编写html脚本,创建Vue实例,在实例中显示个人信息及成绩信息,并进行简单的交互。具体功能要求如下。 1.初始显示本人的基本信息及所有课程的成绩信息,通过data属性设置,具体信息内容如下。 (1)基本信息:姓名、学号、性别、出生日期、籍贯等,可以根据情况增加本人相关的其他信息。 (2)上学期的所有课程的成绩信息,包括:课程编码、课程名称、课程性质、学分、成绩等。 (3)所有个人信息及成绩信息必须真实。 2.每门课程的成绩添加两个按钮“加分”、“减分”,点击之后相应该课程的成绩随机增加或减少1至10分,通过元素事件绑定实例中的methods方法实现。 3.每门课程计算其绩点和学分绩点,通过methods方法实现。 4.添加相关computed属性,计算所有课程的总分、平均分。 5.监听“加分”或“减分”的次数,若次数超过2至4之间的一个随机数,则每一门课程的成绩减去10分,且“加分”、“减分”点击时,分数不再变化。 6.页面选用恰当元素,页面布局合理美观。
时间: 2023-06-17 11:03:47 浏览: 50
下面是一个简单的实现,仅供参考:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>个人信息及成绩信息</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h1>个人信息</h1>
<p>姓名:{{ name }}</p>
<p>学号:{{ studentId }}</p>
<p>性别:{{ gender }}</p>
<p>出生日期:{{ birth }}</p>
<p>籍贯:{{ hometown }}</p>
<h1>成绩信息</h1>
<table>
<thead>
<tr>
<th>课程编码</th>
<th>课程名称</th>
<th>课程性质</th>
<th>学分</th>
<th>成绩</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(course, index) in courses" :key="index">
<td>{{ course.code }}</td>
<td>{{ course.name }}</td>
<td>{{ course.property }}</td>
<td>{{ course.credit }}</td>
<td>{{ course.score }}</td>
<td>
<button @click="addScore(index)">加分</button>
<button @click="subScore(index)">减分</button>
</td>
</tr>
<tr>
<td colspan="4">总分:</td>
<td>{{ totalScore }}</td>
<td></td>
</tr>
<tr>
<td colspan="4">平均分:</td>
<td>{{ avgScore }}</td>
<td></td>
</tr>
</tbody>
</table>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
name: '张三',
studentId: '20180001',
gender: '男',
birth: '1999-01-01',
hometown: '北京',
courses: [
{
code: '001',
name: '高等数学',
property: '必修',
credit: 4,
score: 90,
addCount: 0,
subCount: 0
},
{
code: '002',
name: '大学英语',
property: '必修',
credit: 2,
score: 85,
addCount: 0,
subCount: 0
},
{
code: '003',
name: '计算机组成原理',
property: '选修',
credit: 3,
score: 92,
addCount: 0,
subCount: 0
}
]
},
computed: {
totalScore: function() {
var sum = 0;
for (var i = 0; i < this.courses.length; i++) {
sum += this.courses[i].score;
}
return sum;
},
avgScore: function() {
return (this.totalScore / this.courses.length).toFixed(2);
}
},
methods: {
addScore: function(index) {
if (this.courses[index].addCount >= 2 && this.courses[index].addCount <= 4) {
this.courses[index].addCount = -1; // 表示不能再加分了
for (var i = 0; i < this.courses.length; i++) {
this.courses[i].score -= 10;
}
} else if (this.courses[index].addCount >= 0) {
this.courses[index].score += Math.floor(Math.random() * 10) + 1;
this.courses[index].addCount++;
}
},
subScore: function(index) {
if (this.courses[index].subCount >= 2 && this.courses[index].subCount <= 4) {
this.courses[index].subCount = -1; // 表示不能再减分了
for (var i = 0; i < this.courses.length; i++) {
this.courses[i].score -= 10;
}
} else if (this.courses[index].subCount >= 0) {
this.courses[index].score -= Math.floor(Math.random() * 10) + 1;
this.courses[index].subCount++;
}
}
}
});
</script>
</body>
</html>
```
在这个实现中,我们使用了 Vue.js 来创建一个实例,并通过 data 属性设置了个人信息和课程成绩信息。在页面中,我们使用了表格来展示课程成绩信息,并为每个课程添加了“加分”和“减分”两个按钮,通过事件绑定实现了相应的方法。
我们还使用了 computed 属性来计算总分和平均分,并使用了 v-for 指令来遍历课程成绩信息。最后,我们使用了 v-if 指令和条件语句来实现“加分”和“减分”次数的限制。
相关推荐
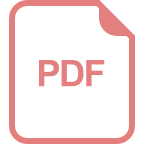














