i * R_i / range_z;
for (int z=0; z<range_z; z++) {
float r_o = sqrt(z * K_o);
Eigen::Tensor<float, 2> data_layer = data_crop.chip(z, 2);
float d_o = sqrt(x_o * x_o + y_o * y_o);
float d_i = sqrt(x_i * x_i + y_i * y_i);
float r_i = (z < z_critical) ? 0 : sqrt(z * K_i);
data_crop.chip(z, 2) = (d_o > r_o || d_i <= r_i).select(0, data_layer);
}
return data_crop;
}
```
Note: This code assumes that the input data is a 3D tensor of shape (N, M, K), where N, M, and K are the number of points in x, y, and z dimensions, respectively.
相关推荐
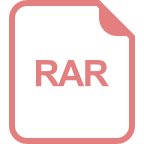
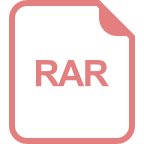



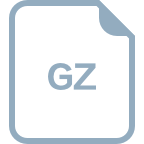
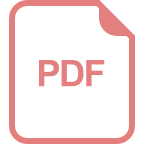
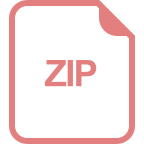