编写单链表的LinList.c和LinList.h头文件。
时间: 2024-04-29 08:26:53 浏览: 72
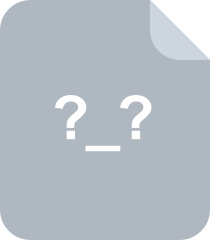
单链表表头文件
LinList.h:
```
#ifndef _LINLIST_H_
#define _LINLIST_H_
typedef struct _node {
int data;
struct _node *next;
} Node;
typedef struct _list {
int size;
Node *head;
} LinList;
void initList(LinList *list);
void addNode(LinList *list, int data);
void insertNode(LinList *list, int index, int data);
void removeNode(LinList *list, int index);
void clearList(LinList *list);
void printList(LinList *list);
#endif
```
LinList.c:
```
#include <stdio.h>
#include <stdlib.h>
#include "LinList.h"
void initList(LinList *list) {
list->size = 0;
list->head = NULL;
}
void addNode(LinList *list, int data) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node *current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
list->size++;
}
void insertNode(LinList *list, int index, int data) {
if (index < 0 || index > list->size) {
printf("Invalid index!\n");
return;
}
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = data;
if (index == 0) {
newNode->next = list->head;
list->head = newNode;
} else {
Node *current = list->head;
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
list->size++;
}
void removeNode(LinList *list, int index) {
if (index < 0 || index >= list->size) {
printf("Invalid index!\n");
return;
}
Node *current = list->head;
if (index == 0) {
list->head = current->next;
} else {
for (int i = 0; i < index - 1; i++) {
current = current->next;
}
Node *temp = current->next;
current->next = temp->next;
free(temp);
}
list->size--;
}
void clearList(LinList *list) {
Node *current = list->head;
Node *temp;
while (current != NULL) {
temp = current;
current = current->next;
free(temp);
}
list->size = 0;
list->head = NULL;
}
void printList(LinList *list) {
Node *current = list->head;
printf("List size: %d\n", list->size);
printf("List contents: ");
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
阅读全文
相关推荐
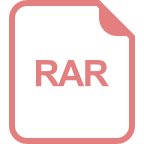



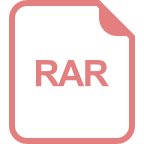
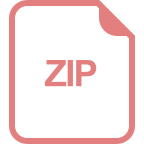
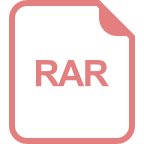
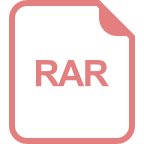
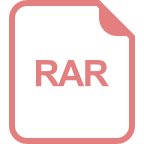
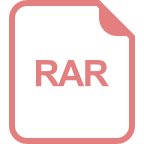
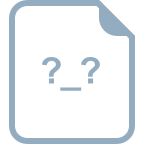
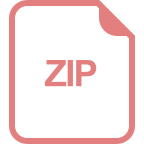