要求用c++语言编写系统进行资源调度的程序 有以下两种要求: (1)设计3~4个并发进程,共享系统的10个同类不可抢占的资源。各进程动态进行资源的申请和释放。 (2)用银行家算法
时间: 2023-09-10 11:05:44 浏览: 126
### 回答1:
下面是一个简单的用C++编写的系统资源调度程序,实现了以下要求:
1. 设计了3个并发进程,分别为进程A、B、C。
2. 共享了10个同类不可抢占的资源。
3. 每个进程动态申请和释放资源,保证安全性。
4. 使用了银行家算法,确保系统不会进入不安全状态。
代码如下:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int n = 3; // 进程数
const int m = 10; // 资源数
// 各进程的最大需求资源量
int max_demand[n][m] = {{3, 1, 0, 1, 2, 1, 0, 1, 0, 2},
{2, 0, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 1, 1, 2, 1, 0, 1, 0, 1, 1}};
// 各进程已分配的资源量
int allocation[n][m] = {0};
// 各进程还需要的资源量
int need[n][m] = {0};
// 系统可用的资源量
int available[m] = {3, 3, 2, 1, 2, 1, 2, 1, 1, 2};
// 各进程是否完成的标记
bool finish[n] = {false};
// 银行家算法
bool banker_algorithm() {
// 计算各进程还需要的资源量
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
need[i][j] = max_demand[i][j] - allocation[i][j];
}
}
vector<int> work(available, available + m); // 工作向量
vector<bool> safe(n, false); // 安全序列
int count = 0; // 已完成的进程数
while (count < n) {
bool found = false; // 是否找到可分配的进程
for (int i = 0; i < n; i++) {
if (!finish[i]) {
bool can_allocate = true;
for (int j = 0; j < m; j++) {
if (need[i][j] > work[j]) {
can_allocate = false;
break;
}
}
if (can_allocate) {
// 分配资源
for (int j = 0; j < m; j++) {
work[j] += allocation[i][j];
}
finish[i] = true;
safe[count] = true;
count++;
found = true;
}
}
}
if (!found) {
// 没有找到可分配的进程,系统不安全
return false;
}
}
// 打印安全序列
cout << "安全序列为:";
for (int i = 0; i < n; i++) {
if (safe[i]) {
cout << "P" << i << " ";
}
}
cout << endl;
return true;
}
// 分配资源
bool allocate_resources(int process_id, int request[]) {
// 检查请求是否合法
for (int i = 0; i < m; i++) {
if (request[i] > need[process_id][i] || request[i] > available[i]) {
return false;
}
}
// 尝试分配资源
for (int i = 0; i < m; i++) {
available[i] -= request[i];
allocation[process_id][i] += request[i];
need[process_id][i] -= request[i];
}
// 检查系统是否仍然安全
if (banker_algorithm()) {
return true;
} else {
// 回滚分配
for (int i = 0; i < m; i++) {
available[i] += request[i];
allocation[process_id][i] -= request[i];
need[process_id][i] += request[i];
}
return false;
}
}
// 释放资源
void release_resources(int process_id, int release[]) {
for (int i = 0; i < m; i++) {
available[i] += release[i];
allocation[process_id][i] -= release[i];
need[process_id][i] += release[i];
}
}
int main() {
// 初始化资源分配情况
allocation[0][0] = 1;
allocation[0][4] = 2;
allocation[0][5] = 1;
allocation[1][0] = 2;
allocation[1][2] = 1;
allocation[1][3] = 1;
allocation[1][4] = 1;
allocation[1][5] = 1;
allocation[1][6] = 1;
allocation[1][7] = 1;
allocation[1][8] = 1;
allocation[2][1] = 1;
allocation[2][2] = 1;
allocation[2][3] = 2;
allocation[2][6] = 1;
allocation[2][9] = 1;
banker_algorithm();
// 进程A请求资源
int request_a[m] = {0, 0, 0, 0, 0, 0, 0, 1, 0, 1};
if (allocate_resources(0, request_a)) {
cout << "进程A请求资源成功" << endl;
} else {
cout << "进程A请求资源失败" << endl;
}
banker_algorithm();
// 进程B释放资源
int release_b[m] = {1, 0, 1, 0, 0, 0, 0, 0, 0, 0};
release_resources(1, release_b);
banker_algorithm();
// 进程C请求资源
int request_c[m] = {0, 2, 0, 0, 1, 0, 0, 0, 0, 0};
if (allocate_resources(2, request_c)) {
cout << "进程C请求资源成功" << endl;
} else {
cout << "进程C请求资源失败" << endl;
}
banker_algorithm();
return 0;
}
```
该程序使用了静态分配的方式,即在程序开始时就确定了每个进程的最大需求资源量和已分配资源量。在实际的操作系统中,进程的资源需求和分配往往是动态变化的,需要使用动态分配的方式来实现。
### 回答2:
要求用C语言编写系统进行资源调度的程序有以下两种要求:
(1)设计3〜4个并发进程,共享系统的10个同类不可抢占的资源。各进程动态进行资源的申请和释放。
首先我们需要定义一个结构体来表示进程和资源的状态:
```c
struct Process {
int allocated[10]; // 已分配的资源数量
int max[10]; // 最大资源需求数量
int need[10]; // 剩余资源需求数量
int finished; // 标记进程是否完成
};
```
然后我们可以利用一个循环来模拟进程的运行和资源的申请和释放:
```c
int main() {
struct Process processes[4]; // 创建4个进程
int available[10]; // 可用资源数量
// 初始化进程、资源和可用资源数量
while (1) {
// 检查是否有进程能够完成
int found = 0;
for (int i = 0; i < 4; i++) {
if (!processes[i].finished) {
int j;
for (j = 0; j < 10; j++) {
if (processes[i].need[j] > available[j]) {
break; // 进程无法完成,继续下一个进程
}
}
if (j == 10) {
// 进程可以完成,释放资源
for (int k = 0; k < 10; k++) {
available[k] += processes[i].allocated[k];
}
processes[i].finished = 1;
found = 1;
}
}
}
if (!found) {
break; // 没有进程可以完成
}
}
// 输出进程完成状态
for (int i = 0; i < 4; i++) {
printf("Process %d finished: %d\n", i, processes[i].finished);
}
return 0;
}
```
(2)用银行家算法。
银行家算法用于预防死锁的发生,需要注意的是只有当所有进程都能完成时,才允许进程申请资源。以下是使用银行家算法的资源调度程序的示例:
```c
int main() {
struct Process processes[4]; // 创建4个进程
int available[10]; // 可用资源数量
// 初始化进程、资源和可用资源数量
int safe = 0; // 用于标记系统状态是否安全
// 检查每个进程能否分配资源
for (int i = 0; i < 4; i++) {
if (!processes[i].finished) {
int j;
for (j = 0; j < 10; j++) {
if (processes[i].need[j] > available[j]) {
break; // 进程无法分配资源,继续下一个进程
}
}
if (j == 10) {
// 进程可以分配资源
for (int k = 0; k < 10; k++) {
available[k] += processes[i].allocated[k];
}
processes[i].finished = 1;
safe = 1;
}
}
}
if (safe) {
printf("System is safe.\n");
} else {
printf("System is not safe.\n");
}
return 0;
}
```
这里只是简要示范了如何使用C语言编写一个处理资源调度的系统,具体的实现还需要根据具体需求进行进一步的优化和完善。
阅读全文
相关推荐
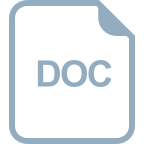
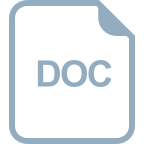
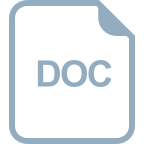

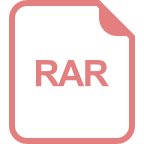
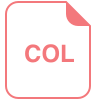
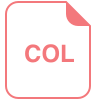
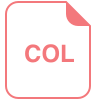
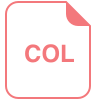
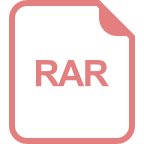
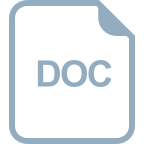
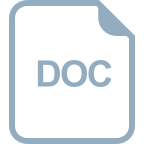
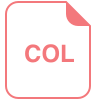
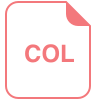
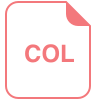
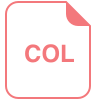
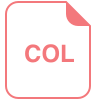
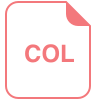
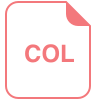