TypeError: 'float' object is not iterable
时间: 2023-10-16 15:15:32 浏览: 73
这个错误通常是因为你在尝试迭代一个浮点数类型的变量,而实际上浮点数是不可迭代的。请检查你的代码,并确认你正在尝试迭代一个可迭代对象,如列表或元组。如果你确定要迭代一个浮点数,你可以将它放到一个单元素的列表中,然后再进行迭代。例如:
```
num = 3.14
for n in [num]:
print(n)
```
相关问题
zip TypeError: float object is not iterable
This error occurs when you try to iterate over a float object using a loop. A float is a numeric data type in Python that represents a decimal number. However, you cannot iterate over a float as it is not an iterable object.
For example, suppose you have a float value and you try to iterate over it using a for loop:
```
my_float = 3.14
for num in my_float:
print(num)
```
This code will result in a TypeError because you cannot iterate over a float.
To fix this error, you need to ensure that you are iterating over an iterable object, such as a list or a tuple. If you need to convert a float to an iterable object, you can do so by wrapping it in a list or tuple:
```
my_float = 3.14
my_list = [my_float]
for num in my_list:
print(num)
```
This code will iterate over the list containing the float value, rather than the float itself.
zip TypeError: 'float' object is not iterable
This error occurs when you try to iterate over a float number using the zip function. The zip function is used to combine two or more iterables into a single iterable object.
For example, consider the following code:
```
a = [1, 2, 3]
b = 4.5
c = [6, 7, 8]
zipped = zip(a, b, c)
```
In this code, we are trying to zip three iterables - a list, a float, and another list. However, since a float is not iterable, Python raises a TypeError.
To fix this error, you need to make sure that all the objects you pass to the zip function are iterable. In the above example, you can convert the float to a list or a tuple before passing it to the zip function:
```
a = [1, 2, 3]
b = [4.5]
c = [6, 7, 8]
zipped = zip(a, b, c)
```
Here, we have converted the float to a list containing a single element. Now, the zip function can combine all three iterables without any errors.
阅读全文
相关推荐
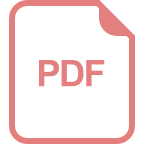
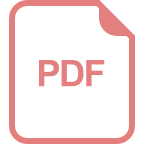




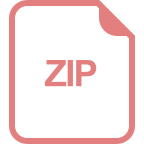
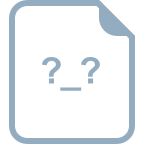
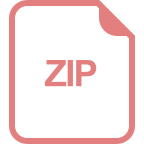
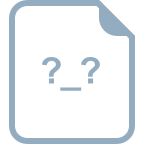
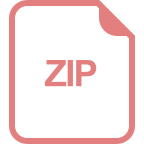
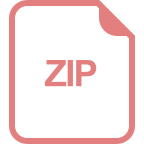