img_path = askopenfilename(initialdir='./testImage', title='选择待识别图片', filetypes=[("jpg", "*.jpg"), ("png", "*.png")]) print(img_path) if img_path: img = cv2.imdecode(fromfile(img_path, dtype=uint8), cv2.IMREAD_COLOR)#彩色 #使用OpenCV库读取指定路径的图像文件,并解码为对应的NumPy数组格式 self.show(img, 400, oriImg) colors, lisencePlates = self.getROI(img) for m in range(len(lisencePlates)): self.show(lisencePlates[m], 40, ROIImg) letters = self.getLetters(lisencePlates[m], colors[m]) results = [] for letter in letters: feature = self.getFeature(letter) result = self.sort(feature, trainingMat, labels, 5) results.append(result) print(result) recogResult = ','.join(results) resultShow.configure(text=recogResult)
时间: 2024-02-14 12:22:35 浏览: 100
这段代码似乎是一个自动识别车牌的程序,它会提示用户选择待识别的车牌图像文件。如果用户选择了文件,程序会读取该文件并将其显示在程序界面上。接着,程序会使用一些图像处理技术(如颜色分割)来获取车牌区域,并对这些车牌进行字符分割。对于每个字符,程序会使用一些特征提取方法来提取其特征,并将其与已知的字符样本进行比较,找到最相似的前五个字符。最后,程序将识别结果以逗号分隔的形式显示在resultShow中。但具体的实现还需要更多上下文信息才能确定。
相关问题
from tkinter import * from tkinter import filedialog from tkinter import messagebox from tqdm import tk from twisted.python import filepath # 初始化窗口 root = Tk() root.title("视频暴力检测系统") root.geometry("300x200") # 选择文件按钮处理函数 def select_file(): filepath = filedialog.askopenfilename(initialdir="/", title="选择文件", filetypes=(("MP4 files", "*.mp4"), ("all files", "*.*"))) if filepath: # 在文件路径文本框中显示所选文件路径 filepath_entry.delete(0, END) filepath_entry.insert(0, filepath) # 检测按钮处理函数 def detect_violence(): filepath = filepath_entry.get() if not filepath: messagebox.showerror("错误", "请选择要检测的视频文件") return # TODO: 在此处编写具体的视频暴力检测代码 messagebox.showinfo("提示", "检测完成") # 文件路径标签和文本框 filepath_label = Label(root, text="文件路径:") filepath_label.pack() filepath_entry = Entry(root, width=30) filepath_entry.pack() # 选择文件按钮 select_file_button = Button(root, text="选择文件", command=select_file) select_file_button.pack() # 检测按钮 detect_button = Button(root, text="检测", command=detect_violence) detect_button.pack() def button_callback(): print("Button clicked") def mouse_callback(event): print("Mouse clicked at", event.x, event.y) # 运行窗口 root.mainloop() 为此程序加入回调程序,实现GUI界面与程序连接起来。
可以在选择文件和检测按钮的回调函数中添加具体的视频暴力检测代码。例如,在检测按钮的回调函数 `detect_violence` 中,可以调用你的视频暴力检测模型进行检测。同时,可以在检测完成后弹出提示框,向用户显示检测结果。代码示例:
```python
# 检测按钮处理函数
def detect_violence():
filepath = filepath_entry.get()
if not filepath:
messagebox.showerror("错误", "请选择要检测的视频文件")
return
# TODO: 调用视频暴力检测模型进行检测
is_violent = check_violence(filepath)
if is_violent:
messagebox.showwarning("警告", "视频中存在暴力内容")
else:
messagebox.showinfo("提示", "视频中不存在暴力内容")
```
其中 `check_violence` 是你的视频暴力检测模型的接口,可以根据具体的实现进行修改。同时,可以在选择文件的回调函数中,将所选文件的路径显示在文本框中,以便用户确认选择的文件是否正确。代码示例:
```python
# 选择文件按钮处理函数
def select_file():
filepath = filedialog.askopenfilename(initialdir="/", title="选择文件", filetypes=(("MP4 files", "*.mp4"), ("all files", "*.*")))
if filepath:
# 在文件路径文本框中显示所选文件路径
filepath_entry.delete(0, END)
filepath_entry.insert(0, filepath)
```
另外,如果需要添加其他的回调函数,例如按钮点击或鼠标点击事件,可以使用 `command` 或 `bind` 方法将回调函数与相应的控件绑定。例如:
```python
# 按钮点击事件回调函数
def button_callback():
print("Button clicked")
# 鼠标点击事件回调函数
def mouse_callback(event):
print("Mouse clicked at", event.x, event.y)
# 创建按钮和标签
button = Button(root, text="Click me", command=button_callback)
label = Label(root, text="Hello, world!")
# 将按钮和标签添加到窗口
button.pack()
label.pack()
# 绑定鼠标点击事件
label.bind("<Button-1>", mouse_callback)
# 运行窗口
root.mainloop()
```
window = tk.Tk() window.title("三维显示系统登录界面") window.geometry("805x660") # 背景画布 import tkinter canvas = tk.Canvas(window,width=10250,height=100) img_gif = tkinter.PhotoImage(file='title3.gif') tkinter.Label(window, image=img_gif).place(relx=-0, rely=0) canvas.pack()sign_in_button = tk.Button(window,text="登录",command=sign_in_f) sign_up_button = tk.Button(window,text="注册",command=sign_up_f) sign_in_button.place(x=350,y=600) sign_up_button.place(x=470,y=600)加入文件调用按钮
下面是修改后的代码,加入了一个按钮,可以让用户选择文件:
```python
import tkinter as tk
from tkinter import filedialog
def sign_in_f():
pass
def sign_up_f():
pass
def open_file():
filename = filedialog.askopenfilename(initialdir="/", title="Select file",
filetypes=(("Text files", "*.txt*"), ("all files", "*.*")))
print(filename)
window = tk.Tk()
window.title("三维显示系统登录界面")
window.geometry("805x660")
# 背景画布
canvas = tk.Canvas(window, width=10250, height=100)
img_gif = tk.PhotoImage(file='title3.gif')
tk.Label(window, image=img_gif).place(relx=-0, rely=0)
canvas.pack()
# 登录和注册按钮
sign_in_button = tk.Button(window, text="登录", command=sign_in_f)
sign_up_button = tk.Button(window, text="注册", command=sign_up_f)
sign_in_button.place(x=350, y=600)
sign_up_button.place(x=470, y=600)
# 文件调用按钮
file_button = tk.Button(window, text="选择文件", command=open_file)
file_button.place(x=600, y=600)
window.mainloop()
```
在这个例子中,我们定义了一个新的函数`open_file()`,当用户点击文件按钮时,调用该函数打开文件对话框。当用户选择文件后,文件的路径会打印到控制台上。你可以根据需要修改`open_file()`函数的实现,以便在GUI中显示所选文件的路径。
阅读全文
相关推荐
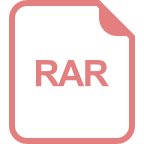
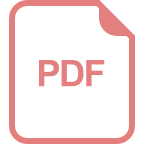
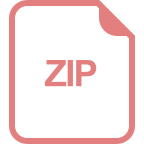


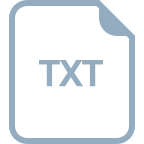











