arcgis engine 实现在图上画一个点,让其随机移动的代码实现
时间: 2023-07-30 18:11:46 浏览: 104
以下是使用ArcGIS Engine实现在图上画一个点,并让其随机移动的代码实现:
```csharp
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using ESRI.ArcGIS.Controls;
using ESRI.ArcGIS.Carto;
using ESRI.ArcGIS.Geometry;
using ESRI.ArcGIS.Display;
namespace RandomPointMove
{
public partial class Form1 : Form
{
private IMapControl3 m_pMapControl;
private IGraphicsContainer m_pGraphicsContainer;
private IPoint m_pPoint;
private Random m_pRandom;
public Form1()
{
InitializeComponent();
axMapControl1.OnMouseDown += new IMapControlEvents2_Ax_OnMouseDownEventHandler(axMapControl1_OnMouseDown);
}
private void Form1_Load(object sender, EventArgs e)
{
m_pMapControl = axMapControl1.Object as IMapControl3;
m_pGraphicsContainer = m_pMapControl.Map as IGraphicsContainer;
m_pRandom = new Random(DateTime.Now.Millisecond);
}
private void axMapControl1_OnMouseDown(object sender, IMapControlEvents2_OnMouseDownEvent e)
{
if (e.button == 1)
{
IPoint pPoint = m_pMapControl.ToMapPoint(e.x, e.y);
AddPointGraphic(pPoint);
}
}
private void AddPointGraphic(IPoint pPoint)
{
m_pPoint = pPoint;
IMarkerSymbol pMarkerSymbol = new SimpleMarkerSymbolClass();
IRgbColor pColor = new RgbColorClass();
pColor.Red = 255;
pMarkerSymbol.Color = pColor;
pMarkerSymbol.Size = 10;
IElement pElement = new MarkerElementClass();
pElement.Geometry = m_pPoint;
pElement.Symbol = pMarkerSymbol;
m_pGraphicsContainer.AddElement(pElement, 0);
m_pMapControl.ActiveView.PartialRefresh(esriViewDrawPhase.esriViewGraphics, null, null);
timer1.Start();
}
private void timer1_Tick(object sender, EventArgs e)
{
double x = m_pPoint.X + m_pRandom.NextDouble() * 100 - 50;
double y = m_pPoint.Y + m_pRandom.NextDouble() * 100 - 50;
m_pPoint.PutCoords(x, y);
IElement pElement = m_pGraphicsContainer.Element[0];
pElement.Geometry = m_pPoint;
m_pGraphicsContainer.UpdateElement(pElement);
m_pMapControl.ActiveView.PartialRefresh(esriViewDrawPhase.esriViewGraphics, null, null);
}
}
}
```
这段代码中,我们首先在`IMapControlEvents2_OnMouseDownEvent`事件中添加了一个点的图形,然后在`timer1_Tick`事件中随机移动这个点的位置,通过`IGraphicsContainer`接口实现图形的添加、更新和刷新。
阅读全文
相关推荐
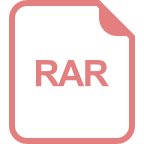
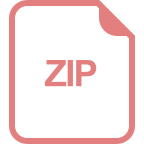
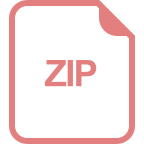
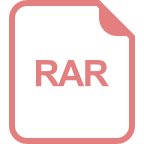
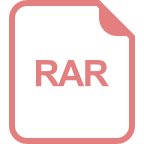
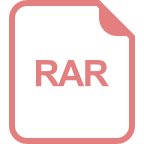
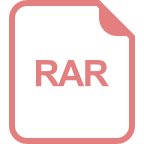
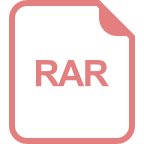
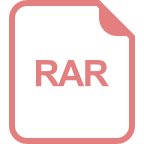
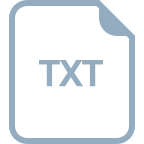
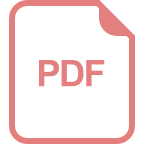
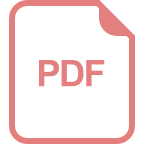
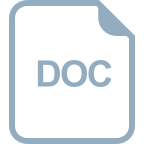
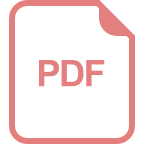