根据用户输入的商品号从数据库中查询该商品信息,或者查询所有商品信息。遵循MVC设计模式,其中视图有queryProduct.jsp、displayProduct.jsp、displayAllProduct.jsp和error.jsp几个页面,Product类实现模型,QueryProductServlet类实现控制器。数据库表为products。
时间: 2024-02-05 22:10:51 浏览: 99
好的,这是一个典型的MVC架构的Web应用程序。下面是实现该功能的代码示例:
1. 创建Product类实现模型
```java
public class Product {
private String id;
private String name;
private double price;
private int stock;
public Product(String id, String name, double price, int stock) {
this.id = id;
this.name = name;
this.price = price;
this.stock = stock;
}
// getter and setter methods
}
```
2. 创建QueryProductServlet类实现控制器
```java
public class QueryProductServlet extends HttpServlet {
private static final String QUERY_ALL = "all";
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String productId = request.getParameter("productId");
if (productId == null || productId.trim().isEmpty()) {
request.getRequestDispatcher("/error.jsp").forward(request, response);
} else if (productId.equals(QUERY_ALL)) {
List<Product> productList = queryAllProducts();
request.setAttribute("productList", productList);
request.getRequestDispatcher("/displayAllProduct.jsp").forward(request, response);
} else {
Product product = queryProduct(productId);
if (product == null) {
request.getRequestDispatcher("/error.jsp").forward(request, response);
} else {
request.setAttribute("product", product);
request.getRequestDispatcher("/displayProduct.jsp").forward(request, response);
}
}
}
private Product queryProduct(String productId) {
// connect to database and query product by id
// return null if the product is not found
}
private List<Product> queryAllProducts() {
// connect to database and query all products
}
}
```
3. 创建queryProduct.jsp页面
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Query Product</title>
</head>
<body>
<form action="/queryProduct" method="get">
<label for="productId">Product ID:</label>
<input type="text" name="productId" id="productId">
<button type="submit">Query</button>
</form>
</body>
</html>
```
4. 创建displayProduct.jsp页面
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Product Information</title>
</head>
<body>
<h1>Product Information</h1>
<table>
<tr>
<td>ID:</td>
<td>${product.id}</td>
</tr>
<tr>
<td>Name:</td>
<td>${product.name}</td>
</tr>
<tr>
<td>Price:</td>
<td>${product.price}</td>
</tr>
<tr>
<td>Stock:</td>
<td>${product.stock}</td>
</tr>
</table>
</body>
</html>
```
5. 创建displayAllProduct.jsp页面
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>All Products</title>
</head>
<body>
<h1>All Products</h1>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Price</th>
<th>Stock</th>
</tr>
<c:forEach var="product" items="${productList}">
<tr>
<td>${product.id}</td>
<td>${product.name}</td>
<td>${product.price}</td>
<td>${product.stock}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
6. 创建error.jsp页面
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Error</title>
</head>
<body>
<h1>Error</h1>
<p>Product not found!</p>
</body>
</html>
```
以上就是一个简单的查询商品信息的MVC架构Web应用程序的示例代码。需要注意的是,这里只是一个简单的示例,实际开发中还需要进行一些安全性和性能方面的优化。
阅读全文
相关推荐
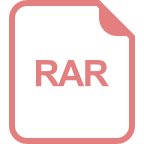



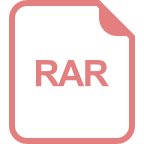
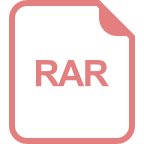



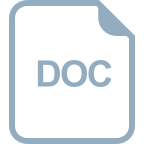

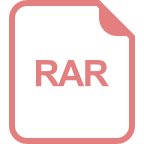



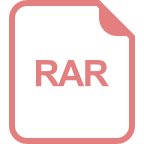