java 浏览器在线预览excel例子
时间: 2023-08-20 19:05:04 浏览: 55
以下是一个使用Java浏览器在线预览Excel的示例代码:
```java
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.net.URL;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
public class ExcelViewer extends JFrame {
private static final long serialVersionUID = 1L;
private JTable table;
public ExcelViewer(String url) {
super("Excel Viewer");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
dispose();
}
});
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
JPanel panel = new JPanel();
panel.setLayout(new BorderLayout());
table = new JTable();
panel.add(new JScrollPane(table));
contentPane.add(panel, BorderLayout.CENTER);
pack();
setLocationRelativeTo(null);
try {
URL excelUrl = new URL(url);
InputStream is = excelUrl.openStream();
Workbook wb = new HSSFWorkbook(is);
Sheet sheet = wb.getSheetAt(0);
int numColumns = sheet.getRow(0).getLastCellNum();
String[] columnNames = new String[numColumns];
for (int i = 0; i < numColumns; i++) {
columnNames[i] = sheet.getRow(0).getCell(i).getStringCellValue();
}
DefaultTableModel model = new DefaultTableModel(columnNames, 0);
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
Object[] rowData = new Object[numColumns];
for (int j = 0; j < numColumns; j++) {
Cell cell = row.getCell(j);
if (cell != null) {
switch (cell.getCellType()) {
case Cell.CELL_TYPE_BLANK:
rowData[j] = "";
break;
case Cell.CELL_TYPE_BOOLEAN:
rowData[j] = cell.getBooleanCellValue();
break;
case Cell.CELL_TYPE_ERROR:
rowData[j] = cell.getErrorCellValue();
break;
case Cell.CELL_TYPE_FORMULA:
ByteArrayOutputStream baos = new ByteArrayOutputStream();
wb.write(baos);
InputStream formulaIs = new ByteArrayInputStream(baos.toByteArray());
Workbook formulaWb = new HSSFWorkbook(formulaIs);
Sheet formulaSheet = formulaWb.getSheetAt(0);
Cell formulaCell = formulaSheet.getRow(row.getRowNum())
.getCell(cell.getColumnIndex());
switch (formulaCell.getCachedFormulaResultType()) {
case Cell.CELL_TYPE_BLANK:
rowData[j] = "";
break;
case Cell.CELL_TYPE_BOOLEAN:
rowData[j] = formulaCell.getBooleanCellValue();
break;
case Cell.CELL_TYPE_ERROR:
rowData[j] = formulaCell.getErrorCellValue();
break;
case Cell.CELL_TYPE_NUMERIC:
rowData[j] = formulaCell.getNumericCellValue();
break;
case Cell.CELL_TYPE_STRING:
rowData[j] = formulaCell.getStringCellValue();
break;
}
break;
case Cell.CELL_TYPE_NUMERIC:
rowData[j] = cell.getNumericCellValue();
break;
case Cell.CELL_TYPE_STRING:
rowData[j] = cell.getStringCellValue();
break;
}
} else {
rowData[j] = "";
}
}
model.addRow(rowData);
}
table.setModel(model);
} catch (Exception e) {
JOptionPane.showMessageDialog(this, "Error loading Excel file: " + e.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}
public static void main(String[] args) {
new ExcelViewer("http://example.com/example.xls").setVisible(true);
}
}
```
这个Java程序可以从指定的URL加载Excel文件,并将其显示在一个JTable中。可以使用此代码作为基础,根据需要进行修改和扩展。
相关推荐
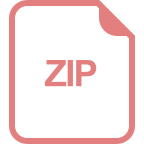
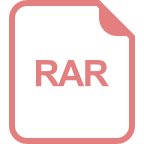















