在项目中整合MyBatis或者MyBatisPlus,并使用单元测试类向t_account表中分别新增三个三种角色的用户,注意新增时,密码要使用加密编码器(BCryptPasswordEncoder)进行加密后存储到数据库。
时间: 2024-02-01 22:17:08 浏览: 69
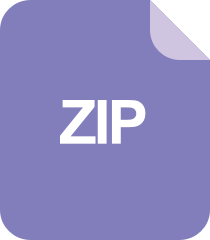
SpringBoot 整合 MyBatisPlus 2.x和MyBatisPlus 3.x【完整源码+数据库】

好的,下面是整合MyBatisPlus并使用单元测试类向t_account表中新增三个不同角色的用户的步骤:
1. 添加MyBatisPlus依赖
在pom.xml文件中添加MyBatisPlus的依赖:
```xml
<!-- MyBatis Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version>
</dependency>
```
2. 配置数据源和MyBatisPlus
在application.properties中配置数据源和MyBatisPlus:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=GMT%2B8
spring.datasource.username=root
spring.datasource.password=root
# MyBatis Plus
mybatis-plus.mapper-locations=classpath*:mapper/*.xml
mybatis-plus.type-aliases-package=com.example.demo.entity
```
3. 创建实体类和Mapper接口
在com.example.demo.entity包下创建Account实体类:
```java
@Data
public class Account {
private Long id;
private String username;
private String password;
private String role;
}
```
在com.example.demo.mapper包下创建AccountMapper接口:
```java
public interface AccountMapper extends BaseMapper<Account> {
}
```
4. 创建加密编码器
在com.example.demo.config包下创建PasswordEncoderConfig类:
```java
@Configuration
public class PasswordEncoderConfig {
@Bean
public BCryptPasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
5. 创建单元测试类
在com.example.demo包下创建AccountTest类:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class AccountTest {
@Autowired
private AccountMapper accountMapper;
@Autowired
private BCryptPasswordEncoder passwordEncoder;
@Test
public void testInsert() {
Account account1 = new Account();
account1.setUsername("user1");
account1.setPassword(passwordEncoder.encode("password1"));
account1.setRole("ROLE_USER");
accountMapper.insert(account1);
Account account2 = new Account();
account2.setUsername("admin1");
account2.setPassword(passwordEncoder.encode("password2"));
account2.setRole("ROLE_ADMIN");
accountMapper.insert(account2);
Account account3 = new Account();
account3.setUsername("guest1");
account3.setPassword(passwordEncoder.encode("password3"));
account3.setRole("ROLE_GUEST");
accountMapper.insert(account3);
}
}
```
6. 运行单元测试
运行单元测试,查看是否成功向t_account表中新增了三个不同角色的用户,并且密码已经加密存储到数据库中。
阅读全文
相关推荐
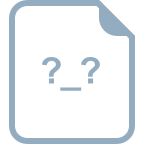
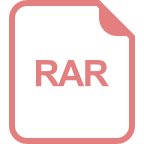
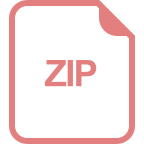
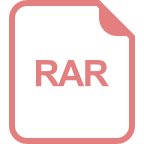
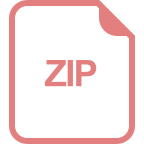
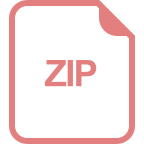
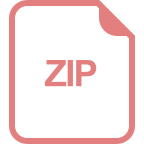
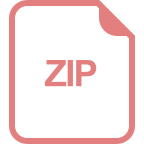
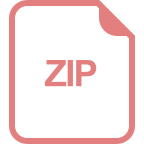
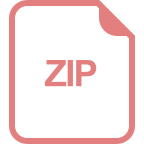
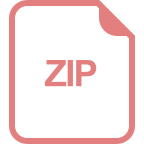
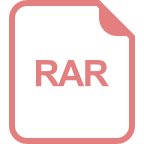
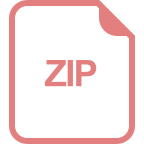
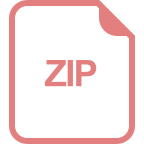