python YOLO v5实现图像模型检测代码
时间: 2024-05-14 19:12:08 浏览: 112
抱歉,作为AI语言模型,我无法生成实际代码。以下是YOLO v5的Python代码示例,供您参考:
1. 导入必要的库
```python
import torch
import cv2
import numpy as np
from PIL import Image
from torchvision import transforms
from models.experimental import attempt_load
from utils.general import non_max_suppression, scale_coords
from utils.plots import plot_one_box
```
2. 定义函数
```python
def detect(image_path, weights_path, conf_thres=0.25, iou_thres=0.45, device='cpu'):
# Load model
model = attempt_load(weights_path, map_location=device)
# Set model to evaluation mode
model.eval()
# Define transforms for input image
transform = transforms.Compose([
transforms.Resize((640, 640)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# Load image
img = Image.open(image_path)
# Apply transforms
img = transform(img).unsqueeze(0)
# Move image to device
img = img.to(device)
# Make predictions
with torch.no_grad():
# Get prediction
pred = model(img)
# Apply non-maximum suppression
pred = non_max_suppression(pred, conf_thres, iou_thres)
# Get image size
img_size = img.shape[-2:]
# Get prediction boxes and labels
boxes = []
labels = []
scores = []
for i, det in enumerate(pred):
# Rescale boxes from 640 to image size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img_size).round()
for *xyxy, conf, cls in reversed(det):
label = model.names[int(cls)]
score = float(conf)
# Add box and label to lists
boxes.append(xyxy)
labels.append(label)
scores.append(score)
return boxes, labels, scores
```
3. 运行检测
```python
if __name__ == '__main__':
# Define device (GPU or CPU)
device = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu')
# Define paths to image and model weights
image_path = 'path/to/image.jpg'
weights_path = 'path/to/weights.pt'
# Run detection
boxes, labels, scores = detect(image_path, weights_path, device=device)
# Load image
img = cv2.imread(image_path)
# Plot boxes on image
for box, label, score in zip(boxes, labels, scores):
plot_one_box(box, img, label=label, color=(0, 255, 0), line_thickness=2)
# Show image
cv2.imshow('Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
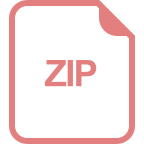
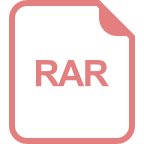
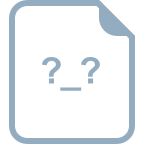
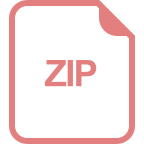
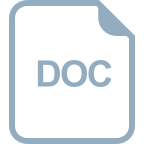
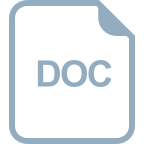
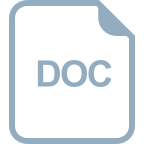

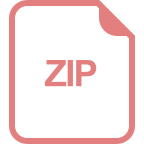
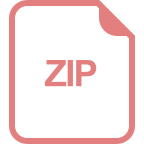
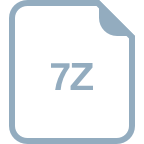
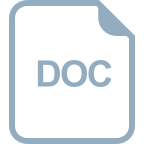
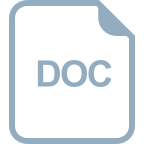
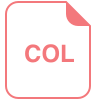
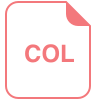
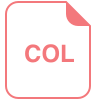
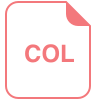
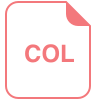